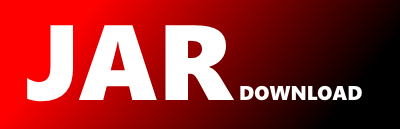
org.refcodes.properties.ProfileProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.util.Collection;
import org.refcodes.data.Delimiter;
import org.refcodes.struct.PathMap;
import org.refcodes.struct.Property;
import org.refcodes.struct.Relation;
/**
* The {@link ProfileProperties} extend the {@link Properties} with
* Runtime-Profiles support. Properties belonging to provided Runtime-Profiles
* overwrite the default properties. Use {@link #toRuntimeProfile()} to generate
* accordingly processed Runtime-Profiles with Runtime-Profiles defined below
* the {@link #getRuntimeProfilesPath()}, which by default is
* "/runtime/profiles". As more than one profile can be evaluated (a preceding
* profile overwriting succeeding profile), the
* {@link #getRuntimeProfilesPath()} points to an array with the
* Runtime-Profiles denoted as follows (assuming "/runtime/profiles" containing
* your active Runtime-Profiles):
*
* - runtime/profiles/0=local
* - runtime/profiles/1=oauth
* - runtime/profiles/2=development
*
* ({@link #getRuntimeProfilesPath()} defaults to "runtime/profiles" represented
* by the path defined by {@link PropertiesPath#RUNTIME_PROFILES_PATH}) In case
* a value is found at the path "runtime/profiles", this is parsed as a
* comma-separated list and converted to an array. The above configuration
* therewith may also be written as follows:
* runtime/profiles=local,oauth,development
This array beats the
* above kind of declaration so that the active Runtime-Profiles can easily be
* overwritten by {@link ProfileProperties} supporting Java System-Properties
* (passed to the JVM via "-Dx=y") e.g. the
* ApplicationPropertiesComposite
found in the
* refcodes-runtime
artifact. Below find an example on properties
* with settings for the above Runtime-Profiles:
*
* - db_url=someStagingDbUrl
* - db_user=admin
* - security=false
* - oauth/security=true
* - local/db_user=devops
* - local/db_url=someLocalDbUrl
* - development/db_user=admin
* - development/db_url=someDevelopmentDbUrl
* - test/db_user=test
* - test/db_url=someTestDbUrl
*
* In the above example, the default settings would be:
*
* - db_url=someStagingDbUrl
* - db_user=admin
*
* The default settings are replaced / enriched by the settings found in the
* "local" profile, any settings not found for the "local" profile are
* overwritten / enriched by the "oauth" profile. Any settings not found in the
* "oauth" profile (and not found in the "local" profile") are overwritten /
* enriched by the "development" profile. The properties created from the active
* Runtime-Profiles specified below the path "runtime/profiles" via
* {@link #toRuntimeProfile()} result in the below default properties:
*
* - /db_url:= someLocalDbUrl
* - /db_user:= devops
* - /security:= true
*
*/
public interface ProfileProperties extends Properties {
/**
* The {@link #getRuntimeProfilesPath()} points to an array with the
* Runtime-Profiles denoted as follows (pointing to your active
* Runtime-Profiles):
*
* - runtime/profiles/0=local
* - runtime/profiles/1=oauth
* - runtime/profiles/2=development
*
* ({@link #getRuntimeProfilesPath()} defaults to "runtime/profiles"
* represented by the path defined by
* {@link PropertiesPath#RUNTIME_PROFILES_PATH}) In case a value is found at
* the path "runtime/profiles", this is parsed as a comma-separated list and
* converted to an array. The above configuration therewith may also be
* written as follows: runtime/profiles=local,oauth,development
* This array beats the above kind of declaration so that the active
* Runtime-Profiles can easily be overwritten by {@link ProfileProperties}
* supporting Java System-Properties (passed to the JVM via "-Dx=y") e.g.
* the ApplicationPropertiesComposite
found in the
* refcodes-runtime
artifact.
*
* @return Your active Runtime-Profiles defined at the
* {@link #getRuntimeProfilesPath()}.
*/
default String[] getRuntimeProfiles() {
final String theRecord = get( getRuntimeProfilesPath() );
if ( theRecord != null ) {
final String[] theProfiles = theRecord.split( Delimiter.LIST.getChar() + "" );
if ( theProfiles != null && theProfiles.length != 0 ) {
return theProfiles;
}
}
return getArray( getRuntimeProfilesPath() );
}
/**
* Returns the path which points to your active Runtime-Profiles. The method
* {@link #getRuntimeProfiles()} evaluates the path and creates a list of
* active Runtime-Profiles. By default, the path points to the path
* represented by {@link PropertiesPath#RUNTIME_PROFILES_PATH}.
*
* @return The path pointing to your active Runtime-Profiles, defaults to
* "runtime/profiles".
*/
default String getRuntimeProfilesPath() {
return PropertiesPath.RUNTIME_PROFILES_PATH.getPath();
}
/**
* Evaluates the active Runtime-Profiles as of {@link #getRuntimeProfiles()}
* and creates the according {@link Properties}.
*
* @return The {@link Properties} as of the active Runtime-Profiles.
*/
default Properties toRuntimeProfile() {
return toRuntimeProfile( getRuntimeProfiles() );
}
/**
* Evaluates the provided Runtime-Profiles and creates the according
* {@link Properties}.
*
* @param aProfiles the profiles
*
* @return The {@link Properties} as of the provided Runtime-Profiles.
*/
default Properties toRuntimeProfile( String... aProfiles ) {
final PropertiesBuilder theProperties = new PropertiesBuilderImpl( this );
String eKey;
if ( aProfiles != null ) {
for ( int i = aProfiles.length - 1; i >= 0; i-- ) {
eKey = aProfiles[i];
if ( eKey != null && eKey.length() != 0 ) {
// theProperties.removeFrom( eKey );
final PathMap theRetrieved = retrieveFrom( eKey );
theProperties.insert( theRetrieved );
}
}
}
return new PropertiesImpl( (Properties) theProperties );
}
// /////////////////////////////////////////////////////////////////////////
// MUTATOR:
// /////////////////////////////////////////////////////////////////////////
/**
* The Interface MutableProfileProperties.
*/
public interface MutableProfileProperties extends ProfileProperties, MutableProperties {}
// /////////////////////////////////////////////////////////////////////////
// BUILDER:
// /////////////////////////////////////////////////////////////////////////
/**
* The Interface ProfilePropertiesBuilder.
*/
public interface ProfilePropertiesBuilder extends MutableProfileProperties, PropertiesBuilder {
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsert( Object aObj ) {
insert( aObj );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsert( PathMap aFrom ) {
insert( aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( Collection> aToPathElements, Object aFrom, Collection> aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( Collection> aToPathElements, PathMap aFrom, Collection> aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
// /**
// * {@inheritDoc}
// */
// @Override
// default ProfilePropertiesBuilder withPut( Object aPath, String aValue ) {
// put( toPath( aPath ), aValue );
// return this;
// }
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( Object aToPath, Object aFrom, Object aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( Object aToPath, PathMap aFrom, Object aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( Object[] aToPathElements, Object aFrom, Object[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( Object[] aToPathElements, PathMap aFrom, Object[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( String aToPath, Object aFrom, String aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( String aToPath, PathMap aFrom, String aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( String[] aToPathElements, Object aFrom, String[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertBetween( String[] aToPathElements, PathMap aFrom, String[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( Object aFrom, Collection> aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( Object aFrom, Object aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( Object aFrom, Object... aFromPathElements ) {
withInsertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( Object aFrom, String aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( Object aFrom, String... aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( PathMap aFrom, Collection> aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( PathMap aFrom, Object aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( PathMap aFrom, Object... aFromPathElements ) {
withInsertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( PathMap aFrom, String aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertFrom( PathMap aFrom, String... aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( Collection> aToPathElements, Object aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( Collection> aToPathElements, PathMap aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( Object aToPath, Object aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( Object aToPath, PathMap aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( Object[] aToPathElements, Object aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( Object[] aToPathElements, PathMap aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( String aToPath, Object aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( String aToPath, PathMap aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( String[] aToPathElements, Object aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withInsertTo( String[] aToPathElements, PathMap aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMerge( Object aObj ) {
merge( aObj );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMerge( PathMap aFrom ) {
merge( aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( Collection> aToPathElements, Object aFrom, Collection> aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( Collection> aToPathElements, PathMap aFrom, Collection> aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( Object aToPath, Object aFrom, Object aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( Object aToPath, PathMap aFrom, Object aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( Object[] aToPathElements, Object aFrom, Object[] aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( Object[] aToPathElements, PathMap aFrom, Object[] aFromPathElements ) {
mergeBetween( aToPathElements, aFromPathElements, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( String aToPath, Object aFrom, String aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( String aToPath, PathMap aFrom, String aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( String[] aToPathElements, Object aFrom, String[] aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeBetween( String[] aToPathElements, PathMap aFrom, String[] aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( Object aFrom, Collection> aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( Object aFrom, Object aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( Object aFrom, Object... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( Object aFrom, String aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( Object aFrom, String... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( PathMap aFrom, Collection> aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( PathMap aFrom, Object aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( PathMap aFrom, Object... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( PathMap aFrom, String aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeFrom( PathMap aFrom, String... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( Collection> aToPathElements, Object aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( Collection> aToPathElements, PathMap aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( Object aToPath, Object aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( Object aToPath, PathMap aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( Object[] aToPathElements, Object aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( Object[] aToPathElements, PathMap aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( String aToPath, Object aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( String aToPath, PathMap aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( String[] aToPathElements, Object aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withMergeTo( String[] aToPathElements, PathMap aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPut( Collection> aPathElements, String aValue ) {
put( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPut( Object[] aPathElements, String aValue ) {
put( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPut( Property aProperty ) {
put( aProperty );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPut( Relation aProperty ) {
put( aProperty );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPut( String aKey, String aValue ) {
put( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPut( String[] aKey, String aValue ) {
put( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutBoolean( Collection> aPathElements, Boolean aValue ) {
putBoolean( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutBoolean( Object aKey, Boolean aValue ) {
putBoolean( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutBoolean( Object[] aPathElements, Boolean aValue ) {
putBoolean( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutBoolean( String aKey, Boolean aValue ) {
putBoolean( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutBoolean( String[] aPathElements, Boolean aValue ) {
putBoolean( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutByte( Collection> aPathElements, Byte aValue ) {
putByte( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutByte( Object aKey, Byte aValue ) {
putByte( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutByte( Object[] aPathElements, Byte aValue ) {
putByte( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutByte( String aKey, Byte aValue ) {
putByte( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutByte( String[] aPathElements, Byte aValue ) {
putByte( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutChar( Collection> aPathElements, Character aValue ) {
putChar( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutChar( Object aKey, Character aValue ) {
putChar( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutChar( Object[] aPathElements, Character aValue ) {
putChar( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutChar( String aKey, Character aValue ) {
putChar( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutChar( String[] aPathElements, Character aValue ) {
putChar( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutClass( Collection> aPathElements, Class aValue ) {
putClass( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutClass( Object aKey, Class aValue ) {
putClass( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutClass( Object[] aPathElements, Class aValue ) {
putClass( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutClass( String aKey, Class aValue ) {
putClass( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutClass( String[] aPathElements, Class aValue ) {
putClass( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( Collection> aPathElements, int aIndex, Object aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( Collection> aPathElements, int aIndex, PathMap aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( int aIndex, Object aDir ) {
putDirAt( aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( int aIndex, PathMap aDir ) {
putDirAt( aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( Object aPath, int aIndex, Object aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( Object aPath, int aIndex, PathMap aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( Object[] aPathElements, int aIndex, Object aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( Object[] aPathElements, int aIndex, PathMap aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( String aPath, int aIndex, Object aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( String aPath, int aIndex, PathMap aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( String[] aPathElements, int aIndex, Object aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDirAt( String[] aPathElements, int aIndex, PathMap aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDouble( Collection> aPathElements, Double aValue ) {
putDouble( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDouble( Object aKey, Double aValue ) {
putDouble( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDouble( Object[] aPathElements, Double aValue ) {
putDouble( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDouble( String aKey, Double aValue ) {
putDouble( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutDouble( String[] aPathElements, Double aValue ) {
putDouble( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ProfilePropertiesBuilder withPutEnum( Collection> aPathElements, E aValue ) {
putEnum( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ProfilePropertiesBuilder withPutEnum( Object aKey, E aValue ) {
putEnum( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ProfilePropertiesBuilder withPutEnum( Object[] aPathElements, E aValue ) {
putEnum( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ProfilePropertiesBuilder withPutEnum( String aKey, E aValue ) {
putEnum( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ProfilePropertiesBuilder withPutEnum( String[] aPathElements, E aValue ) {
putEnum( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutFloat( Collection> aPathElements, Float aValue ) {
putFloat( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutFloat( Object aKey, Float aValue ) {
putFloat( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutFloat( Object[] aPathElements, Float aValue ) {
putFloat( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutFloat( String aKey, Float aValue ) {
putFloat( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutFloat( String[] aPathElements, Float aValue ) {
putFloat( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutInt( Collection> aPathElements, Integer aValue ) {
putInt( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutInt( Object aKey, Integer aValue ) {
putInt( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutInt( Object[] aPathElements, Integer aValue ) {
putInt( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutInt( String aKey, Integer aValue ) {
putInt( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutInt( String[] aPathElements, Integer aValue ) {
putInt( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutLong( Collection> aPathElements, Long aValue ) {
putLong( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutLong( Object aKey, Long aValue ) {
putLong( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutLong( Object[] aPathElements, Long aValue ) {
putLong( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutLong( String aKey, Long aValue ) {
putLong( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutLong( String[] aPathElements, Long aValue ) {
putLong( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutShort( Collection> aPathElements, Short aValue ) {
putShort( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutShort( Object aKey, Short aValue ) {
putShort( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutShort( Object[] aPathElements, Short aValue ) {
putShort( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutShort( String aKey, Short aValue ) {
putShort( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutShort( String[] aPathElements, Short aValue ) {
putShort( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutString( Collection> aPathElements, String aValue ) {
putString( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutString( Object aKey, String aValue ) {
putString( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutString( Object[] aPathElements, String aValue ) {
putString( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutString( String aKey, String aValue ) {
putString( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withPutString( String[] aPathElements, String aValue ) {
putString( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withRemoveFrom( Collection> aPathElements ) {
removeFrom( aPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withRemoveFrom( Object aPath ) {
removeFrom( aPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withRemoveFrom( Object... aPathElements ) {
removeFrom( aPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withRemoveFrom( String aPath ) {
removeFrom( aPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withRemoveFrom( String... aPathElements ) {
removeFrom( aPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ProfilePropertiesBuilder withRemovePaths( String... aPathElements ) {
removeFrom( aPathElements );
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy