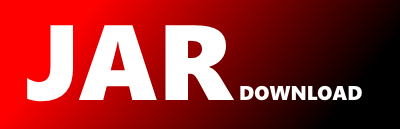
org.refcodes.net.HttpStatusCode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-net Show documentation
Show all versions of refcodes-net Show documentation
This artifact provides networking (TCP/IP) related definitions and types being
used by REFCODES.ORG networking related functionality and artifacts.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.net;
import org.refcodes.mixin.ValueAccessor;
/**
* The org.apache.commons.httpclient.HttpStatus constants as enumeration.
*/
public enum HttpStatusCode implements ValueAccessor { // StatusCodeAccessor {
// /////////////////////////////////////////////////////////////////////////
// 1XX INFORMATIONAL:
// /////////////////////////////////////////////////////////////////////////
/**
* 100 Continue (HTTP/1.1 - RFC 2616)
*/
CONTINUE(100),
/**
* 101 Switching Protocols (HTTP/1.1 - RFC 2616)
*/
SWITCHING_PROTOCOLS(101),
/**
* 102 Processing (WebDAV - RFC 2518)
*/
PROCESSING(102),
// /////////////////////////////////////////////////////////////////////////
// 2XX SUCCESS:
// /////////////////////////////////////////////////////////////////////////
/**
* 200 OK (HTTP/1.0 - RFC 1945)
*/
OK(200),
/**
* 201 Created (HTTP/1.0 - RFC 1945)
*/
CREATED(201),
/**
* 202 Accepted (HTTP/1.0 - RFC 1945)
*/
ACCEPTED(202),
/**
* 203 Non Authoritative Information (HTTP/1.1 - RFC 2616)
*/
NON_AUTHORITATIVE_INFORMATION(203),
/**
* 204 No Content (HTTP/1.0 - RFC 1945)
*/
NO_CONTENT(204),
/**
* 205 Reset Content (HTTP/1.1 - RFC 2616)
*/
RESET_CONTENT(205),
/**
* 206 Partial Content (HTTP/1.1 - RFC 2616)
*/
PARTIAL_CONTENT(206),
/**
* 207 Multi-Status (WebDAV - RFC 2518) or 207 Partial Update OK (HTTP/1.1 -
* draft-ietf-http-v11-spec-rev-01?)
*/
MULTI_STATUS(207),
// /////////////////////////////////////////////////////////////////////////
// 3XX REDIRECTION:
// /////////////////////////////////////////////////////////////////////////
/**
* 300 Mutliple Choices (HTTP/1.1 - RFC 2616)
*/
MULTIPLE_CHOICES(300),
/**
* 301 Moved Permanently (HTTP/1.0 - RFC 1945)
*/
MOVED_PERMANENTLY(301),
/**
* 302 Moved Temporarily (Sometimes Found) (HTTP/1.0 - RFC 1945)
*/
MOVED_TEMPORARILY(302),
/**
* 303 See Other (HTTP/1.1 - RFC 2616)
*/
SEE_OTHER(303),
/**
* 304 Not Modified (HTTP/1.0 - RFC 1945)
*/
NOT_MODIFIED(304),
/**
* 305 Use Proxy (HTTP/1.1 - RFC 2616)
*/
USE_PROXY(305),
/**
* 307 Temporary Redirect (HTTP/1.1 - RFC 2616)
*/
TEMPORARY_REDIRECT(307),
// /////////////////////////////////////////////////////////////////////////
// 4XX CLIENT ERROR:
// /////////////////////////////////////////////////////////////////////////
/**
* 400 Bad Request (HTTP/1.1 - RFC 2616)
*/
BAD_REQUEST(400),
/**
* 401 Unauthorized (HTTP/1.0 - RFC 1945)
*/
UNAUTHORIZED(401),
/**
* 402 Payment Required (HTTP/1.1 - RFC 2616)
*/
PAYMENT_REQUIRED(402),
/**
* 403 Forbidden (HTTP/1.0 - RFC 1945)
*/
FORBIDDEN(403),
/**
* 404 Not Found (HTTP/1.0 - RFC 1945)
*/
NOT_FOUND(404),
/**
* 405 Method Not Allowed (HTTP/1.1 - RFC 2616)
*/
METHOD_NOT_ALLOWED(405),
/**
* 406 Not Acceptable (HTTP/1.1 - RFC 2616)
*/
NOT_ACCEPTABLE(406),
/**
* 407 Proxy Authentication Required (HTTP/1.1 - RFC 2616)
*/
PROXY_AUTHENTICATION_REQUIRED(407),
/**
* 408 Request Timeout (HTTP/1.1 - RFC 2616)
*/
REQUEST_TIMEOUT(408),
/**
* 409 Conflict (HTTP/1.1 - RFC 2616)
*/
CONFLICT(409),
/**
* 410 Gone (HTTP/1.1 - RFC 2616)
*/
GONE(410),
/**
* 411 Length Required (HTTP/1.1 - RFC 2616)
*/
LENGTH_REQUIRED(411),
/**
* 412 Precondition Failed (HTTP/1.1 - RFC 2616)
*/
PRECONDITION_FAILED(412),
/**
* 413 Request Entity Too Large (HTTP/1.1 - RFC 2616)
*/
REQUEST_TOO_LONG(413),
/**
* 414 Request-URI Too Long (HTTP/1.1 - RFC 2616)
*/
REQUEST_URI_TOO_LONG(414),
/**
* 415 Unsupported Media Type (HTTP/1.1 - RFC 2616)
*/
UNSUPPORTED_MEDIA_TYPE(415),
/**
* 416 Requested Range Not Satisfiable (HTTP/1.1 - RFC 2616)
*/
REQUESTED_RANGE_NOT_SATISFIABLE(416),
/**
* 417 Expectation Failed (HTTP/1.1 - RFC 2616)
*/
EXPECTATION_FAILED(417),
/**
* 418 Unprocessable Entity (WebDAV drafts?) or 418 Reauthentication
* Required (HTTP/1.1 drafts?)
*/
REAUTHENTICATION_REQUIRED(418),
/**
* 419 Insufficient Space on Resource (WebDAV -
* draft-ietf-webdav-protocol-05?) or 419 Proxy Reauthentication Required
* (HTTP/1.1 drafts?)
*/
INSUFFICIENT_SPACE_ON_RESOURCE(419),
/**
* 420 Method Failure (WebDAV - draft-ietf-webdav-protocol-05?)
*/
METHOD_FAILURE(420),
/**
* 422 Unprocessable Entity (WebDAV - RFC 2518)
*/
UNPROCESSABLE_ENTITY(422),
/**
* 423 Locked (WebDAV - RFC 2518)
*/
LOCKED(423),
/**
* 424 Failed Dependency (WebDAV - RFC 2518)
*/
FAILED_DEPENDENCY(424),
// /////////////////////////////////////////////////////////////////////////
// 5XX SERVER ERROR:
// /////////////////////////////////////////////////////////////////////////
/**
* 500 Server Error (HTTP/1.0 - RFC 1945)
*/
INTERNAL_SERVER_ERROR(500),
/**
* 501 Not Implemented (HTTP/1.0 - RFC 1945)
*/
NOT_IMPLEMENTED(501),
/**
* 502 Bad Gateway (HTTP/1.0 - RFC 1945)
*/
BAD_GATEWAY(502),
/**
* 503 Service Unavailable (HTTP/1.0 - RFC 1945)
*/
SERVICE_UNAVAILABLE(503),
/**
* 504 Gateway Timeout (HTTP/1.1 - RFC 2616)
*/
GATEWAY_TIMEOUT(504),
/**
* 505 HTTP Version Not Supported (HTTP/1.1 - RFC 2616)
*/
HTTP_VERSION_NOT_SUPPORTED(505),
/**
* 507 Insufficient Storage (WebDAV - RFC 2518)
*/
INSUFFICIENT_STORAGE(507);
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private Integer _statusCode;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
private HttpStatusCode( Integer aStatusCode ) {
_statusCode = aStatusCode;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
@Override
public Integer getValue() {
return _statusCode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy