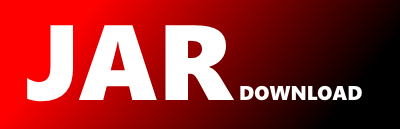
org.refcodes.mixin.BoxGrid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-mixin Show documentation
Show all versions of refcodes-mixin Show documentation
Artifact for mixins of general interest.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.mixin;
/**
* The {@link BoxGrid} interface defines all elements required to draw boxes
* into a grid. For example the grid might be a text mode screen and the
* elements might be the according characters. Taking the example of the text
* mode screen and the character elements, them elements might be as follows:
*
* ┌──┬──┐ ╔══╦══╗ ╒══╤══╕ ╓──╥──╖
* │ │ │ ║ ║ ║ │ │ │ ║ ║ ║
* ├──┼──┤ ╠══╬══╣ ╞══╪══╡ ╟──╫──╢
* │ │ │ ║ ║ ║ │ │ │ ║ ║ ║
* └──┴──┘ ╚══╩══╝ ╘══╧══╛ ╙──╨──╜
*
*
* @param The type of the grid elements for drawing a box grid, may be a
* char or a sprite or a byte array.
*/
public interface BoxGrid {
/**
* Returns the box's left edge character, for example "├".
*
* @return The left edge character.
*/
T getLeftEdge();
/**
* Returns the box's top left edge character, for example "┌".
*
* @return The top left edge character.
*/
T getTopLeftEdge();
/**
* Returns the box's divider edge character, for example "┼".
*
* @return The divider edge character.
*/
T getDividerEdge();
/**
* Returns the box's top divider edge character, for example "┬".
*
* @return The top divider edge character.
*/
T getTopDividerEdge();
/**
* Returns the box's top right edge character, for example "┐".
*
* @return The top right edge character.
*/
T getTopRightEdge();
/**
* Returns the box's right edge character, for example "┤".
*
* @return The top left edge character.
*/
T getRightEdge();
/**
* Returns the box's left line character, for example "│".
*
* @return The left line character.
*/
T getLeftLine();
/**
* Returns the box's divider line character, for example "│".
*
* @return The divider line character.
*/
T getDividerLine();
/**
* Returns the box row's right line character, for example "│".
*
* @return The right line character.
*/
T getRightLine();
/**
* Returns the box's top line character, for example "─" .
*
* @return The top line character.
*/
T getTopLine();
/**
* Returns the box's bottom right edge character, for example "┘".
*
* @return The bottom left edge character.
*/
T getBottomRightEdge();
/**
* Returns the box's bottom divider edge character, for example "┴".
*
* @return The bottom divider edge character.
*/
T getBottomDividerEdge();
/**
* Returns the box's bottom left edge character, for example "└".
*
* @return The bottom left edge character.
*/
T getBottomLeftEdge();
/**
* Returns the box's bottom line character, for example "─".
*
* @return The bottom line character.
*/
T getBottomLine();
/**
* Returns the box's inner line character, for example "─".
*
* @return The inner line character.
*/
T getInnerLine();
/**
* Provides a mutator for a box grid property.
*
* @param The type of the grid elements for drawing a box grid, may be a
* char or a sprite or a byte array.
*/
public interface BoxGridMutator {
/**
* Sets the box's left edge character, for example "├".
*
* @param aGridElement The left edge character.
*/
void setLeftEdge( T aGridElement );
/**
* Sets the box's top left edge character, for example "┌".
*
* @param aGridElement The top left edge character.
*/
void setTopLeftEdge( T aGridElement );
/**
* Sets the box's divider edge character, for example "┼".
*
* @param aGridElement The divider edge character.
*/
void setDividerEdge( T aGridElement );
/**
* Sets the box's top divider edge character, for example "┬".
*
* @param aGridElement The top divider edge character.
*/
void setTopDividerEdge( T aGridElement );
/**
* Sets the box's top right edge character, for example "┐".
*
* @param aGridElement The top right edge character.
*/
void setTopRightEdge( T aGridElement );
/**
* Sets the box's right edge character, for example "┤".
*
* @param aGridElement The top left edge character.
*/
void setRightEdge( T aGridElement );
/**
* Sets the box's left line character, for example "│".
*
* @param aGridElement The left line character.
*/
void setLeftLine( T aGridElement );
/**
* Sets the box's divider line character, for example "│".
*
* @param aGridElement The divider line character.
*/
void setDividerLine( T aGridElement );
/**
* Sets the box row's right line character, for example "│".
*
* @param aGridElement The right line character.
*/
void setRightLine( T aGridElement );
/**
* Sets the box's top line character, for example "─" .
*
* @param aGridElement The top line character.
*/
void setTopLine( T aGridElement );
/**
* Sets the box's bottom right edge character, for example "┘".
*
* @param aGridElement The bottom left edge character.
*/
void setBottomRightEdge( T aGridElement );
/**
* Sets the box's bottom divider edge character, "┴".
*
* @param aGridElement The bottom divider edge character.
*/
void setBottomDividerEdge( T aGridElement );
/**
* Sets the box's bottom left edge character, for example "└".
*
* @param aGridElement The bottom left edge character.
*/
void setBottomLeftEdge( T aGridElement );
/**
* Sets the box's bottom line character, for example "─".
*
* @param aGridElement The bottom line character.
*/
void setBottomLine( T aGridElement );
/**
* Sets the box's inner line character, for example "─".
*
* @param aGridElement The inner line character.
*/
void setInnerLine( T aGridElement );
}
/**
* Provides a builder for a box grid property returning the builder for
* applying multiple build operations.
*
* @param The type of the grid elements for drawing a box grid, may be a
* char or a sprite or a byte array.
* @param The builder to return in order to be able to apply multiple
* build operations.
*/
public interface BoxGridBuilder {
/**
* Sets the box's left edge character, for example "├".
*
* @param aGridElement The left edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withLeftEdge( T aGridElement );
/**
* Sets the box's top left edge character, for example "┌".
*
* @param aGridElement The top left edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withTopLeftEdge( T aGridElement );
/**
* Sets the box's divider edge character, for example "┼".
*
* @param aGridElement The divider edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withDividerEdge( T aGridElement );
/**
* Sets the box's top divider edge character, for example "┬".
*
* @param aGridElement The top divider edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withTopDividerEdge( T aGridElement );
/**
* Sets the box's top right edge character, for example "┐".
*
* @param aGridElement The top right edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withTopRightEdge( T aGridElement );
/**
* Sets the box's right edge character, for example "┤".
*
* @param aGridElement The top left edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withRightEdge( T aGridElement );
/**
* Sets the box's left line character, for example "│".
*
* @param aGridElement The left line character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withLeftLine( T aGridElement );
/**
* Sets the box's divider line character, for example "│".
*
* @param aGridElement The divider line character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withDividerLine( T aGridElement );
/**
* Sets the box row's right line character, for example "│".
*
* @param aGridElement The right line character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withRightLine( T aGridElement );
/**
* Sets the box's top line character, for example "─" .
*
* @param aGridElement The top line character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withTopLine( T aGridElement );
/**
* Sets the box's bottom right edge character, for example "┘".
*
* @param aGridElement The bottom left edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withBottomRightEdge( T aGridElement );
/**
* Sets the box's bottom divider edge character, "┴".
*
* @param aGridElement The bottom divider edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withBottomDividerEdge( T aGridElement );
/**
* Sets the box's bottom left edge character, for example "└".
*
* @param aGridElement The bottom left edge character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withBottomLeftEdge( T aGridElement );
/**
* Sets the box's bottom line character, for example "─".
*
* @param aGridElement The bottom line character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withBottomLine( T aGridElement );
/**
* Sets the box's inner line character, for example "─".
*
* @param aGridElement The inner line character.
*
* @return The implementing instance as of the builder pattern for
* chaining chain method calls.
*/
B withInnerLine( T aGridElement );
}
/**
* Provides a channel property.
*
* @param the generic type
*/
public interface BoxGridProperty extends BoxGrid, BoxGridMutator {
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setBottomDividerEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setBottomDividerEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letBottomDividerEdge( T aGridElement ) {
setBottomDividerEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setBottomLeftEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setBottomLeftEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letBottomLeftEdge( T aGridElement ) {
setBottomLeftEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setBottomLine(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setBottomLine(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letBottomLine( T aGridElement ) {
setBottomLine( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setBottomRightEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setBottomRightEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letBottomRightEdge( T aGridElement ) {
setBottomRightEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setDividerEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setDividerEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letDividerEdge( T aGridElement ) {
setDividerEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setDividerLine(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setDividerLine(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letDividerLine( T aGridElement ) {
setDividerLine( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setInnerLine(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setInnerLine(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letInnerLine( T aGridElement ) {
setInnerLine( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setLeftEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setLeftEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letLeftEdge( T aGridElement ) {
setLeftEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setLeftLine(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setLeftLine(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letLeftLine( T aGridElement ) {
setLeftLine( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setRightEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setRightEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letRightEdge( T aGridElement ) {
setRightEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setRightLine(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setRightLine(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letRightLine( T aGridElement ) {
setRightLine( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setTopDividerEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setTopDividerEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letTopDividerEdge( T aGridElement ) {
setTopDividerEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setTopLeftEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setTopLeftEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letTopLeftEdge( T aGridElement ) {
setTopLeftEdge( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setTopLine(Object)} and returns the very same value (getter).
*
* @param aGridElement The value to set (via
* {@link #setTopLine(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letTopLine( T aGridElement ) {
setTopLine( aGridElement );
return aGridElement;
}
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given value (setter) as of
* {@link #setTopRightEdge(Object)} and returns the very same value
* (getter).
*
* @param aGridElement The value to set (via
* {@link #setTopRightEdge(Object)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default T letTopRightEdge( T aGridElement ) {
setTopRightEdge( aGridElement );
return aGridElement;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy