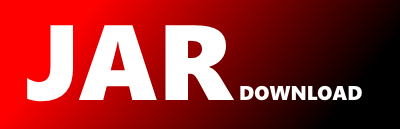
org.refcodes.properties.PropertiesBuilderImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.refcodes.data.Delimiter;
import org.refcodes.properties.Properties.PropertiesBuilder;
import org.refcodes.struct.CanonicalMapBuilderImpl;
import org.refcodes.struct.Property;
/**
* The Class PropertiesBuilderImpl.
*/
public class PropertiesBuilderImpl extends CanonicalMapBuilderImpl implements PropertiesBuilder {
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Create an empty {@link PropertiesBuilder} instance using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*/
public PropertiesBuilderImpl() {}
/**
* Create a {@link PropertiesBuilder} instance containing the elements of
* the provided {@link Map} instance using the default path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public PropertiesBuilderImpl( Map, ?> aProperties ) {
super( aProperties );
}
/**
* Create a {@link PropertiesBuilder} instance containing the elements as of
* {@link MutablePathMap#insert(Object)} using the default path delimiter
* "/" ({@link Delimiter#PATH}) for the path declarations: "Inspects the
* given object and adds all elements found in the given object. Elements of
* type {@link Map}, {@link Collection} and arrays are identified and
* handled as of their type: The path for each value in a {@link Map} is
* appended with its according key. The path for each value in a
* {@link Collection} or array is appended with its according index of
* occurrence (in case of a {@link List} or an array, its actual index). In
* case of reflection, the path for each member is appended with its
* according mamber's name. All elements (e.g. the members and values) are
* inspected recursively which results in the according paths of the
* terminating values."
*
* @param aObj The object from which the elements are to be added.
*/
public PropertiesBuilderImpl( Object aObj ) {
super( aObj );
}
/**
* Create a {@link PropertiesBuilder} instance containing the elements of
* the provided {@link Properties} instance using the default path delimiter
* "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public PropertiesBuilderImpl( Properties aProperties ) {
for ( String eKey : aProperties.keySet() ) {
put( eKey, aProperties.get( eKey ) );
}
}
/**
* Create a {@link PropertiesBuilder} instance containing the elements of
* the provided {@link Properties} instance using the default path delimiter
* "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public PropertiesBuilderImpl( PropertiesBuilder aProperties ) {
this( (Properties) aProperties );
}
/**
* Create a {@link PropertiesBuilder} instance containing the provided
* {@link Property} instances' key/value-pairs.
*
* @param aProperties the properties to be added.
*/
public PropertiesBuilderImpl( Property... aProperties ) {
for ( Property eProperty : aProperties ) {
put( eProperty );
}
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public PropertiesBuilder retrieveFrom( String aFromPath ) {
return new PropertiesBuilderImpl( super.retrieveFrom( aFromPath ) );
}
/**
* {@inheritDoc}
*/
@Override
public PropertiesBuilder retrieveTo( String aToPath ) {
return new PropertiesBuilderImpl( super.retrieveTo( aToPath ) );
}
/**
* {@inheritDoc}
*/
@Override
public PropertiesBuilder withPut( String aKey, String aValue ) {
put( aKey, aValue );
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy