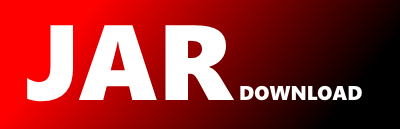
org.refcodes.properties.PropertiesPrecedenceComposite Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.refcodes.exception.IntegrityException;
/**
* Retrieve properties from various different properties sources
* ({@link Properties} instances) by querying all the herein contained
* {@link Properties} instances in the order of them being added. Queried
* properties of the first {@link Properties} instance containing them are
* returned. {@link Properties} instances before have a higher precedence than
* the instances added next.
*/
public class PropertiesPrecedenceComposite implements PropertiesPrecedence {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
protected List _properties;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link PropertiesPrecedence} composite querying the provided
* {@link Properties} in the given order. Queried properties of the first
* {@link Properties} instance containing them are returned.
* {@link Properties} before have a higher precedence over
* {@link Properties} provided next.
*
* @param aProperties The {@link Properties} to be queried in the provided
* order.
*/
public PropertiesPrecedenceComposite( List aProperties ) {
_properties = aProperties;
}
/**
* Creates a {@link PropertiesPrecedence} composite querying the provided
* {@link Properties} in the given order. Queried properties of the first
* {@link Properties} instance containing them are returned.
* {@link Properties} before have a higher precedence over
* {@link Properties} provided next.
*
* @param aProperties The {@link Properties} to be queried in the provided
* order.
*/
public PropertiesPrecedenceComposite( Properties... aProperties ) {
_properties = new ArrayList<>( Arrays.asList( aProperties ) );
}
/**
* {@inheritDoc}
*/
@Override
public boolean containsKey( Object aKey ) {
for ( Properties _propertie : _properties ) {
if ( _propertie.containsKey( aKey ) ) {
return true;
}
}
return false;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean containsProperties( Properties aProperties ) {
return _properties.contains( aProperties );
}
/**
* {@inheritDoc}
*/
@Override
public String get( Object aKey ) {
Properties eProperties;
for ( Properties _propertie : _properties ) {
eProperties = _propertie;
if ( eProperties.containsKey( aKey ) ) {
return eProperties.get( aKey );
}
}
return null;
}
/**
* {@inheritDoc}
*/
@Override
public char getDelimiter() {
Character theDelimiter = null;
char eDelimiter;
for ( Properties _propertie : _properties ) {
eDelimiter = _propertie.getDelimiter();
if ( theDelimiter != null && !theDelimiter.equals( eDelimiter ) ) {
throw new IntegrityException( "The encapsulated properties define different ambiguous delimiter <" + theDelimiter + "> and <" + eDelimiter + "> definitions." );
}
theDelimiter = eDelimiter;
}
return theDelimiter;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isEmpty() {
for ( Properties _propertie : _properties ) {
if ( !_propertie.isEmpty() ) {
return false;
}
}
return true;
}
/**
* {@inheritDoc}
*/
@Override
public Set keySet() {
final Set theSet = new LinkedHashSet<>();
for ( Properties _propertie : _properties ) {
theSet.addAll( _propertie.keySet() );
}
return theSet;
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveFrom( String aFromPath ) {
final PropertiesBuilder theBuilder = new PropertiesBuilderImpl();
for ( int i = _properties.size() - 1; i >= 0; i-- ) {
theBuilder.insert( _properties.get( i ).retrieveFrom( aFromPath ) );
}
return theBuilder;
}
/**
* {@inheritDoc}
*/
@Override
public Properties retrieveTo( String aToPath ) {
final PropertiesBuilder theBuilder = new PropertiesBuilderImpl();
for ( int i = _properties.size() - 1; i >= 0; i-- ) {
theBuilder.insert( _properties.get( i ).retrieveTo( aToPath ) );
}
return theBuilder;
}
/**
* {@inheritDoc}
*/
@Override
public int size() {
int size = 0;
for ( Properties _propertie : _properties ) {
size += _propertie.size();
}
return size;
}
/**
* {@inheritDoc}
*/
@Override
public Object toDataStructure( String aFromPath ) {
final PropertiesBuilder theProperties = new PropertiesBuilderImpl();
for ( int i = _properties.size() - 1; i >= 0; i-- ) {
theProperties.insert( _properties.get( i ).retrieveFrom( aFromPath ) );
}
return theProperties.toDataStructure();
}
/**
* {@inheritDoc}
*/
@Override
public Collection values() {
final Collection theCollection = new ArrayList<>();
for ( Properties _propertie : _properties ) {
theCollection.addAll( _propertie.values() );
}
return theCollection;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy