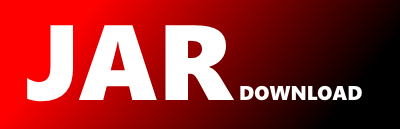
org.refcodes.net.HttpFields Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-net Show documentation
Show all versions of refcodes-net Show documentation
This artifact provides networking (TCP/IP) related definitions and types being
used by REFCODES.ORG networking related functionality and artifacts.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.net;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* Various key / value "collections" we run across when we develop HTTP based
* applications may have more then one value for one key. Such "collections" in
* this package are the {@link HeaderFields} as well as the {@link FormFields}.
*
* This interface represents this kind of data structure providing some common
* convenience methods.
*/
public interface HttpFields> extends Map> {
/**
* Returns the first header field value in the list of values associated
* with the given header field.
*
* @param aField The header field (key) of which's values the first value is
* to be retrieved.
*
* @return The first value in the list of values associated to the given
* header field (key).
*/
default String getFirst( String aField ) {
List theValues = get( aField );
if ( theValues != null ) {
synchronized ( this ) {
if ( theValues.size() > 0 ) { return theValues.get( 0 ); }
}
}
return null;
}
/**
* Sets a single value for the header field (key). Any values previously
* associated to the given header field (key) are lost.
*
* @param aField The header field for which to set a single value.
*
* @param aValue The single value to be set for the header field (key).
*/
default void set( String aField, String aValue ) {
if ( aValue == null ) {
remove( aField );
return;
}
List theValues = get( aField );
if ( theValues == null ) {
synchronized ( this ) {
theValues = get( aField );
if ( theValues == null ) {
theValues = new ArrayList<>();
put( aField, theValues );
}
else {
theValues.clear();
}
}
}
theValues.add( aValue );
}
/**
* Adds a value to the list of values associated with the given header field
* (key),
*
* @param aField The header field (key) of which's list of values a value is
* to be added.
*
* @param aValue The value to be added to the list of values associated to
* the given header field (key).
*/
default void addTo( String aField, String aValue ) {
if ( aValue == null ) { throw new IllegalArgumentException( "You must not add a <" + aValue + "> value to a header field <" + aField + ">." ); }
List theValues = get( aField );
if ( theValues == null ) {
synchronized ( this ) {
theValues = get( aField );
if ( theValues == null ) {
theValues = new ArrayList<>();
put( aField, theValues );
}
}
}
theValues.add( aValue );
}
/**
* Builder method for the {@link #addTo(String, String)} method.
*
* @param aField The header field (key) of which's list of values a value is
* to be added.
*
* @param aValue The value to be added to the list of values associated to
* the given header field (key).
*
* @return This {@link HeaderFields} instance to continue building up the
* header fields.
*/
@SuppressWarnings("unchecked")
default T withAddTo( String aField, String aValue ) {
addTo( aField, aValue );
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy