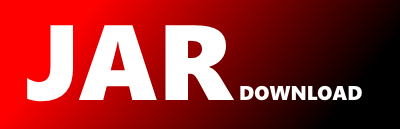
org.refcodes.matcher.PathMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-matcher Show documentation
Show all versions of refcodes-matcher Show documentation
Artifact for matcher frameworks and matching issues.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.matcher;
/**
* Path {@link Matcher}, matching its ANT like path pattern against the path
* provided to the {@link #isMatching(String)} and the like methods.
*
* The {@link PathMatcher} applies the following rules from the ANT path pattern
* to the path provided via {@link #isMatching(String)} method:
*
* A single asterisk ("*") matches zero or more characters within a path name. A
* double asterisk ("**") matches zero or more characters across directory
* levels. A question mark ("?") matches exactly one character within a path
* name.
*
* The single asterisk ("*"), the double asterisk ("**") and the question mark
* ("?") we refer to as wildcards: You get an array with the substitutes of the
* wildcards using the method {@link #toWildcardSubstitutes(String)} (or null,
* if your {@link String} does not match your path pattern).
*
* You may name a wildcard by prefixing it with "${somewildcardName}=". For
* example a named wildcard may look as follows: "${arg1}=*" or "${arg2}=**" or
* "${arg3}=?".
*
* The regular expression pattern construction is inspired by:
* "http://stackoverflow.com/questions/33171025/regex-matching-an-ant-path"
*/
public interface PathMatcher extends Matcher {
/**
* The this method applies the following rules from the configured ANT path
* pattern to the path provided via {@link #isMatching(String)} method:
*
* A single asterisk ("*") matches zero or more characters within a path
* name. A double asterisk ("**") matches zero or more characters across
* directory levels. A question mark ("?") matches exactly one character
* within a path name
*/
@Override
boolean isMatching( String aPath );
/**
* Returns the path pattern being used by the {@link PathMatcher}.
*
* @return The path pattern being used.
*/
String getPathPattern();
/**
* Returns all available wildcard substitutes as well as the named wildcard
* substitutes.
*
* @param aPath The path for which to retrieve the wildcard substitutes.
*
* @return The {@link WildcardSubstitutes} of the wildcards being
* substituted.
*/
WildcardSubstitutes toWildcardSubstitutes( String aPath );
/**
* Returns an array of the wildcard substitutes for the wildcards in your
* path pattern (see {@link PathMatcher#getPathPattern()}) compared to the
* actual path (as of {@link PathMatcher#toWildcardSubstitutes(String)}).
* The order of the wildcard substitutes aligns to the order of the
* wildcards (from left to right) defined in your path pattern.
*
* @param aPath The path for which to retrieve the wildcard substitutes.
*
* @return The text substituting the wildcards in the order of the wildcards
* being substituted or null if there are none such substitutes.
*/
String[] toWildcardReplacements( String aPath );
/**
* Returns the wildcard substitute for the wildcards in your path pattern
* (see {@link PathMatcher#getPathPattern()}) compared to the actual path
* (as of {@link PathMatcher#toWildcardSubstitutes(String)}). The text of
* the wildcard substitute aligns to the index of the wildcard (from left to
* right) as defined in your path pattern.
*
* @param aPath The path for which to retrieve the wildcard substitutes.
*
* @param aIndex The index of the wildcard in question for which to retrieve
* the substitute.
*
* @return The text substituting the wildcard at the given path pattern's
* wildcard index or null if there is none such substitute.
*/
String toWildcardReplacementAt( String aPath, int aIndex );
/**
* Returns the wildcard substitutes for the wildcards in your path pattern
* (see {@link PathMatcher#getPathPattern()}) compared to the actual path
* (as of {@link PathMatcher#toWildcardSubstitutes(String)}). The text of
* the wildcard substitutes aligns to the indexes of the wildcard (from left
* to right) as defined in your path pattern.
*
* @param aPath The path for which to retrieve the wildcard substitutes.
*
* @param aIndexes The indexes of the wildcards in question for which to
* retrieve the substitutes.
*
* @return The text substituting the wildcards at the given path pattern's
* wildcard indexes or null if there is none such substitute.
*/
String[] toWildcardReplacementsAt( String aPath, int... aIndexes );
/**
* Returns the wildcard substitute for the wildcards in your path pattern
* (see {@link PathMatcher#getPathPattern()}) compared to the actual path
* (as of {@link PathMatcher#toWildcardSubstitutes(String)}). The text of
* the wildcard substitute aligns to the name of the wildcard (as defined in
* your path pattern).
*
* @param aPath The path for which to retrieve the wildcard substitutes.
*
* @param aWildcardName The name of the wildcard in question for which to
* retrieve the substitute.
*
* @return The text substituting the wildcard with the given path pattern's
* wildcard name or null if there is none such substitute.
*/
String toWildcardReplacement( String aPath, String aWildcardName );
/**
* Returns the wildcard substitutes for the wildcards in your path pattern
* (see {@link PathMatcher#getPathPattern()}) compared to the actual path
* (as of {@link PathMatcher#toWildcardSubstitutes(String)}). The text of
* the wildcard substitutes aligns to the order of the provided wildcard
* names (as defined in your path pattern).
*
* @param aPath The path for which to retrieve the wildcard substitutes.
*
* @param aWildcardNames The names of the wildcards in question for which to
* retrieve the substitutes in the order of the provided names.
*
* @return The text substituting the wildcard with the given path pattern's
* wildcard names or null if there are none such substitute.
*/
String[] toWildcardReplacements( String aPath, String... aWildcardNames );
/**
* Retrieves the list of wildcard names identifying the wildcards as
* specified by the path pattern.
*
* @return The wild card names or null of no wild card names have been
* defined.
*/
String[] getWildcardNames();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy