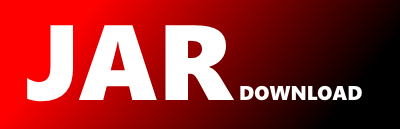
org.refcodes.properties.ext.cli.ArgsParserProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties-ext-cli Show documentation
Show all versions of refcodes-properties-ext-cli Show documentation
Artifact extending the refcodes-properties artifact with CLI functionality.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties.ext.cli;
import java.io.PrintStream;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import java.util.regex.Pattern;
import org.refcodes.cli.ArgsFilter;
import org.refcodes.cli.ArgsParser;
import org.refcodes.cli.ArgsSyntaxException;
import org.refcodes.cli.CliContext;
import org.refcodes.cli.Condition;
import org.refcodes.cli.Example;
import org.refcodes.cli.Operand;
import org.refcodes.cli.Option;
import org.refcodes.cli.ParseArgs;
import org.refcodes.cli.SyntaxMetrics;
import org.refcodes.cli.SyntaxNotation;
import org.refcodes.cli.Term;
import org.refcodes.data.ArgsPrefix;
import org.refcodes.data.AsciiColorPalette;
import org.refcodes.properties.Properties;
import org.refcodes.properties.PropertiesBuilderImpl;
import org.refcodes.properties.PropertiesImpl;
import org.refcodes.runtime.Arguments;
import org.refcodes.textual.Font;
import org.refcodes.textual.TextBoxGrid;
import org.refcodes.textual.TextBoxStyle;
/**
* The {@link ArgsParserProperties} class implements the
* {@link ParseArgsProperties} type to combine the {@link ParseArgs} type with
* the {@link Properties} type in a dedicated class.
*/
public class ArgsParserProperties extends ArgsParser implements ParseArgsProperties {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private Properties _properties = new PropertiesImpl();
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs the {@link ParseArgsProperties} instance with no syntax
* notation (no root {@link Condition}). As no syntax notation is required
* by the constructor (no root {@link Condition}), no syntax validation is
* done by {@link #evalArgs(String[])} and the like methods. When no syntax
* validation is fine for you, you can directly go for the constructor
* {@link #ArgsParserProperties(String[])}.
*/
public ArgsParserProperties() {}
/**
* Constructs the {@link ParseArgsProperties} instance with the given root
* {@link Term} and the default {@link SyntaxNotation#LOGICAL}.
*
* @param aArgsSyntax The args syntax root {@link Term} node being the node
* from which parsing the command line arguments starts.
*/
public ArgsParserProperties( Term aArgsSyntax ) {
super( aArgsSyntax );
}
/**
* Constructs the {@link ParseArgsProperties} instance with the given
* arguments and the default {@link SyntaxNotation#LOGICAL}. As no syntax
* notation is required by the constructor (no root {@link Condition}), no
* syntax validation is done. Therefore the properties are heuristically
* determined from the provided command line arguments.
*
* @param aArgs The command line arguments to be evaluated.
*/
public ArgsParserProperties( String[] aArgs ) {
_properties = new PropertiesBuilderImpl( Arguments.toProperties( aArgs, ArgsPrefix.toPrefixes(), getDelimiter() ) );
}
/**
* Constructs the {@link ParseArgsProperties} instance with the given root
* {@link Condition} and the default {@link SyntaxNotation#LOGICAL}.
*
* @param aArgs The command line arguments to be evaluated.
* @param aArgsSyntax The root condition being the node from which parsing
* the command line arguments starts.
*
* @throws ArgsSyntaxException thrown in case of a command line arguments
* mismatch regarding provided and expected args.
*/
public ArgsParserProperties( String[] aArgs, Condition aArgsSyntax ) throws ArgsSyntaxException {
super( aArgsSyntax );
evalArgs( aArgs );
}
/**
* Constructs the {@link ParseArgsProperties} instance with the given root
* {@link Option} and the default {@link SyntaxNotation#LOGICAL}.
*
* @param aArgs The command line arguments to be evaluated.
* @param aArgsSyntax The root option being the node from which parsing the
* command line arguments starts.
*
* @throws ArgsSyntaxException thrown in case of a command line arguments
* mismatch regarding provided and expected args.
*/
public ArgsParserProperties( String[] aArgs, Option> aArgsSyntax ) throws ArgsSyntaxException {
super( aArgsSyntax );
evalArgs( aArgs );
}
/**
* Constructs the {@link ParseArgsProperties} instance with no syntax
* notation (no root {@link Condition}). As no syntax notation is required
* by the constructor (no root {@link Condition}), no syntax validation is
* done by {@link #evalArgs(String[])} and the like methods. When no syntax
* validation is fine for you, you can directly go for the constructor
* {@link #ArgsParserProperties(String[])}.
*
* @param aCliCtx The {@link CliContext} to be used for initializing.
*/
public ArgsParserProperties( CliContext aCliCtx ) {
super( aCliCtx );
}
/**
* Constructs the {@link ParseArgsProperties} instance with the given root
* {@link Term} and the default {@link SyntaxNotation#LOGICAL}.
*
* @param aArgsSyntax The args syntax root {@link Term} node being the node
* from which parsing the command line arguments starts.
* @param aCliCtx The {@link CliContext} to be used for initializing.
*/
public ArgsParserProperties( Term aArgsSyntax, CliContext aCliCtx ) {
super( aArgsSyntax, aCliCtx );
}
/**
* Constructs the {@link ParseArgsProperties} instance with the given
* arguments and the default {@link SyntaxNotation#LOGICAL}. As no syntax
* notation is required by the constructor (no root {@link Condition}), no
* syntax validation is done. Therefore the properties are heuristically
* determined from the provided command line arguments.
*
* @param aArgs The command line arguments to be evaluated.
* @param aCliCtx The {@link CliContext} to be used for initializing.
*/
public ArgsParserProperties( String[] aArgs, CliContext aCliCtx ) {
super( aCliCtx );
_properties = new PropertiesBuilderImpl( Arguments.toProperties( aArgs, aCliCtx.getSyntaxMetrics().toOptionPrefixes(), getDelimiter() ) );
}
/**
* Constructs the {@link ParseArgsProperties} instance with the given root
* {@link Condition} and the default {@link SyntaxNotation#LOGICAL}.
*
* @param aArgs The command line arguments to be evaluated.
* @param aArgsSyntax The root condition being the node from which parsing
* the command line arguments starts.
* @param aCliCtx The {@link CliContext} to be used for initializing.
*
* @throws ArgsSyntaxException thrown in case of a command line arguments
* mismatch regarding provided and expected args.
*/
public ArgsParserProperties( String[] aArgs, Condition aArgsSyntax, CliContext aCliCtx ) throws ArgsSyntaxException {
super( aArgsSyntax, aCliCtx );
evalArgs( aArgs );
}
/**
* Constructs the {@link ParseArgsProperties} instance with the given root
* {@link Option} and the default {@link SyntaxNotation#LOGICAL}.
*
* @param aArgs The command line arguments to be evaluated.
* @param aArgsSyntax The root option being the node from which parsing the
* command line arguments starts.
* @param aCliCtx The {@link CliContext} to be used for initializing.
*
* @throws ArgsSyntaxException thrown in case of a command line arguments
* mismatch regarding provided and expected args.
*/
public ArgsParserProperties( String[] aArgs, Option> aArgsSyntax, CliContext aCliCtx ) throws ArgsSyntaxException {
super( aArgsSyntax, aCliCtx );
evalArgs( aArgs );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Operand>[] evalArgs( String[] aArgs ) throws ArgsSyntaxException {
return evalArgs( getRootPath(), aArgs );
}
/**
* {@inheritDoc}
*/
@Override
public Operand>[] evalArgs( List aArgs ) throws ArgsSyntaxException {
return evalArgs( getRootPath(), aArgs.toArray( new String[aArgs.size()] ) );
}
/**
* {@inheritDoc}
*/
@Override
public Operand>[] evalArgs( String aToPath, List aArgs ) throws ArgsSyntaxException {
return evalArgs( aToPath, aArgs.toArray( new String[aArgs.size()] ) );
}
/**
* {@inheritDoc}
*/
@Override
public char getAnnotator() {
return _properties.getAnnotator();
}
/**
* {@inheritDoc}
*/
@Override
public char getDelimiter() {
return _properties.getDelimiter();
}
/**
* {@inheritDoc}
*/
@Override
public int size() {
return _properties.size();
}
/**
* {@inheritDoc}
*/
@Override
public boolean containsKey( Object aKey ) {
return _properties.containsKey( aKey );
}
/**
* {@inheritDoc}
*/
@Override
public boolean isEmpty() {
return _properties.isEmpty();
}
/**
* {@inheritDoc}
*/
@Override
public String get( Object aKey ) {
return _properties.get( aKey );
}
/**
* {@inheritDoc}
*/
@Override
public Set keySet() {
return _properties.keySet();
}
/**
* {@inheritDoc}
*/
@Override
public Collection values() {
return _properties.values();
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveFrom( String aFromPath ) {
return _properties.retrieveFrom( aFromPath );
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveTo( String aToPath ) {
return _properties.retrieveTo( aToPath );
}
/**
* {@inheritDoc}
*/
@Override
public Object toDataStructure( String aPath ) {
return _properties.toDataStructure( aPath );
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withAddExample( Example aExamples ) {
addExample( aExamples );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withAddExample( String aDescription, Operand>... aOperands ) {
addExample( aDescription, aOperands );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withArgsSyntax( Term aArgsSyntax ) {
setArgsSyntax( aArgsSyntax );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withBannerFontPalette( AsciiColorPalette aBannerFontPalette ) {
setBannerFontPalette( aBannerFontPalette );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withSyntaxMetrics( SyntaxNotation aSyntaxNotation ) {
setSyntaxMetrics( aSyntaxNotation );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withEvalArgs( String[] aArgs, Pattern aFilterExp ) throws ArgsSyntaxException {
evalArgs( aArgs, aFilterExp );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withEvalArgs( List aArgs, Pattern aFilterExp ) throws ArgsSyntaxException {
evalArgs( aArgs, aFilterExp );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withEvalArgs( String[] aArgs, ArgsFilter aArgsFilter ) throws ArgsSyntaxException {
evalArgs( aArgs, aArgsFilter );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withEvalArgs( List aArgs, ArgsFilter aArgsFilter ) throws ArgsSyntaxException {
evalArgs( aArgs, aArgsFilter );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withArgumentEscapeCode( String aParamEscCode ) {
setArgumentEscapeCode( aParamEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withBannerBorderEscapeCode( String aBannerBorderEscCode ) {
setBannerBorderEscapeCode( aBannerBorderEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withBannerEscapeCode( String aBannerEscCode ) {
setBannerEscapeCode( aBannerEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withBannerFont( Font aBannerFont ) {
setBannerFont( aBannerFont );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withBannerFontPalette( char[] aColorPalette ) {
setBannerFontPalette( aColorPalette );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withCommandEscapeCode( String aCommandEscCode ) {
setCommandEscapeCode( aCommandEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withConsoleWidth( int aConsoleWidth ) {
setConsoleWidth( aConsoleWidth );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withCopyright( String aCopyright ) {
setCopyright( aCopyright );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withDescription( String aDescription ) {
setDescription( aDescription );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withDescriptionEscapeCode( String aDescriptionEscCode ) {
setDescriptionEscapeCode( aDescriptionEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withEscapeCodesEnabled( boolean aIsEscCodeEnabled ) {
setEscapeCodesEnabled( aIsEscCodeEnabled );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withEvalArgs( List aArgs ) throws ArgsSyntaxException {
evalArgs( aArgs );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withEvalArgs( String[] aArgs ) throws ArgsSyntaxException {
evalArgs( aArgs );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withExamples( Collection aExamples ) {
setExamples( aExamples );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withExamples( Example[] aExamples ) {
setExamples( aExamples );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withLicense( String aLicense ) {
setLicense( aLicense );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withLineBreak( String aLineBreak ) {
setLineBreak( aLineBreak );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withLineSeparatorEscapeCode( String aLineSeparatorEscCode ) {
setLineSeparatorEscapeCode( aLineSeparatorEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withLongOptionPrefix( String aLongOptionPrefix ) {
setLongOptionPrefix( aLongOptionPrefix );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withMaxConsoleWidth( int aMaxConsoleWidth ) {
setMaxConsoleWidth( aMaxConsoleWidth );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withName( String aName ) {
setName( aName );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withOptionEscapeCode( String aOptEscCode ) {
setOptionEscapeCode( aOptEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withResetEscapeCode( String aResetEscCode ) {
setResetEscapeCode( aResetEscCode );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withShortOptionPrefix( Character aShortOptionPrefix ) {
setShortOptionPrefix( aShortOptionPrefix );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withSyntaxMetrics( SyntaxMetrics aSyntaxMetrics ) {
setSyntaxMetrics( aSyntaxMetrics );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withTextBoxGrid( TextBoxGrid aTextBoxGrid ) {
setTextBoxGrid( aTextBoxGrid );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withTextBoxGrid( TextBoxStyle aTextBoxStyle ) {
setTextBoxGrid( aTextBoxStyle );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withTitle( String aTitle ) {
setTitle( aTitle );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withSeparatorLnChar( char aSeparatorLnChar ) {
setSeparatorLnChar( aSeparatorLnChar );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withStandardOut( PrintStream aStandardOut ) {
setStandardOut( aStandardOut );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ArgsParserProperties withErrorOut( PrintStream aErrorOut ) {
setErrorOut( aErrorOut );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ParseArgsProperties withEvalArgs( String aToPath, List aArgs ) throws ArgsSyntaxException {
evalArgs( aToPath, aArgs );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ParseArgsProperties withEvalArgs( String aToPath, String[] aArgs ) throws ArgsSyntaxException {
evalArgs( aToPath, aArgs );
return this;
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Operand>[] evalArgs( String aToPath, String[] aArgs ) throws ArgsSyntaxException {
final Operand>[] theEvalArgs = super.evalArgs( aArgs );
final PropertiesBuilder theBuilder = new PropertiesBuilderImpl();
String eAlias;
for ( Operand> e : theEvalArgs ) {
if ( e.hasValue() ) {
eAlias = e.getAlias();
if ( eAlias == null ) {
if ( e instanceof Option> theOption ) {
eAlias = theOption.getLongOption() != null ? theOption.getLongOption() : ( theOption.getShortOption() != null ? theOption.getShortOption().toString() : "" );
}
}
theBuilder.add( toPath( aToPath, eAlias ), ( e.getValue() instanceof Enum> theEnum ) ? theEnum.name() : e.getValue().toString() );
}
}
_properties = theBuilder;
return theEvalArgs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy