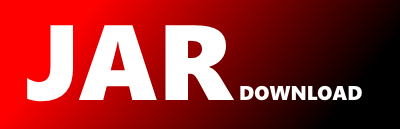
org.refcodes.properties.PropertiesPrecedence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
/**
* Defines a meta-interface in order to retrieve properties from various
* different properties sources ({@link Properties} instances) by querying all
* the herein contained {@link Properties} instances in the order of them being
* added. Queried properties of the first {@link Properties} instance containing
* them are returned. {@link Properties} instances before have a higher
* precedence than the instances added next.
*/
public interface PropertiesPrecedence extends Properties {
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Tests whether the given properties have already been added.
*
* @param aProperties The {@link Properties} to be tested for being already
* contained or not.
*
* @return True if the given {@link Properties} are already contained in the
* {@link PropertiesPrecedence} instance.
*/
boolean containsProperties( Properties aProperties );
// /////////////////////////////////////////////////////////////////////////
// MUTATOR:
// /////////////////////////////////////////////////////////////////////////
/**
* The interface {@link MutablePropertiesPrecedence} defines "dirty" methods
* allowing to modify ("mutate") the {@link PropertiesPrecedence}: Add or
* remove {@link Properties} inside a {@link PropertiesPrecedence} instance.
*/
public interface MutablePropertiesPrecedence extends PropertiesPrecedence {
/**
* Appends the provided {@link Properties} to the end of the precedence
* list, meaning that the added {@link Properties} have lowest priority.
* Them therein contained properties will lose in favor of properties
* (with the same key) from preceding {@link Properties}.
* {@link Properties} can only be added once!
*
* @param aProperties The properties to be appended.
*
* @return True in case the {@link Properties} have been added, false if
* them have already been added before.
*/
boolean appendProperties( Properties aProperties );
/**
* Prepends the provided {@link Properties} to beginning of the
* precedence list, meaning that the added {@link Properties} have
* highest priority. Them therein contained properties will rule out
* properties (with the same key) of succeeding {@link Properties}.
* {@link Properties} can only be added once!
*
* @param aProperties The properties to be prepended.
*
* @return True in case the {@link Properties} have been added, false if
* them have already been added before.
*/
boolean prependProperties( Properties aProperties );
}
// /////////////////////////////////////////////////////////////////////////
// BUILDER:
// /////////////////////////////////////////////////////////////////////////
/**
* The interface {@link MutablePropertiesPrecedence} defines builder
* functionality on top of the properties .
*/
public interface PropertiesPrecedenceBuilder extends MutablePropertiesPrecedence {
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* Builder method for the {@link #appendProperties(Properties)} method.
*
* @param aProperties The properties to be appended.
*
* @return Returns this instance as of the builder pattern for chained
* method calls.
*/
default PropertiesPrecedenceBuilder withAppendProperties( Properties aProperties ) {
appendProperties( aProperties );
return this;
}
/**
* Builder method for the {@link #prependProperties(Properties)} method.
*
* @param aProperties The properties to be prepended.
*
* @return Returns this instance as of the builder pattern for chained
* method calls.
*/
default PropertiesPrecedenceBuilder withPrependProperties( Properties aProperties ) {
prependProperties( aProperties );
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy