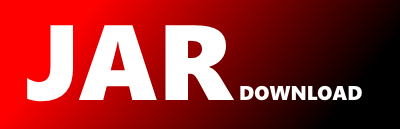
org.refcodes.properties.DocumentMetrics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.refcodes.data.Delimiters;
import org.refcodes.mixin.ArrayIndexAccessor;
import org.refcodes.mixin.DelimitersAccessor;
import org.refcodes.mixin.EnvelopeAccessor;
import org.refcodes.struct.CanonicalMap;
import org.refcodes.struct.ext.factory.DocumentProperty;
/**
* The {@link DocumentMetrics} describe various metrics which may be tweaked
* when marshaling or unmarshaling documents of various nations (such as INI,
* XML, YAML, JSON, TOML, PROPERTIES, etc.).
*/
public interface DocumentMetrics extends DelimitersAccessor, EnvelopeAccessor, ArrayIndexAccessor {
/**
* Creates properties when unmarshaling (marshaling) an object.
*
* @param aDelimiter The delimiter as of {@link CanonicalMap#getDelimiter()}
* of the {@link CanonicalMap} (or its sub-types) to be produced.
*
* @return The according properties instance.
*/
default Map toProperties( char aDelimiter ) {
final Map theProperties = new HashMap<>();
theProperties.put( DocumentProperty.DELIMITER.getKey(), aDelimiter + "" );
theProperties.put( DocumentProperty.DELIMITERS.getKey(), new String( toDelimiters( aDelimiter, getDelimiters(), Delimiters.PROPERTIES.getChars() ) ) );
theProperties.put( DocumentProperty.ENVELOPE.getKey(), Boolean.toString( isEnvelope() ) );
return theProperties;
}
/**
* Creates properties when marshaling (unmarshaling) an object.
*
* @param aComment The comment to be used for the marshaled representation.
* @param aDelimiter The (default) delimiter, usually as returned by the
* method {@link Properties#getDelimiter()}.
*
* @return The according properties instance.
*/
default Map toProperties( String aComment, char aDelimiter ) {
final Map theProperties = new HashMap<>();
theProperties.put( DocumentProperty.COMMENT.getKey(), aComment );
theProperties.put( DocumentProperty.DELIMITER.getKey(), aDelimiter + "" );
theProperties.put( DocumentProperty.ARRAY_INDEX.getKey(), Boolean.toString( isArrayIndex() ) );
theProperties.put( DocumentProperty.ENVELOPE.getKey(), Boolean.toString( isEnvelope() ) );
return theProperties;
}
/**
* Returns the provided (custom) delimiters or the (default) delimiters
* alongside the (default) delimiter.
*
* @param aDelimiter The (default) delimiter, usually as returned by the
* method {@link Properties#getDelimiter()}.
* @param aDelimiters The (custom) delimiters to use in case not null and
* not empty.
* @param aDefaultDelimiters The (default) delimiters, usually something
* like {@link Delimiters#PROPERTIES}.
*
* @return The resulting delimiters as of the method's logic.
*/
static char[] toDelimiters( char aDelimiter, char[] aDelimiters, final char[] aDefaultDelimiters ) { // Extracted method For testability!
if ( aDelimiters != null && aDelimiters.length != 0 ) {
return aDelimiters;
}
final List theChars = new LinkedList<>();
theChars.add( aDelimiter );
for ( char aDefaultDelimiter : aDefaultDelimiters ) {
if ( !theChars.contains( aDefaultDelimiter ) ) {
theChars.add( aDefaultDelimiter );
}
}
final char[] theDelimiters = new char[theChars.size()];
for ( int i = 0; i < theDelimiters.length; i++ ) {
theDelimiters[i] = theChars.get( i );
}
return theDelimiters;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy