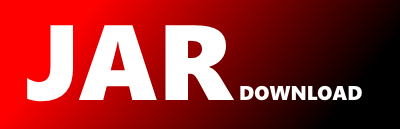
org.refcodes.properties.PropertiesNotationAccessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
/**
* Provides an accessor for a {@link PropertiesNotation} property.
*/
public interface PropertiesNotationAccessor {
/**
* Retrieves the properties notation from the {@link PropertiesNotation}
* property.
*
* @return The properties notation stored by the {@link PropertiesNotation}
* property.
*/
PropertiesNotation getPropertiesNotation();
/**
* Provides a builder method for a {@link PropertiesNotation} property
* returning the builder for applying multiple build operations.
*
* @param The builder to return in order to be able to apply multiple
* build operations.
*/
public interface PropertiesNotationBuilder> {
/**
* Sets the properties notation for the {@link PropertiesNotation}
* property.
*
* @param aPropertiesNotation The properties notation to be stored by
* the {@link PropertiesNotation} property.
*
* @return The builder for applying multiple build operations.
*/
B withPropertiesNotation( PropertiesNotation aPropertiesNotation );
}
/**
* Provides a mutator for a {@link PropertiesNotation} property.
*/
public interface PropertiesNotationMutator {
/**
* Sets the properties notation for the {@link PropertiesNotation}
* property.
*
* @param aPropertiesNotation The properties notation to be stored by
* the {@link PropertiesNotation} property.
*/
void setPropertiesNotation( PropertiesNotation aPropertiesNotation );
}
/**
* Provides a {@link PropertiesNotation} property.
*/
public interface PropertiesNotationProperty extends PropertiesNotationAccessor, PropertiesNotationMutator {
/**
* This method stores and passes through the given argument, which is
* very useful for builder APIs: Sets the given
* {@link PropertiesNotation} (setter) as of
* {@link #setPropertiesNotation(PropertiesNotation)} and returns the
* very same value (getter).
*
* @param aPropertiesNotation The {@link PropertiesNotation} to set (via
* {@link #setPropertiesNotation(PropertiesNotation)}).
*
* @return Returns the value passed for it to be used in conclusive
* processing steps.
*/
default PropertiesNotation letPropertiesNotation( PropertiesNotation aPropertiesNotation ) {
setPropertiesNotation( aPropertiesNotation );
return aPropertiesNotation;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy