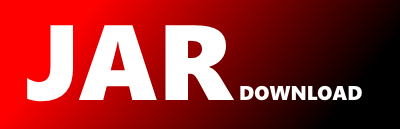
org.refcodes.filesystem.FileSystem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-filesystem Show documentation
Show all versions of refcodes-filesystem Show documentation
Artifact for the refcodes virtual file system design.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.filesystem;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
import org.refcodes.component.Component;
import org.refcodes.data.Delimiter;
/**
* A file system represents an abstraction for any "physical" storage medium
* where file (handles) can be stored and retrieved. The file (handles) are
* structured in a hierarchy similar to a file system's hierarchy with folders
* and files. The hierarchy may be completely virtual (the underlying system
* just knows of "keys" and directories are simulated by key naming
* conventions), so the underlying physical file system might be an FTP server
* or an in-memory {@link Map} (with virtual files and folders) or a real file
* system with a real directory and file hierarchy. The file system only
* contains file (handle)s, any construct such as a "directory" does not exist
* from the file system's point of view and therefore cannot be created or
* removed explicitly. Them are created or removed implicitly when file
* (handles) are created or removed. An implementation for, lets say, a file
* system, should take care to remove physical folders from the file system when
* removing a file (handle) (the corresponding file from the file system's
* folder) in case the folder from which the file (handle) (file) was removed
* ends up empty! In turn, when creating a file (handle), the corresponding
* directory structure must be created in case we have a file system with a
* "file system nature". File (handles) are never instantiated directly, them
* are retrieved from the file system as them can be implementation specific. A
* specific file (handle) instance therefore is always attached to a specific
* instance of a file system. Using file (handle)s interchangeably between tow
* different file system instances will (usually) fail. A file system and the
* file (handle)s are a self contained systems similar to the entities of an O/R
* mapper being attached to a specific persistence provider. Whenever an
* operation returns a file (handle), this file (handle) is to be considered the
* actual working copy and to be used in favor to a previous data file (handle)
* identifying the same data. The returned file (handle) will provide updated
* information. A file (handle) in the file system is identified by a unique
* key, which in turn consists of a path and a name. The path and the name are
* concatenated by a path separator, usually the slash "/". The path itself
* might be composed with path separators. Having this, a "file system nature"
* file system can map the file (handle)s to files in a directory hierarchy on
* the file system. Any path or key being provided is to be normalized by an
* implementation of the file system (using the {@link FileSystemUtility}) by
* removing any trailing path separators. Illegal paths will aCause an
* {@link IllegalPathException}, {@link IllegalNameException} or an
* {@link IllegalKeyException}, i.e. path which do not start with a path
* separator or have two or more subsequent path separators in then or any other
* illegal characters.
*/
public interface FileSystem extends Component {
/**
* The default path delimiter to be used by all implementations if this file
* system to ensure interoperability.
*/
char PATH_DELIMITER = Delimiter.PATH.getChar();
/**
* Tests whether the file system knows a file (handle) with the given key.
*
* @param aKey The key which to test if there is a file (handle).
*
* @return True in case there is a file (handle) with the given key exists.
*
* @throws IllegalKeyException in case the path was not a valid path.
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle).
*/
boolean hasFile( String aKey ) throws IllegalKeyException, NoListAccessException, UnknownFileSystemException, IOException;
/**
* Tests whether the file system knows a file (handle) with the given path
* and name. Caution, path and name are not just concatenated with a path
* separator in between to get the key: The name must not contain any path
* separator! In case of a file system implementation of the file system
* ("file system nature") the name must actually represent the filename of
* the file representing the file (handle).
*
* @param aPath The path part of the key for which to test if there is a
* file (handle).
* @param aName The name part of the key for which to test if there is a
* file (handle).
*
* @return True in case there is a file (handle) with the given path and
* name exists.
*
* @throws IllegalPathException in case the path was not a valid path.
* @throws IllegalNameException in case the name was not a valid path.
* @throws NoListAccessException in case listing the content in the file
* syste was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
*/
boolean hasFile( String aPath, String aName ) throws IllegalPathException, IllegalNameException, NoListAccessException, UnknownFileSystemException, IOException;
/**
* Returns true in case the given file (handle) exists.
*
* @param aFileHandle The file (handle) which's existence is to be tested.
*
* @return True in case the file (handle) exists, else false is returned.
*
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
boolean hasFile( FileHandle aFileHandle ) throws NoListAccessException, UnknownFileSystemException, IOException, IllegalFileException;
/**
* Creates a file (handle) with the given key.
*
* @param aKey The key for which to create the file (handle).
*
* @return The newly created file (handle).
*
* @throws FileAlreadyExistsException in case a file (handle) with the given
* key (path and name) already exists.
* @throws NoCreateAccessException in case create access to the file system
* was denied.
* @throws IllegalKeyException in case the key with name and path is not
* valid, i.e. the name contains a path separator.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
*/
FileHandle createFile( String aKey ) throws FileAlreadyExistsException, NoCreateAccessException, IllegalKeyException, UnknownFileSystemException, IOException, NoListAccessException;
/**
* Creates a file (handle) with the given path and name. Caution, path and
* name are not just concatenated with a path separator in between to get
* the key: The name must not contain any path separator! In case of a file
* system implementation of the file system ("file system alternative") the
* name will actually represent the filename of the file representing the
* file handle.
*
* @param aPath The path part of the key for which to create the file
* (handle).
* @param aName The name part of the key for which to create the file
* (handle).
*
* @return The newly created file (handle).
*
* @throws FileAlreadyExistsException in case a file (handle) with the given
* key (path and name) already exists.
* @throws NoCreateAccessException in case create access to the file system
* was denied.
* @throws IllegalNameException in case the name was not a valid path.
* @throws IllegalPathException in case the path was not a valid path.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
*/
FileHandle createFile( String aPath, String aName ) throws FileAlreadyExistsException, NoCreateAccessException, IllegalNameException, IllegalPathException, UnknownFileSystemException, IOException, NoListAccessException;
/**
* Gets a file (handle) with the given key from the file system.
*
* @param aKey The key for which to retrieve the file (handle).
*
* @return The retrieved file (handle).
*
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalKeyException in case the key with name and path is not
* valid, i.e. the name contains a path separator.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws UnknownKeyException in case the given key (path and name) does
* not exist in the file system.
*/
FileHandle getFileHandle( String aKey ) throws NoListAccessException, IllegalKeyException, UnknownFileSystemException, IOException, UnknownKeyException;
/**
* Gets a file (handle) with the given path and name from the file system.
* Caution, path and name are not just concatenated with a path separator in
* between to get the key: The name must not contain any path separator! In
* case of a file system implementation of the file system ("file system
* nature") the name will actually represent the filename of the file
* representing the file (handle).
*
* @param aPath The path part of the key for which to retrieve the file
* (handle).
* @param aName The name part of the key for which to retrieve the file
* (handle).
*
* @return The retrieved file (handle).
*
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalNameException in case the name was not a valid path.
* @throws IllegalPathException in case the path was not a valid path.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws UnknownKeyException in case the given key (path and name) does
* not exist in the file system.
*/
FileHandle getFileHandle( String aPath, String aName ) throws NoListAccessException, IllegalNameException, IllegalPathException, UnknownFileSystemException, IOException, UnknownKeyException;
/**
* The data contained in the given file (handle) is written to the provided
* output stream. This may be used to write the file (handle) to a file.
*
* @param aFromFileHandle The file (handle) which is to be written to the
* output stream.
* @param aOutputStream The stream to which to write.
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoReadAccessException in case read access to the file system was
* denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
void fromFile( FileHandle aFromFileHandle, OutputStream aOutputStream ) throws ConcurrentAccessException, UnknownFileException, NoReadAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Data provided by the given input stream is written to the file (handle)
* (or appended to the file (handle)'s data in case the file (handle) did
* already contain data). This may be used to store data from a file in a
* file (handle).
*
* @param aToFileHandle The file (handle) to which data is to be written.
* @param aInputStream The input stream from which to read the data which is
* to be written to the file (handle).
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoWriteAccessException in case write access to the file system
* was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
void toFile( FileHandle aToFileHandle, InputStream aInputStream ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* An input stream is being provided from which the data of the file
* (handle) may be read.
*
* @param aFromFileHandle The file (handle) for which to get the input
* stream.
*
* @return The input stream providing the data of the file (handle).
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoReadAccessException in case read access to the file system was
* denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
InputStream fromFile( FileHandle aFromFileHandle ) throws ConcurrentAccessException, UnknownFileException, NoReadAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Returns an output stream which may be used to write (or append, in case
* data did already exist for the file system) data to a file (handle).
*
* @param aToFileHandle The file (handle) to which to write data.
*
* @return The output stream to be used to write data to.
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoWriteAccessException in case write access to the file system
* was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
OutputStream toFile( FileHandle aToFileHandle ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, IllegalFileException;
/**
* The data contained in the given file (handle) is written to the provided
* file.
*
* @param aFromFileHandle The file (handle) which is to be written to the
* output stream.
* @param aToFile The file to which the data is to be written.
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoReadAccessException in case read access to the file system was
* denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
void fromFile( FileHandle aFromFileHandle, File aToFile ) throws ConcurrentAccessException, UnknownFileException, NoReadAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Data provided by the given input stream is written to the file (handle)
* (or appended to the file (handle)'s data in case the file (handle) did
* already contain data). This may be used to store data from a file in a
* file (handle).
*
* @param aToFileHandle The file (handle) to which data is to be written.
* @param aFromFile The file from which to read the data which is to be
* written to the file (handle).
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoWriteAccessException in case write access to the file system
* was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
void toFile( FileHandle aToFileHandle, File aFromFile ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Data provided by the given buffer is written to the file (handle) (or
* appended to the file (handle)'s data in case the file (handle) did
* already contain data). This may be used to store data from a string or
* other data structure.
*
* @param aToFileHandle The file (handle) to which data is to be written.
* @param aBuffer The buffer from which to read the data which is to be
* written to the file (handle).
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoWriteAccessException in case write access to the file system
* was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
void toFile( FileHandle aToFileHandle, byte[] aBuffer ) throws ConcurrentAccessException, UnknownFileException, NoWriteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Renames the file (handle), the name part of the key will be renamed to
* the new name. The name must not contain any path separator.
*
* @param aFileHandle The file (handle) to be renamed.
* @param aNewName The new name of the file (handle).
*
* @return The resulting file (handle) in case the operation was successful.
*
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws FileAlreadyExistsException in case a file (handle) with the given
* key (path and name) already exists.
* @throws NoCreateAccessException in case create access to the file system
* was denied.
* @throws NoDeleteAccessException in case create access to the file system
* was denied.
* @throws IllegalNameException in case the name was not a valid path.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
FileHandle renameFile( FileHandle aFileHandle, String aNewName ) throws UnknownFileException, ConcurrentAccessException, FileAlreadyExistsException, NoCreateAccessException, NoDeleteAccessException, IllegalNameException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Renames the file (handle), it will be accessible via the provided key.
*
* @param aFileHandle The file (handle) to be renamed.
* @param aNewKey The new key (path and name) of the file (handle).
*
* @return the file handle
*
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws FileAlreadyExistsException in case a file (handle) with the given
* key (path and name) already exists.
* @throws NoCreateAccessException in case create access to the file system
* was denied.
* @throws NoDeleteAccessException in case create access to the file system
* was denied.
* @throws IllegalKeyException in case the key with name and path is not
* valid, i.e. the name contains a path separator.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
FileHandle moveFile( FileHandle aFileHandle, String aNewKey ) throws UnknownFileException, ConcurrentAccessException, FileAlreadyExistsException, NoCreateAccessException, NoDeleteAccessException, IllegalKeyException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Deletes a file (handle) from the file system. In case a path is "empty"
* after deletion then the path will stop existing as well.
*
* @param aFileHandle The file (handle) to be deleted.
*
* @throws ConcurrentAccessException in case the file (handle) is
* concurrently read, modified or deleted.
* @throws UnknownFileException in case none such file (handle) was found in
* the file system.
* @throws NoDeleteAccessException in case create access to the file system
* was denied.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalFileException in case the file handle with name and path
* is not valid, i.e. the name contains a path separator.
*/
void deleteFile( FileHandle aFileHandle ) throws ConcurrentAccessException, UnknownFileException, NoDeleteAccessException, UnknownFileSystemException, IOException, NoListAccessException, IllegalFileException;
/**
* Determines whether there is any file (handle)s found for the given path.
* In case the recursive flag is set, then the specified path and all
* children of that path (separated by the path separator) are examined,
* else exactly the specified path is examined. Speaking of a set recursive
* flag, it is merely checked whether the given path is part of any key of
* the file (handle)s stored in the file system by taking the path
* separators into account. I.e. the given path must match a key from the
* beginning till the end of the path and the key must either continue from
* then on with a path separator as next character or must end there (be
* equal to the path). I.e. matches in between a key where the next position
* of the key does not contain a path separator are ignored. Example: A
* given path is "/a/bc/def", a given key is "a/bc/defg", the path does not
* match the key, although the path is contained in the key! A given path is
* "/a/bc/def", a given key is "a/bc/def/g", the path does match the key as
* the key continues the path with a path separator "/".
*
* @param aPath The path where to look whether there are file (handle)s or
* not.
* @param isRecursively When true all children of that path are examined as
* well.
*
* @return True in case file (handle)s were found.
*
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws IllegalPathException in case the path was not a valid path.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
*/
boolean hasFiles( String aPath, boolean isRecursively ) throws NoListAccessException, IllegalPathException, UnknownFileSystemException, IOException;
/**
* With the behavior of the {@link #hasFiles(String, boolean)} method, all
* file (handle)s found for the path are returned.
* Ehttps://www.metacodes.proly the recursive flag is described in the
* method {@link #hasFiles(String, boolean)}.
*
* @param aPath The path where to search for the file (handle)s.
* @param isRecursively the is recursively
*
* @return A list with the file (handle)s found or an empty list.
*
* @throws NoListAccessException in case listing the content in the file
* system was denied.
* @throws UnknownPathException in case the given path did not exist in the
* file system.
* @throws IllegalPathException in case the path was not a valid path.
* @throws UnknownFileSystemException in case the "underlying" (physical)
* file system is not known (may not exist any more), i.e. it may
* have been removed after the file system instance has been
* created.
* @throws IOException in case an I/O related problem occurred while
* accessing the file (handle)
*/
List getFileHandles( String aPath, boolean isRecursively ) throws NoListAccessException, UnknownPathException, IllegalPathException, UnknownFileSystemException, IOException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy