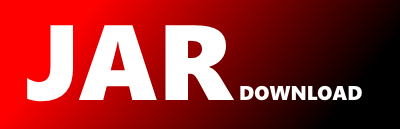
org.refcodes.properties.DocumentMetricsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import org.refcodes.data.Delimiters;
/**
* This class implements the {@link DocumentMetrics} interface and provides a
* {@link #builder()} for easily creating {@link DocumentMetrics} instances.
*/
public class DocumentMetricsImpl implements DocumentMetrics {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
private static final char[] DEFAULT_DELIMITERS = Delimiters.PROPERTIES.getChars();
private static final boolean DEFAULT_ENVELOPE = false;
private static final boolean DEFAULT_ARRAY_INDEX = false;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private final boolean _isArrayIndex;
private final boolean _isEnvelope;
private final char[] _delimiters;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
private DocumentMetricsImpl( Builder builder ) {
_isArrayIndex = builder.isArrayIndex;
_isEnvelope = builder.isEnvelope;
_delimiters = builder.delimiters;
}
/**
* Constructs the {@link DocumentMetricsImpl} instance with the default
* metrics being document's array index disabled, document's envelope
* disabled and delimiters set to {@link Delimiters#PROPERTIES}.
*/
public DocumentMetricsImpl() {
this( DEFAULT_ENVELOPE, DEFAULT_ARRAY_INDEX, DEFAULT_DELIMITERS );
}
/**
* Constructs the {@link DocumentMetricsImpl} instance with the according
* metrics being set.
*
* @param aEnvelope Determines whether unmarshaling (XML) documents will
* preserve the preserve the root element (isEnvelope) even when
* merely acting as an isEnvelope.
* @param aArrayIndex Determines whether marshaling (XML) documents will
* preserve array index information by using an index attribute for
* array elements in the produced XML.
*/
public DocumentMetricsImpl( boolean aEnvelope, boolean aArrayIndex ) {
this( aEnvelope, aArrayIndex, DEFAULT_DELIMITERS );
}
/**
* Constructs the {@link DocumentMetricsImpl} instance with the according
* metrics being set.
*
* @param aDelimiters The delimiters to be used when unmarshaling paths from
* properties' keys.
*/
public DocumentMetricsImpl( char... aDelimiters ) {
this( DEFAULT_ENVELOPE, DEFAULT_ARRAY_INDEX, aDelimiters );
}
/**
* Constructs the {@link DocumentMetricsImpl} instance with the according
* metrics being set.
*
* @param aEnvelope Determines whether unmarshaling (XML) documents will
* preserve the preserve the root element (isEnvelope) even when
* merely acting as an isEnvelope.
* @param aArrayIndex Determines whether marshaling (XML) documents will
* preserve array index information by using an index attribute for
* array elements in the produced XML.
* @param aDelimiters The delimiters to be used when unmarshaling paths from
* properties' keys.
*/
public DocumentMetricsImpl( boolean aEnvelope, boolean aArrayIndex, char... aDelimiters ) {
_isEnvelope = aEnvelope;
_isArrayIndex = aArrayIndex;
_delimiters = aDelimiters;
}
// /////////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean isArrayIndex() {
return _isArrayIndex;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isEnvelope() {
return _isEnvelope;
}
/**
* {@inheritDoc}
*/
@Override
public char[] getDelimiters() {
return _delimiters;
}
/**
* This methods provides a {@link Builder} instance for creating
* {@link DocumentMetrics} instances.
*
* @return The according {@link Builder} instance.
*/
public static Builder builder() {
return new Builder();
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* {@link Builder} for constructing {@link DocumentMetricsImpl} instances.
*/
public static final class Builder implements ArrayIndexBuilder, EnvelopeBuilder, DelimitersBuilder {
private boolean isArrayIndex = DEFAULT_ARRAY_INDEX;
private boolean isEnvelope = DEFAULT_ENVELOPE;
private char[] delimiters = DEFAULT_DELIMITERS;
private Builder() {}
/**
* {@inheritDoc}
*/
@Override
public Builder withArrayIndex( boolean isArrayIndex ) {
this.isArrayIndex = isArrayIndex;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public Builder withEnvelope( boolean isEnvelope ) {
this.isEnvelope = isEnvelope;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public Builder withDelimiters( char... delimiters ) {
this.delimiters = delimiters;
return this;
}
/**
* Builds the.
*
* @return the document metrics
*/
public DocumentMetrics build() {
return new DocumentMetricsImpl( this );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy