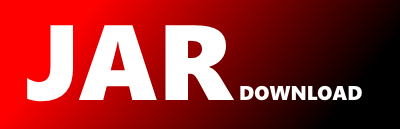
org.refcodes.properties.PropertiesImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.refcodes.data.Delimiter;
import org.refcodes.struct.PathMap.MutablePathMap;
import org.refcodes.struct.Property;
/**
* The Class PropertiesImpl.
*/
public class PropertiesImpl implements Properties {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
protected PropertiesBuilder _properties;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates an empty immutable {@link Properties} object.
*/
public PropertiesImpl() {
_properties = new PropertiesBuilderImpl();
}
/**
* Create a {@link Properties} instance containing the elements of the
* provided {@link Map} instance using the default path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public PropertiesImpl( Map, ?> aProperties ) {
_properties = new PropertiesBuilderImpl( aProperties );
}
/**
* Create a {@link Properties} instance containing the elements as of
* {@link MutablePathMap#insert(Object)} using the default path delimiter
* "/" ({@link Delimiter#PATH}) for the path declarations: "Inspects the
* given object and adds all elements found in the given object. Elements of
* type {@link Map}, {@link Collection} and arrays are identified and
* handled as of their type: The path for each value in a {@link Map} is
* appended with its according key. The path for each value in a
* {@link Collection} or array is appended with its according index of
* occurrence (in case of a {@link List} or an array, its actual index). In
* case of reflection, the path for each member is appended with its
* according mamber's name. All elements (e.g. the members and values) are
* inspected recursively which results in the according paths of the
* terminating values."
*
* @param aObj The object from which the elements are to be added.
*/
public PropertiesImpl( Object aObj ) {
_properties = new PropertiesBuilderImpl( aObj );
}
/**
* Create a {@link Properties} instance containing the elements of the
* provided {@link Properties} instance using the default path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public PropertiesImpl( Properties aProperties ) {
_properties = new PropertiesBuilderImpl( aProperties );
}
/**
* Create a {@link Properties} instance containing the provided
* {@link Property} instances' key/value-pairs.
*
* @param aProperties the properties to be added.
*/
public PropertiesImpl( Property... aProperties ) {
_properties = new PropertiesBuilderImpl( aProperties );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean containsKey( Object aKey ) {
return _properties.containsKey( aKey );
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) {
return true;
}
if ( obj == null ) {
return false;
}
if ( getClass() != obj.getClass() ) {
return false;
}
final PropertiesImpl other = (PropertiesImpl) obj;
if ( _properties == null ) {
if ( other._properties != null ) {
return false;
}
}
else if ( !_properties.equals( other._properties ) ) {
return false;
}
return true;
}
/**
* {@inheritDoc}
*/
@Override
public String get( Object aKey ) {
return _properties.get( aKey );
}
/**
* {@inheritDoc}
*/
@Override
public char getDelimiter() {
return _properties.getDelimiter();
}
/**
* {@inheritDoc}
*/
@Override
public Class getType() {
return _properties.getType();
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ( ( _properties == null ) ? 0 : _properties.hashCode() );
return result;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isEmpty() {
return _properties.isEmpty();
}
/**
* {@inheritDoc}
*/
@Override
public Set keySet() {
return _properties.keySet();
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveFrom( String aFromPath ) {
return _properties.retrieveFrom( aFromPath );
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveTo( String aToPath ) {
return _properties.retrieveTo( aToPath );
}
/**
* {@inheritDoc}
*/
@Override
public int size() {
return _properties.size();
}
/**
* {@inheritDoc}
*/
@Override
public Object toDataStructure( String aFromPath ) {
return _properties.toDataStructure( aFromPath );
}
/**
* {@inheritDoc}
*/
@Override
public Collection values() {
return _properties.values();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy