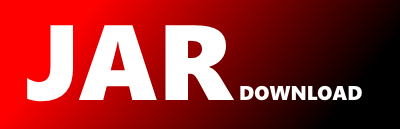
org.refcodes.filesystem.FileSystemUtility Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-filesystem Show documentation
Show all versions of refcodes-filesystem Show documentation
Artifact for the refcodes virtual file system design.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.filesystem;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.refcodes.exception.Trap;
/**
* The Class FileSystemUtility.
*/
public final class FileSystemUtility {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final Logger LOGGER = Logger.getLogger( FileSystemUtility.class.getName() );
private static final String RELATIVE_PATH_ELEMENT = "..";
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Private empty constructor to prevent instantiation as of being a utility
* with just static public methods.
*/
private FileSystemUtility() {}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Normalizes the given path. Some implementations have problems with a
* beginning separator character. This method removes the beginning
* separator, also replaces all "/" to the valid separator and returns the
* result.
*
* @param aPath The path to be normalized
*
* @return The normalized path
*/
public static String toNormalizedPath( String aPath ) {
aPath = aPath.replaceAll( "" + FileSystem.PATH_DELIMITER, "" + FileSystem.PATH_DELIMITER );
if ( aPath.startsWith( "" + FileSystem.PATH_DELIMITER ) ) {
aPath = aPath.substring( 1 );
}
return aPath;
}
/**
* Gets the name portion from the provided key (the key without the path
* portion).
*
* @param aKey The key from which to get the name portion
*
* @return The name portion
*/
public static String getName( String aKey ) {
final String name = aKey.substring( aKey.lastIndexOf( FileSystem.PATH_DELIMITER ) + 1 );
return name;
}
/**
* Gets the path portion from the provided key (the key without the name
* portion).
*
* @param aKey The key from which to get the path portion
*
* @return The path portion
*/
public static String getPath( String aKey ) {
final String path = aKey.subSequence( 0, aKey.lastIndexOf( FileSystem.PATH_DELIMITER ) ).toString();
return path;
}
/**
* Creates a key from the given path portion and name portion.
*
* @param aPath The path to use
* @param aName The name to use
*
* @return The key
*/
public static String toKey( String aPath, String aName ) {
return aPath + FileSystem.PATH_DELIMITER + aName;
}
/**
* Deletes the entries found for the given path.
*
* @param aFileSystem the file system
* @param aPath The path where to look whether there are file (handle)s or
* not.
* @param isRecursively When true all children of that path are examined as
* well.
*/
public static void deleteFiles( FileSystem aFileSystem, String aPath, boolean isRecursively ) {
try {
if ( aFileSystem.hasFiles( aPath, isRecursively ) ) {
final List theFiles = aFileSystem.getFileHandles( aPath, isRecursively );
for ( FileHandle eFile : theFiles ) {
LOGGER.log( Level.FINE, "Trying to delete file (handle) with key \"" + eFile.toKey() + "\"..." );
try {
aFileSystem.deleteFile( eFile );
}
catch ( Exception e ) {
LOGGER.log( Level.WARNING, "Unable to delete a file (handle) for path \"" + aPath + "\" though continuing with next entry (" + ( isRecursively ? "recursive" : "non-recursive" ) + ") as of: " + Trap.asMessage( e ), e );
}
LOGGER.log( Level.FINE, "Deleted file (handle) with key \"" + eFile.toKey() + "\"!" );
}
}
}
catch ( Exception e ) {
LOGGER.log( Level.WARNING, "Unable to delete file (handles) for path \"" + aPath + "\" and will abort (" + ( isRecursively ? "recursive" : "non-recursive" ) + ") as of: " + Trap.asMessage( e ), e );
}
}
// /////////////////////////////////////////////////////////////////////////
// HELPER
// /////////////////////////////////////////////////////////////////////////
/**
* Truncates any prefixed path separator as we assemble our new path with
* the according namespace in front.
*
* @param aPath The path to truncate.
*
* @return The truncated path, e.g. no prefixed path delimiters.
*/
public static String toTruncated( String aPath ) {
if ( aPath.startsWith( "" + FileSystem.PATH_DELIMITER ) ) {
aPath = aPath.substring( 1 );
}
return aPath;
}
/**
* Truncates any prefixed path separator as we assemble our new path with
* the according namespace in front. Test whether the given path may jail
* break from the name space. In case this "could" be the case, an
* {@link IllegalPathException} is thrown.
*
* @param aPath The path to be checked.
* @param aFileSystem The file system to use to get additional information.
*
* @return The truncated path.
*
* @throws IllegalPathException in case the path may jail break the name
* space.
*/
public static String toNormalizedPath( String aPath, FileSystem aFileSystem ) throws IllegalPathException {
if ( aPath.contains( RELATIVE_PATH_ELEMENT ) ) {
throw new IllegalPathException( "The given path containes relative path elements \"" + RELATIVE_PATH_ELEMENT + "\" which may aCause a jail break from the provided name space.", aPath );
}
return toTruncated( aPath );
}
/**
* Truncates any prefixed path separator as we assemble our new path with
* the according namespace in front. Test whether the given key may jail
* break from the name space. In case this "could" be the case, an
* {@link IllegalKeyException} is thrown.
*
* @param aKey The key to be checked.
* @param aFileSystem The file system to use to get additional information.
*
* @return The truncated key.
*
* @throws IllegalKeyException in case the key may jail break the name
* space.
*/
public static String toNormalizedKey( String aKey, FileSystem aFileSystem ) throws IllegalKeyException {
if ( aKey.contains( RELATIVE_PATH_ELEMENT ) ) {
throw new IllegalKeyException( "The given key containes relative path elements \"" + RELATIVE_PATH_ELEMENT + "\" which may aCause a jail break from the provided name space.", aKey );
}
return toTruncated( aKey );
}
/**
* Truncates any prefixed path separator as we assemble our new path with
* the according namespace in front. Test whether the given key may jail
* break from the name space. In case this "could" be the case, an
* {@link IllegalKeyException} is thrown.
*
* @param aName The key to be checked.
* @param aFileSystem The file system to use to get additional information.
*
* @return The truncated key.
*
* @throws IllegalNameException in case the name may jail break the name
* space.
*/
public static String toNormalizedName( String aName, FileSystem aFileSystem ) throws IllegalNameException {
if ( aName.contains( RELATIVE_PATH_ELEMENT ) ) {
throw new IllegalNameException( aName, "The given name containes relative path elements \"" + RELATIVE_PATH_ELEMENT + "\" which may aCause a jail break from the provided name space." );
}
return toTruncated( aName );
}
/**
* Test whether the given key may jail break from the name space. In case
* this "could" be the case, an {@link IllegalKeyException} is thrown.
*
* @param aFileHandle The file handle to be checked.
* @param aFileSystem The file system to use to get additional information.
*
* @return the file handle
*
* @throws IllegalFileException in case the key may jail break the name
* space.
*/
public static FileHandle toNormalizedFileHandle( FileHandle aFileHandle, FileSystem aFileSystem ) throws IllegalFileException {
if ( aFileHandle.getName().contains( RELATIVE_PATH_ELEMENT ) || aFileHandle.getPath().contains( RELATIVE_PATH_ELEMENT ) ) {
throw new IllegalFileException( aFileHandle, "The given file handle containes relative path elements \"" + RELATIVE_PATH_ELEMENT + "\" which may aCause a jail break from the provided name space." );
}
// ---------------------------------------------------------------------
// ATTENTION: In case the handle is modified, you must use the method
// aFileHandle.toMutableFileHandlke() and fiddle with the
// MutableFileHandle.
// ---------------------------------------------------------------------
return aFileHandle;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy