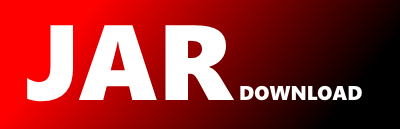
org.refcodes.properties.ProfilePropertiesProjection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.util.Collection;
import java.util.Set;
/**
* The {@link ProfilePropertiesProjection} applies the profiles as of
* {@link ProfileProperties#getRuntimeProfiles()} onto the encapsulated
* {@link Properties} ({@link ProfileProperties}) and provides a view of them
* {@link Properties} ({@link ProfileProperties}) from the profiles' point of
* view e.g. as when profiles have been applied to them {@link Properties}
* ({@link ProfileProperties}). In other words, the profiles are resolved and
* the result is provided as view: Under the hood, the provided
* {@link Properties} are decorated with a {@link ProfilePropertiesDecorator}
* which then is used to provide the profile projection via
* {@link ProfileProperties#toRuntimeProfile()}.
*/
public class ProfilePropertiesProjection implements Properties {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private final ProfileProperties _properties;
private String[] _profiles = null;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Uses the {@link ProfileProperties} for the profile projection. The
* {@link ProfileProperties} provide the profile projection via
* {@link ProfileProperties#toRuntimeProfile()}.
*
* @param aProperties The properties which provide the according profile
* projections applying the therein set profiles. The evaluation is
* done as of {@link ProfileProperties#toRuntimeProfile(String...)}.
*/
public ProfilePropertiesProjection( ProfileProperties aProperties ) {
_properties = aProperties;
}
/**
* Uses the {@link ProfileProperties} for the profile projection. The
* {@link ProfileProperties} provide the profile projection via
* {@link ProfileProperties#toRuntimeProfile(String...)} using the provided
* profiles
*
* @param aProperties The properties which are to be projected according to
* the therein set profiles. The evaluation is done as of
* {@link ProfileProperties#toRuntimeProfile(String...)}.
* @param aProfiles The profiles to be used when constructing the
* projection.
*/
public ProfilePropertiesProjection( ProfileProperties aProperties, String... aProfiles ) {
_properties = aProperties;
if ( aProfiles != null && aProfiles.length > 0 ) {
_profiles = aProfiles;
}
}
/**
* Wraps the {@link Properties} with a profile projection. The
* {@link Properties} are decorated with a
* {@link ProfilePropertiesDecorator} which provides the projected
* {@link Properties} from the wrapped {@link Properties} with the given
* profiles applied. See also
* {@link ProfileProperties#toRuntimeProfile(String...)}.
*
* @param aProperties The properties which are to be projected according to
* the provided profiles. The evaluation is done as of
* {@link ProfileProperties#toRuntimeProfile(String...)}.
* @param aProfiles The profiles to be used when constructing the
* projection.
*/
public ProfilePropertiesProjection( Properties aProperties, String... aProfiles ) {
_properties = new ProfilePropertiesDecorator( aProperties );
if ( aProfiles != null && aProfiles.length > 0 ) {
_profiles = aProfiles;
}
}
/**
* Wraps the {@link PropertiesBuilder} with a profile projection. The
* {@link PropertiesBuilder} are decorated with a
* {@link ProfilePropertiesDecorator} which then is used to provide the
* profile projection via
* {@link ProfileProperties#toRuntimeProfile(String...)} using the provided
* profiles
*
* @param aProperties The properties which are to be projected according to
* the therein set profiles. The evaluation is done as of
* {@link ProfileProperties#toRuntimeProfile(String...)}.
* @param aProfiles The profiles to be used when constructing the
* projection.
*/
public ProfilePropertiesProjection( PropertiesBuilder aProperties, String... aProfiles ) {
_properties = new ProfilePropertiesDecorator( aProperties );
if ( aProfiles != null && aProfiles.length > 0 ) {
_profiles = aProfiles;
}
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean containsKey( Object aKey ) {
return toRuntimeProfile().containsKey( aKey );
}
/**
* {@inheritDoc}
*/
@Override
public String get( Object aKey ) {
return toRuntimeProfile().get( aKey );
}
/**
* {@inheritDoc}
*/
@Override
public char getDelimiter() {
return toRuntimeProfile().getDelimiter();
}
/**
* {@inheritDoc}
*/
@Override
public boolean isEmpty() {
return toRuntimeProfile().isEmpty();
}
/**
* {@inheritDoc}
*/
@Override
public Set keySet() {
return toRuntimeProfile().keySet();
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveFrom( String aFromPath ) {
return toRuntimeProfile().retrieveFrom( aFromPath );
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveTo( String aToPath ) {
return toRuntimeProfile().retrieveTo( aToPath );
}
/**
* {@inheritDoc}
*/
@Override
public int size() {
return toRuntimeProfile().size();
}
/**
* {@inheritDoc}
*/
@Override
public Object toDataStructure( String aFromPath ) {
return toRuntimeProfile().toDataStructure( aFromPath );
}
/**
* {@inheritDoc}
*/
@Override
public Collection values() {
return toRuntimeProfile().values();
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
private Properties toRuntimeProfile() {
if ( _profiles == null || _profiles.length == 0 ) {
return _properties.toRuntimeProfile();
}
return _properties.toRuntimeProfile( _profiles );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy