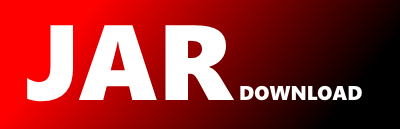
org.refcodes.filesystem.FileHandleImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-filesystem Show documentation
Show all versions of refcodes-filesystem Show documentation
Artifact for the refcodes virtual file system design.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.filesystem;
import java.util.Date;
/**
* Straight forward implementation of the {@link FileHandle},.
*/
public class FileHandleImpl implements FileHandle {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
protected String _path;
protected String _name;
protected long _size;
protected Date _createdDate;
protected Date _modifiedDate;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs a {@link FileHandle} object with the given properties.
*
* @param aPath The path to which the file handle points.
* @param aName The name to which the path points
* @param aSize The size of the file
* @param aCreatedDate The creation date of the file
* @param aModifiedDate The modified date of the file
*/
public FileHandleImpl( String aPath, String aName, long aSize, Date aCreatedDate, Date aModifiedDate ) {
_path = aPath;
_name = aName;
_size = aSize;
_createdDate = aCreatedDate;
_modifiedDate = aModifiedDate;
}
/**
* Constructs a {@link FileHandle} object with the given properties.
*
* @param aKey The path and the name (= the key) to which the file handle
* points.
*/
public FileHandleImpl( String aKey ) {
_path = FileSystemUtility.getPath( aKey );
_name = FileSystemUtility.getName( aKey );
}
/**
* Constructs a {@link FileHandle} object with the given properties.
*
* @param aPath The path to which the file handle points.
* @param aName The name to which the path points
*/
public FileHandleImpl( String aPath, String aName ) {
_path = aPath;
_name = aName;
}
/**
* Constructs a {@link FileHandle} object with the properties of the given
* {@link FileHandle}.
*
* @param aFileHandle The {@link FileHandle} from which to take the required
* properties.
*/
public FileHandleImpl( FileHandle aFileHandle ) {
_path = aFileHandle.getPath();
_name = aFileHandle.getName();
_size = aFileHandle.getFileSize();
_createdDate = aFileHandle.getCreatedDate();
_modifiedDate = aFileHandle.getModifiedDate();
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getPath() {
return _path;
}
/**
* {@inheritDoc}
*/
@Override
public String getName() {
return _name;
}
/**
* {@inheritDoc}
*/
@Override
public String toKey() {
return FileSystemUtility.toKey( _path, _name );
}
/**
* {@inheritDoc}
*/
@Override
public long getFileSize() {
return _size;
}
/**
* {@inheritDoc}
*/
@Override
public Date getCreatedDate() {
return _createdDate;
}
/**
* {@inheritDoc}
*/
@Override
public Date getModifiedDate() {
return _modifiedDate;
}
// /////////////////////////////////////////////////////////////////////////
// EQUALITY:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ( ( _name == null ) ? 0 : _name.hashCode() );
result = prime * result + ( ( _path == null ) ? 0 : _path.hashCode() );
return result;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) {
return true;
}
if ( obj == null ) {
return false;
}
// ---------------------------------------------------------------------
// We treat a mutable file handle the same as an immutable file handle
// in terms of equality. This makes live easier in collections though
// complicates things when modifying attributes while the mutable file
// handle is stored in a collection!
// ---------------------------------------------------------------------
if ( MutableFileHandleImpl.class != obj.getClass() && FileHandleImpl.class != obj.getClass() ) {
return false;
}
final FileHandle other = (FileHandle) obj;
if ( _name == null ) {
if ( other.getName() != null ) {
return false;
}
}
else if ( !_name.equals( other.getName() ) ) {
return false;
}
if ( _path == null ) {
if ( other.getPath() != null ) {
return false;
}
}
else if ( !_path.equals( other.getPath() ) ) {
return false;
}
return true;
}
// /////////////////////////////////////////////////////////////////////////
// MUTABLE:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public MutableFileHandle toMutableFileHandle() {
return new MutableFileHandleImpl( this );
}
/**
* The implementation of a {@link MutableFileHandle}.
* -------------------------------------------------------------------------
* CAUTION: fiddling with the path and the name attributes causes the
* {@link #hashCode()} and {@link #equals(Object)} methods to change
* behavior which can aCause problems ehttps://www.metacodes.proly in
* collections!
* -------------------------------------------------------------------------
* ATTENTION: In order to avoid the above mentioned problems with the
* {@link #equals(Object)} and {@link #hashCode()} methods, use
* {@link #toFileHandle()} before storing a {@link MutableFileHandle} in a
* collection.
*/
public class MutableFileHandleImpl extends FileHandleImpl implements MutableFileHandle {
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Instantiates a new mutable file handle impl.
*
* @param aPath the path
* @param aName the name
* @param aSize the size
* @param aCreatedDate the created date
* @param aModifiedDate the modified date
*/
public MutableFileHandleImpl( String aPath, String aName, long aSize, Date aCreatedDate, Date aModifiedDate ) {
super( aPath, aName, aSize, aCreatedDate, aModifiedDate );
}
/**
* Instantiates a new mutable file handle impl.
*
* @param aFileHandle the file handle
*/
public MutableFileHandleImpl( FileHandle aFileHandle ) {
super( aFileHandle );
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void setModifiedDate( Date aModifiedDate ) {
_modifiedDate = aModifiedDate;
}
/**
* {@inheritDoc}
*/
@Override
public void setFileSize( long aSize ) {
_size = aSize;
}
/**
* {@inheritDoc}
*/
@Override
public void setName( String aName ) {
_name = aName;
}
/**
* {@inheritDoc}
*/
@Override
public void setPath( String aPath ) {
_path = aPath;
}
/**
* {@inheritDoc}
*/
@Override
public void setCreatedDate( Date aCreatedDate ) {
_createdDate = aCreatedDate;
}
// /////////////////////////////////////////////////////////////////////////
// EQUALITY:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
return super.hashCode();
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
return super.equals( obj );
}
// /////////////////////////////////////////////////////////////////////////
// IMMUTABLE:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public FileHandle toFileHandle() {
return new FileHandleImpl( this );
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy