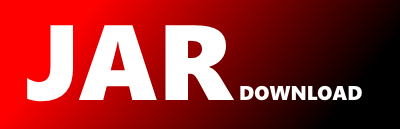
org.refcodes.jobbus.SimpleJobBus Maven / Gradle / Ivy
Show all versions of refcodes-jobbus Show documentation
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.jobbus;
import java.io.IOException;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import org.refcodes.command.NoExceptionAvailableRuntimeException;
import org.refcodes.command.NoResultAvailableRuntimeException;
import org.refcodes.command.NotYetExecutedRuntimeException;
import org.refcodes.command.Undoable;
import org.refcodes.component.HandleGenerator;
import org.refcodes.component.HandleTimeoutRuntimeException;
import org.refcodes.component.UnknownHandleRuntimeException;
import org.refcodes.component.UnsupportedHandleOperationRuntimeException;
/**
* The {@link SimpleJobBus} is a ready to use implementation of a composite
* {@link JobBus} wrapping a {@link JobBusDirectory} (actually a {@link JobBus}
* is sufficient) and delegating the method calls to the wrapped instances. The
* {@link JobBusDirectory} is considered to be the master from which multiple
* {@link JobBus} instances can be derived. In case none {@link JobBusDirectory}
* is considered to be required, use the empty constructor which creates its
* internal {@link JobBusDirectory}.
*
* In case you want to extend the {@link SimpleJobBus} with setting concrete
* parameters for the generic types, please also overwrite the hook method
*
* @param The context type to use, can by any component, service or POJO.
*/
public class SimpleJobBus implements JobBus {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private JobBus _jobBus;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates the {@link SimpleJobBus} by using the provided
* {@link JobBus} to which the method calls are delegated. Usually this
* constructor is used when you created a master {@link JobBusDirectory}
* from which client {@link JobBus} instances are to be derived. In case you
* do not require a master {@link JobBusDirectory}, please use the
* constructor {@link #SimpleJobBus(Object)}, which constructs the
* {@link JobBus} delegate with the the provided context.
*
* @param aJobBus The {@link JobBus} to delegate the method calls to.
*/
public SimpleJobBus( JobBus aJobBus ) {
_jobBus = aJobBus;
}
/**
* Instantiates the {@link SimpleJobBus} with the provided context and and a
* pre-defined {@link String} objects generating {@link HandleGenerator}. It
* is up to you which context (service,
* {@link org.refcodes.component.Component}, POJO) you want to provide to a
* job ({@link Undoable}) when being executed.
*
* @param aContext The context which is passed to the job ({@link Undoable})
* instances when being executed.
*/
public SimpleJobBus( CTX aContext ) {
_jobBus = createJobBus( aContext );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean hasHandle( String aHandle ) {
return _jobBus.hasHandle( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasProgress( String aHandle ) throws UnknownHandleRuntimeException {
return _jobBus.hasProgress( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasReset( String aHandle ) throws UnknownHandleRuntimeException {
return _jobBus.hasReset( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasFlush( String aHandle ) throws UnknownHandleRuntimeException {
return _jobBus.hasFlush( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public float getProgress( String aHandle ) throws UnsupportedHandleOperationRuntimeException, UnknownHandleRuntimeException {
return _jobBus.getProgress( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public Undoable lookupHandle( String aHandle ) throws UnknownHandleRuntimeException {
return _jobBus.lookupHandle( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public void reset( String aHandle ) throws UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException {
_jobBus.reset( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public void flush( String aHandle ) throws IOException, UnknownHandleRuntimeException, UnsupportedHandleOperationRuntimeException {
_jobBus.flush( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public Undoable removeHandle( String aHandle ) throws UnknownHandleRuntimeException {
return _jobBus.removeHandle( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public String execute( Undoable aJob ) {
return _jobBus.execute( aJob );
}
/**
* {@inheritDoc}
*/
@Override
public void execute( Undoable aJob, Consumer aResultConsumer ) {
_jobBus.execute( aJob, aResultConsumer );
}
/**
* {@inheritDoc}
*/
@Override
public void execute( Undoable aJob, BiConsumer aResultConsumer ) {
_jobBus.execute( aJob, aResultConsumer );
}
/**
* {@inheritDoc}
*/
@Override
public void waitForExecution( String aHandle ) throws UnknownHandleRuntimeException {
_jobBus.waitForExecution( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public void waitForExecution( String aHandle, long aTimeoutMillis ) throws UnknownHandleRuntimeException, HandleTimeoutRuntimeException {
_jobBus.waitForExecution( aHandle, aTimeoutMillis );
}
/**
* {@inheritDoc}
*/
@Override
public , RET> RET getResult( JOB aJob ) throws NoResultAvailableRuntimeException {
return _jobBus.getResult( aJob );
}
/**
* {@inheritDoc}
*/
@Override
public , RET> RET getResult( JOB aJob, long aTimeoutMillis ) throws NoResultAvailableRuntimeException, HandleTimeoutRuntimeException {
return _jobBus.getResult( aJob, aTimeoutMillis );
}
/**
* {@inheritDoc}
*/
@Override
public boolean isExecuted( String aHandle ) throws UnknownHandleRuntimeException {
return _jobBus.isExecuted( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasResult( String aHandle ) throws UnknownHandleRuntimeException, NotYetExecutedRuntimeException {
return _jobBus.hasResult( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public boolean hasException( String aHandle ) throws UnknownHandleRuntimeException, NotYetExecutedRuntimeException {
return _jobBus.hasException( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public RET getResult( String aHandle ) throws UnknownHandleRuntimeException, NotYetExecutedRuntimeException, NoResultAvailableRuntimeException {
return _jobBus.getResult( aHandle );
}
/**
* {@inheritDoc}
*/
@Override
public E getException( String aHandle ) throws UnknownHandleRuntimeException, NotYetExecutedRuntimeException, NoExceptionAvailableRuntimeException {
return _jobBus.getException( aHandle );
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Hook method pre-implemented useful when extending this class.
*
* @param aContext The context to be passed to the job ({@link Undoable})
* instances when being executed.
*
* @return The ready to use {@link JobBus}.
*/
protected JobBus createJobBus( CTX aContext ) {
return new SimpleJobBusDirectory( aContext );
}
}