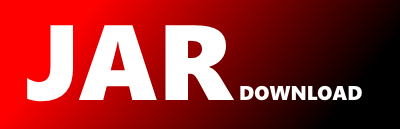
org.refcodes.properties.EnvironmentProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.refcodes.data.Delimiter;
import org.refcodes.struct.StructureUtility;
/**
* Extension of the {@link Properties} type overwriting methods in order to
* access the operating system's environment variables as of env
on
* Linux or Unix shells or set
on Windows machines (e.g."set
* JAVA_HOME=/opt/java9"). The keys are transformed to an environment variable
* by removing a prefixed "/" path delimiter (as of {@link #getDelimiter()} and
* converting all other path delimiters "/" to the environment variable's (de
* facto standard) separator "_". If accessing failed, then the upper case
* version of the so transformed key is probed. Accessing an environment
* variable "JAVA_HOME" would be done with the path "/java/home".
*/
public class EnvironmentProperties implements Properties {
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* The key is transformed to an environment variable by removing a prefixed
* "/" path delimiter (as of {@link #getDelimiter()} and converting all
* other path delimiters "/" to the environment variable's (de facto
* standard) separator "_". If accessing failed, then the upper case version
* of the so transformed key is probed. {@inheritDoc}
*/
@Override
public boolean containsKey( Object aKey ) {
return get( aKey ) != null;
}
/**
* The key is transformed to an environment variable by removing a prefixed
* "/" path delimiter (as of {@link #getDelimiter()} and converting all
* other path delimiters "/" to the environment variable's (de facto
* standard) separator "_". If accessing failed, then the upper case version
* of the so transformed key is probed. {@inheritDoc}
*/
@Override
public String get( Object aKey ) {
String theValue = null;
String theKey = aKey != null ? aKey.toString() : null;
if ( theKey != null ) {
theKey = NormalizedPropertiesDecorator.fromNormalized( theKey, getDelimiter(), Delimiter.SNAKE_CASE.getChar() );
theValue = System.getenv( theKey );
if ( theValue == null ) {
theValue = System.getenv( theKey.toUpperCase() );
if ( theValue == null ) {
for ( String eKey : System.getenv().keySet() ) {
if ( eKey.equalsIgnoreCase( theKey ) ) {
return System.getenv( eKey );
}
}
}
}
}
return theValue;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isEmpty() {
return System.getProperties().isEmpty();
}
/**
* The keys are transformed to a path by prefixing a "/" path delimiter (as
* of {@link #getDelimiter()} and converting all other environment
* variable's (de facto standard) separators "_" to the path delimiter "/".
* {@inheritDoc}
*/
@Override
public Set keySet() {
final Set theKeys = new HashSet<>();
final Map theProperties = System.getenv();
for ( Object eKey : theProperties.keySet() ) {
theKeys.add( NormalizedPropertiesDecorator.toNormalized( eKey.toString(), getDelimiter(), Delimiter.SNAKE_CASE.getChar() ).toLowerCase() );
}
return theKeys;
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveFrom( String aFromPath ) {
final PropertiesBuilder theToPathMap = new PropertiesBuilderImpl();
StructureUtility.retrieveFrom( this, aFromPath, theToPathMap );
return theToPathMap;
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveTo( String aToPath ) {
final PropertiesBuilder theToPathMap = new PropertiesBuilderImpl();
StructureUtility.retrieveTo( this, aToPath, theToPathMap );
return theToPathMap;
}
/**
* {@inheritDoc}
*/
@Override
public int size() {
return System.getenv().size();
}
/**
* {@inheritDoc}
*/
@Override
public Object toDataStructure( String aFromPath ) {
return StructureUtility.toDataStructure( this, aFromPath );
}
/**
* {@inheritDoc}
*/
@Override
public Collection values() {
final List theValues = new ArrayList<>();
final Map theProperties = System.getenv();
for ( Object eValue : theProperties.values() ) {
theValues.add( eValue.toString() );
}
return theValues;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy