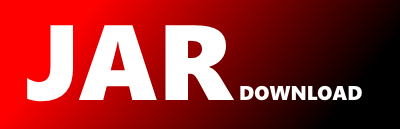
org.refcodes.graphical.Pixmap Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.graphical;
/**
* A pixmap represents the data of a two dimensional image. The pixels may be
* accessed by providing an x and a y position and, depending on the sub-types,
* a pixel my be represented by an {@link RgbPixel} (as of the {@link RgbPixmap}
* type).
*
* @param The type of the pixel being managed by the {@link Pixmap}.
*/
public interface Pixmap {
/**
* Returns the two dimensional pixels array representing the pixmap.
*
* @return The pixmap array.
*/
PX[][] getPixels();
/**
* Retrieves the width of the {@link Pixmap}.
*
* @return The width of the {@link Pixmap}
*/
int getPixmapWidth();
/**
* Retrieves the height of the {@link Pixmap}.
*
* @return The height of the {@link Pixmap}
*/
int getPixmapHeight();
/**
* Retrieves a pixel at a given position.
*
* @param aPosX The x position for the pixel to be retrieved.
* @param aPosY The y position for the pixel to be retrieved.
* @return The according pixel
*
* @throws IndexOutOfBoundsException in case the index is out of bounds.
*/
PX getPixelAt( int aPosX, int aPosY ) throws IndexOutOfBoundsException;
/**
* Provides a mutator for a pixmap property.
*/
public interface PixmapMutator {
/**
* Sets a pixel in the pixmap.
*
* @param aPixel The pixel to be placed at the given position.
* @param aPosX The x position of the pixel.
* @param aPosY The y position of the pixel.
*
* @throws IndexOutOfBoundsException in case the index is out of bounds.
*/
void setPixelAt( PX aPixel, int aPosX, int aPosY ) throws IndexOutOfBoundsException;
void setPixels( PX[][] aPixels );
}
/**
* Provides a builder method for a pixmap property returning the builder for
* applying multiple build operations.
*
* @param The type of the pixel being managed by the {@link Pixmap}.
*
* @param The builder to return in order to be able to apply multiple
* build operations.
*/
public interface PixmapBuilder> {
/**
* Sets the pixmap for the pixmap property.
*
* @param aHeight The pixmap to be stored by the pixmap property.
*
* @return The builder for applying multiple build operations.
*
* @throws IndexOutOfBoundsException in case the index is out of bounds.
*/
B withPixelAt( PX aPixel, int aPosX, int aPosY ) throws IndexOutOfBoundsException;
B withPixels( PX[][] aPixels );
}
/**
* Provides a pixmap property.
*
* @param The type of the pixel being managed by the {@link Pixmap}.
*/
public interface PixmapProperty extends Pixmap, PixmapMutator {}
/**
* Provides a pixmap property builder.
*
* @param The type of the pixel being managed by the {@link Pixmap}.
*/
public interface PixmapPropertyBuilder> extends PixmapProperty, PixmapBuilder> {
@SuppressWarnings("unchecked")
@Override
default B withPixelAt( PX aPixel, int aPosX, int aPosY ) throws IndexOutOfBoundsException {
return (B) this;
}
@SuppressWarnings("unchecked")
@Override
default B withPixels( PX[][] aPixels ) {
setPixels( aPixels );
return (B) this;
}
}
}