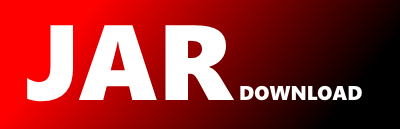
org.refcodes.properties.PropertiesSugar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.text.ParseException;
import org.refcodes.controlflow.ThreadMode;
import org.refcodes.data.Delimiter;
import org.refcodes.data.IoPollLoopTime;
import org.refcodes.properties.Properties.PropertiesBuilder;
import org.refcodes.properties.ResourceProperties.ResourcePropertiesBuilder;
import org.refcodes.properties.ScheduledResourceProperties.ScheduledResourcePropertiesBuilder;
import org.refcodes.runtime.ConfigLocator;
import org.refcodes.struct.Property;
import org.refcodes.struct.PropertyImpl;
/**
* Declarative syntactic sugar which may be statically imported in order to
* allow declarative definitions for the construction of {@link Properties}
* precedences using {@link ProfilePropertiesDecorator},
* {@link ProfilePropertiesProjection}, {@link ResourceProperties} or
* {@link PropertiesPrecedenceComposite} (and the like).
*/
public class PropertiesSugar {
// /////////////////////////////////////////////////////////////////////////
// JAVA PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Files the Java properties to the given {@link File}. For Java properties,
* see "https://en.wikipedia.org/wiki/.properties".
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToJavaProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JavaPropertiesBuilder( aProperties );
theBuilder.fileTo( aFile );
return theBuilder;
}
/**
* Files the Java properties to the given file's path. For Java properties,
* see "https://en.wikipedia.org/wiki/.properties".
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToJavaProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JavaPropertiesBuilder( aProperties );
theBuilder.fileTo( aFilePath );
return theBuilder;
}
/**
* Files the JSON properties to the given {@link File}. For JSON properties,
* see "https://en.wikipedia.org/wiki/JSON"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToJsonProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JsonPropertiesBuilder( aProperties );
theBuilder.fileTo( aFile );
return theBuilder;
}
/**
* Files the JSON properties to the given file's path. For JSON properties,
* see "https://en.wikipedia.org/wiki/JSON"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToJsonProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JsonPropertiesBuilder( aProperties );
theBuilder.fileTo( aFilePath );
return theBuilder;
}
/**
* Files the TOML properties to the given {@link File}. For TOML properties,
* see "https://en.wikipedia.org/wiki/TOML"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToTomlProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new TomlPropertiesBuilder( aProperties );
theBuilder.fileTo( aFile );
return theBuilder;
}
/**
* Files the TOML properties to the given file's path. For TOML properties,
* see "https://en.wikipedia.org/wiki/TOML"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToTomlProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new TomlPropertiesBuilder( aProperties );
theBuilder.fileTo( aFilePath );
return theBuilder;
}
// /////////////////////////////////////////////////////////////////////////
// TOML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Files the XML properties to the given {@link File}. For XML properties,
* see "https://en.wikipedia.org/wiki/XML"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToXmlProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new XmlPropertiesBuilder( aProperties );
theBuilder.fileTo( aFile );
return theBuilder;
}
/**
* Files the XML properties to the given file's path. For XML properties,
* see "https://en.wikipedia.org/wiki/XML"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToXmlProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new XmlPropertiesBuilder( aProperties );
theBuilder.fileTo( aFilePath );
return theBuilder;
}
/**
* Files the YAML properties to the given {@link File}. For YAML properties,
* see "https://en.wikipedia.org/wiki/YAML"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToYamlProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new YamlPropertiesBuilder( aProperties );
theBuilder.fileTo( aFile );
return theBuilder;
}
/**
* Files the YAML properties to the given file's path. For YAML properties,
* see "https://en.wikipedia.org/wiki/YAML"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder fileToYamlProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new YamlPropertiesBuilder( aProperties );
theBuilder.fileTo( aFilePath );
return theBuilder;
}
/**
* Creates a {@link Properties} instance from the provided {@link Property}
* instances. Use {@link #toProperty(String, String)} to compose your
* properties to be added.
*
* @param aProperties The {@link Property} instances' key/value-pairs to be
* added.
*
* @return The according {@link PropertiesBuilder} containing the provided
* {@link Property} instances.
*
* @deprecated Implemented to see how it feels like using it in my sources
*/
@Deprecated
public static PropertiesBuilder from( Property... aProperties ) {
return new PropertiesBuilderImpl( aProperties );
}
/**
* Creates a {@link Property} from the provided key and the provided value.
*
* @param aKey The name of the property.
* @param aValue The value of the property.
*
* @return The {@link Property} representing the key/value-pair.
*
* @deprecated Implemented to see how it feels like using it in my sources
*/
@Deprecated
public static Property from( String aKey, String aValue ) {
return new PropertyImpl( aKey, aValue );
}
// /////////////////////////////////////////////////////////////////////////
// JSON PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Returns {@link Properties} representing the operating system's
* environment variables as of env
on Linux or Unix shells or
* set
on Windows machines.
*
* @return The current JVM's system properties.
*/
public static Properties fromEnvironmentVariables() {
return new EnvironmentProperties();
}
/**
* Wraps the {@link Properties} with a profile projection. The
* {@link Properties} are decorated with a
* {@link ProfilePropertiesProjection} which represents the wrapped
* {@link Properties} with the given profiles applied. See also
* {@link ProfileProperties#toRuntimeProfile(String...)}. The profiles being
* used are found at the given {@link Properties} "runtime/profiles" path as
* defined by {@link PropertiesPath#RUNTIME_PROFILES_PATH}. This path points
* to an array with the Runtime-Profiles denoted as follows, pointing to
* your active Runtime-Profiles:
*
* - runtime/profiles/0=local
* - runtime/profiles/1=oauth
* - runtime/profiles/2=development
*
* In case a value is found at the path "runtime/profiles", this is parsed
* as a comma-separated list and converted to an array. The above
* configuration therewith may also be written as follows:
* runtime/profiles=local,oauth,development
This array beats
* the above kind of declaration so that the active Runtime-Profiles can
* easily be overwritten by {@link ProfileProperties} supporting Java
* System-Properties (passed to the JVM via "-Dx=y") e.g. the
* ApplicationPropertiesComposite
found in the
* refcodes-runtime
artifact.
*
* @param aProperties The properties which are to be projected according to
* the profiles provided by the given {@link Properties}. The
* evaluation is done as of
* {@link ProfileProperties#toRuntimeProfile()}.
*
* @return The {@link Properties} projection as of the profiles provided by
* the given {@link Properties}.
*/
public static Properties fromProfile( Properties aProperties ) {
return new ProfilePropertiesProjection( aProperties );
}
/**
* Wraps the {@link Properties} with a profile projection. The
* {@link Properties} are decorated with a
* {@link ProfilePropertiesProjection} which represents the wrapped
* {@link Properties} with the given profiles applied. See also
* {@link ProfileProperties#toRuntimeProfile(String...)}.
*
* @param aProperties The properties which are to be projected according to
* the provided profiles. The evaluation is done as of
* {@link ProfileProperties#toRuntimeProfile(String...)}.
* @param aProfiles The profiles to be used when constructing the
* projection.
*
* @return The {@link Properties} projection as of the provided profiles.
*/
public static Properties fromProfile( Properties aProperties, String... aProfiles ) {
return new ProfilePropertiesProjection( aProperties, aProfiles );
}
/**
* Creates a {@link Properties} instance from the provided {@link Property}
* instances. Use {@link #toProperty(String, String)} to compose your
* properties to be added.
*
* @param aProperties The {@link Property} instances' key/value-pairs to be
* added.
*
* @return The according {@link PropertiesBuilder} containing the provided
* {@link Property} instances.
*/
public static PropertiesBuilder fromProperties( Property... aProperties ) {
return new PropertiesBuilderImpl( aProperties );
}
/**
* Returns {@link Properties} representing the system properties as passed
* via the "-Dkey=value" when launching the JVM (e.g.java
* -Dconsole.width=220).
*
* @return The current JVM's system properties.
*/
public static Properties fromSystemProperties() {
return new SystemProperties();
}
/**
* Loads the Java properties from the given {@link File}. For Java
* properties, see "https://en.wikipedia.org/wiki/.properties".
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJavaProperties( File aFile ) throws IOException, ParseException {
return new JavaPropertiesBuilder( aFile );
}
// /////////////////////////////////////////////////////////////////////////
// YAML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Reads the properties from the given {@link InputStream}. For Java
* properties, see "https://en.wikipedia.org/wiki/.properties".
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJavaProperties( InputStream aInputStream ) throws IOException, ParseException {
return new JavaPropertiesBuilder( aInputStream );
}
/**
* Loads the Java properties from the given file's path. For Java
* properties, see "https://en.wikipedia.org/wiki/.properties".
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJavaProperties( String aFilePath ) throws IOException, ParseException {
return new JavaPropertiesBuilder( aFilePath );
}
/**
* Loads the Java properties from the given {@link URL}. For Java
* properties, see "https://en.wikipedia.org/wiki/.properties".
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJavaProperties( URL aUrl ) throws IOException, ParseException {
return new JavaPropertiesBuilder( aUrl );
}
/**
* Loads the JSON properties from the given {@link File}. For JSON
* properties, see "https://en.wikipedia.org/wiki/JSON"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJsonProperties( File aFile ) throws IOException, ParseException {
return new JsonPropertiesBuilder( aFile );
}
/**
* Reads the properties from the given {@link InputStream}. For JSON
* properties, see "https://en.wikipedia.org/wiki/JSON"
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJsonProperties( InputStream aInputStream ) throws IOException, ParseException {
return new JsonPropertiesBuilder( aInputStream );
}
/**
* Loads the JSON properties from the given file's path. For JSON
* properties, see "https://en.wikipedia.org/wiki/JSON"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJsonProperties( String aFilePath ) throws IOException, ParseException {
return new JsonPropertiesBuilder( aFilePath );
}
// /////////////////////////////////////////////////////////////////////////
// XML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Loads the JSON properties from the given {@link URL}. For JSON
* properties, see "https://en.wikipedia.org/wiki/JSON"
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromJsonProperties( URL aUrl ) throws IOException, ParseException {
return new JsonPropertiesBuilder( aUrl );
}
/**
* Loads the TOML properties from the given {@link File}. For TOML
* properties, see "https://en.wikipedia.org/wiki/TOML"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromTomlProperties( File aFile ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aFile );
}
/**
* Reads the properties from the given {@link InputStream}. For TOML
* properties, see "https://en.wikipedia.org/wiki/TOML"
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromTomlProperties( InputStream aInputStream ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aInputStream );
}
/**
* Loads the TOML properties from the given file's path. For TOML
* properties, see "https://en.wikipedia.org/wiki/TOML"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromTomlProperties( String aFilePath ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aFilePath );
}
/**
* Loads the TOML properties from the given {@link URL}. For TOML
* properties, see "https://en.wikipedia.org/wiki/TOML"
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromTomlProperties( URL aUrl ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aUrl );
}
/**
* Loads the XML properties from the given {@link File}. For XML properties,
* see "https://en.wikipedia.org/wiki/XML"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromXmlProperties( File aFile ) throws IOException, ParseException {
return new XmlPropertiesBuilder( aFile );
}
// /////////////////////////////////////////////////////////////////////////
// JAVA PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Reads the properties from the given {@link InputStream}. For XML
* properties, see "https://en.wikipedia.org/wiki/XML"
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromXmlProperties( InputStream aInputStream ) throws IOException, ParseException {
return new XmlPropertiesBuilder( aInputStream );
}
/**
* Loads the XML properties from the given file's path. For XML properties,
* see "https://en.wikipedia.org/wiki/XML"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromXmlProperties( String aFilePath ) throws IOException, ParseException {
return new XmlPropertiesBuilder( aFilePath );
}
/**
* Loads the XML properties from the given {@link URL}. For XML properties,
* see "https://en.wikipedia.org/wiki/XML"
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromXmlProperties( URL aUrl ) throws IOException, ParseException {
return new XmlPropertiesBuilder( aUrl );
}
// /////////////////////////////////////////////////////////////////////////
// TOML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Loads the YAML properties from the given {@link File}. For YAML
* properties, see "https://en.wikipedia.org/wiki/YAML"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromYamlProperties( File aFile ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aFile );
}
/**
* Reads the properties from the given {@link InputStream}. For YAML
* properties, see "https://en.wikipedia.org/wiki/YAML"
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromYamlProperties( InputStream aInputStream ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aInputStream );
}
/**
* Loads the YAML properties from the given file's path. For YAML
* properties, see "https://en.wikipedia.org/wiki/YAML"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromYamlProperties( String aFilePath ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aFilePath );
}
// /////////////////////////////////////////////////////////////////////////
// JSON PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Loads the YAML properties from the given {@link URL}. For YAML
* properties, see "https://en.wikipedia.org/wiki/YAML"
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder loadFromYamlProperties( URL aUrl ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aUrl );
}
/**
* Saves the Java properties to the given {@link File}. For Java properties,
* see "https://en.wikipedia.org/wiki/.properties".
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToJavaProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JavaPropertiesBuilder( aProperties );
theBuilder.saveTo( aFile );
return theBuilder;
}
/**
* Writes the properties to the given {@link OutputStream}. For Java
* properties, see "https://en.wikipedia.org/wiki/.properties".
*
* @param aProperties The {@link Properties} to save.
* @param aOutputStream The {@link OutputStream} to which to write the
* properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToJavaProperties( Properties aProperties, OutputStream aOutputStream ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JavaPropertiesBuilder( aProperties );
theBuilder.saveTo( aOutputStream );
return theBuilder;
}
// /////////////////////////////////////////////////////////////////////////
// YAML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Saves the Java properties to the given file's path. For Java properties,
* see "https://en.wikipedia.org/wiki/.properties".
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToJavaProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JavaPropertiesBuilder( aProperties );
theBuilder.saveTo( aFilePath );
return theBuilder;
}
/**
* Saves the JSON properties to the given {@link File}. For JSON properties,
* see "https://en.wikipedia.org/wiki/JSON"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToJsonProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JsonPropertiesBuilder( aProperties );
theBuilder.saveTo( aFile );
return theBuilder;
}
/**
* Writes the properties to the given {@link OutputStream}. For JSON
* properties, see "https://en.wikipedia.org/wiki/JSON"
*
* @param aProperties The {@link Properties} to save.
* @param aOutputStream The {@link OutputStream} to which to write the
* properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToJsonProperties( Properties aProperties, OutputStream aOutputStream ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JsonPropertiesBuilder( aProperties );
theBuilder.saveTo( aOutputStream );
return theBuilder;
}
// /////////////////////////////////////////////////////////////////////////
// XML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Saves the JSON properties to the given file's path. For JSON properties,
* see "https://en.wikipedia.org/wiki/JSON"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToJsonProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new JsonPropertiesBuilder( aProperties );
theBuilder.saveTo( aFilePath );
return theBuilder;
}
/**
* Saves the TOML properties to the given {@link File}. For TOML properties,
* see "https://en.wikipedia.org/wiki/TOML"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToTomlProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new TomlPropertiesBuilder( aProperties );
theBuilder.saveTo( aFile );
return theBuilder;
}
/**
* Writes the properties to the given {@link OutputStream}. For TOML
* properties, see "https://en.wikipedia.org/wiki/TOML"
*
* @param aProperties The {@link Properties} to save.
* @param aOutputStream The {@link OutputStream} to which to write the
* properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToTomlProperties( Properties aProperties, OutputStream aOutputStream ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new TomlPropertiesBuilder( aProperties );
theBuilder.saveTo( aOutputStream );
return theBuilder;
}
// /////////////////////////////////////////////////////////////////////////
// JAVA PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Saves the TOML properties to the given file's path. For TOML properties,
* see "https://en.wikipedia.org/wiki/TOML"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToTomlProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new TomlPropertiesBuilder( aProperties );
theBuilder.saveTo( aFilePath );
return theBuilder;
}
/**
* Saves the XML properties to the given {@link File}. For XML properties,
* see "https://en.wikipedia.org/wiki/XML"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToXmlProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new XmlPropertiesBuilder( aProperties );
theBuilder.saveTo( aFile );
return theBuilder;
}
// /////////////////////////////////////////////////////////////////////////
// TOML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Writes the properties to the given {@link OutputStream}. For XML
* properties, see "https://en.wikipedia.org/wiki/XML"
*
* @param aProperties The {@link Properties} to save.
* @param aOutputStream The {@link OutputStream} to which to write the
* properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToXmlProperties( Properties aProperties, OutputStream aOutputStream ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new XmlPropertiesBuilder( aProperties );
theBuilder.saveTo( aOutputStream );
return theBuilder;
}
/**
* Saves the XML properties to the given file's path. For XML properties,
* see "https://en.wikipedia.org/wiki/XML"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToXmlProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new XmlPropertiesBuilder( aProperties );
theBuilder.saveTo( aFilePath );
return theBuilder;
}
// /////////////////////////////////////////////////////////////////////////
// JSON PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Saves the YAML properties to the given {@link File}. For YAML properties,
* see "https://en.wikipedia.org/wiki/YAML"
*
* @param aProperties The {@link Properties} to save.
* @param aFile The {@link File} to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToYamlProperties( Properties aProperties, File aFile ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new YamlPropertiesBuilder( aProperties );
theBuilder.saveTo( aFile );
return theBuilder;
}
/**
* Writes the properties to the given {@link OutputStream}. For YAML
* properties, see "https://en.wikipedia.org/wiki/YAML"
*
* @param aProperties The {@link Properties} to save.
* @param aOutputStream The {@link OutputStream} to which to write the
* properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToYamlProperties( Properties aProperties, OutputStream aOutputStream ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new YamlPropertiesBuilder( aProperties );
theBuilder.saveTo( aOutputStream );
return theBuilder;
}
// /////////////////////////////////////////////////////////////////////////
// YAML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Saves the YAML properties to the given file's path. For YAML properties,
* see "https://en.wikipedia.org/wiki/YAML"
*
* @param aProperties The {@link Properties} to save.
* @param aFilePath The path to the file to which to save the properties.
*
* @return The according {@link ResourceProperties} instance.
*
* @throws IOException thrown in case writing or processing the properties
* file failed.
*/
public static ResourcePropertiesBuilder saveToYamlProperties( Properties aProperties, String aFilePath ) throws IOException {
final ResourcePropertiesBuilder theBuilder = new YamlPropertiesBuilder( aProperties );
theBuilder.saveTo( aFilePath );
return theBuilder;
}
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with default settings being a poll loop time
* of 10 seconds (as of {@link IoPollLoopTime#NORM} and orphan removal (as
* of {@link ResourceProperties#reload(ReloadMode)} being called with
* {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts polling after
* construction of this instance for new properties. The scheduling
* {@link Thread} is started as daemon thread (see
* {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties );
}
// /////////////////////////////////////////////////////////////////////////
// XML PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with setting the given poll loop time and
* orphan removal (as of {@link ResourceProperties#reload(ReloadMode)} being
* called with {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts
* polling after construction of this instance for new properties. The
* scheduling {@link Thread} is started as daemon thread (see
* {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties, int aScheduleTimeMillis ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties, aScheduleTimeMillis );
}
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with setting the given poll loop time and the
* given orphan removal strategy (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with your
* argument). Immediately starts polling after construction of this instance
* for new properties. The scheduling {@link Thread} is started as daemon
* thread (see {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties, int aScheduleTimeMillis, ReloadMode aReloadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties, aScheduleTimeMillis, aReloadMode );
}
// /////////////////////////////////////////////////////////////////////////
// PROPERTIES PRECEDENCE:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with setting the given poll loop time and the
* given orphan removal strategy (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with your
* argument). Immediately starts polling after construction of this instance
* for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties, int aScheduleTimeMillis, ReloadMode aReloadMode, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties, aScheduleTimeMillis, aReloadMode, aThreadMode );
}
// /////////////////////////////////////////////////////////////////////////
// NORMALIZED PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with setting the given poll loop time and
* orphan removal (as of {@link ResourceProperties#reload(ReloadMode)} being
* called with {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts
* polling after construction of this instance for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties, int aScheduleTimeMillis, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties, aScheduleTimeMillis, aThreadMode );
}
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with setting the the default poll loop time of
* 10 seconds (as of {@link IoPollLoopTime#NORM} and the given orphan
* removal strategy (as of {@link ResourceProperties#reload(ReloadMode)}
* being called with your argument). Immediately starts polling after
* construction of this instance for new properties. The scheduling
* {@link Thread} is started as daemon thread (see
* {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties, ReloadMode aReloadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties, aReloadMode );
}
// /////////////////////////////////////////////////////////////////////////
// BUILDER
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with setting the the default poll loop time of
* 10 seconds (as of {@link IoPollLoopTime#NORM} and the given orphan
* removal strategy (as of {@link ResourceProperties#reload(ReloadMode)}
* being called with your argument). Immediately starts polling after
* construction of this instance for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties, ReloadMode aReloadMode, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties, aReloadMode, aThreadMode );
}
/**
* Creates a {@link ScheduledResourcePropertiesDecorator} wrapping the given
* {@link ResourceProperties} with default settings being a poll loop time
* of 10 seconds (as of {@link IoPollLoopTime#NORM} and orphan removal (as
* of {@link ResourceProperties#reload(ReloadMode)} being called with
* {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts polling after
* construction of this instance for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourceProperties} encapsulating the
* provided {@link ResourceProperties}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourceProperties schedule( ResourceProperties aProperties, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesDecorator( aProperties, aThreadMode );
}
// /////////////////////////////////////////////////////////////////////////
// PROPERTY:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with default settings being a
* poll loop time of 10 seconds (as of {@link IoPollLoopTime#NORM}) and
* orphan removal (as of {@link ResourceProperties#reload(ReloadMode)} being
* called with {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts
* polling after construction of this instance for new properties. The
* scheduling {@link Thread} is started as daemon thread (see
* {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties );
}
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with setting the given poll
* loop time and orphan removal (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with
* {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts polling after
* construction of this instance for new properties. The scheduling
* {@link Thread} is started as daemon thread (see
* {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties, int aScheduleTimeMillis ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties, aScheduleTimeMillis );
}
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with setting the given poll
* loop time and the given orphan removal strategy (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with your
* argument). Immediately starts polling after construction of this instance
* for new properties. The scheduling {@link Thread} is started as daemon
* thread (see {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties, int aScheduleTimeMillis, ReloadMode aReloadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties, aScheduleTimeMillis, aReloadMode );
}
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with setting the given poll
* loop time and the given orphan removal strategy (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with your
* argument). Immediately starts polling after construction of this instance
* for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties, int aScheduleTimeMillis, ReloadMode aReloadMode, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties, aScheduleTimeMillis, aReloadMode, aThreadMode );
}
// /////////////////////////////////////////////////////////////////////////
// SYSTEM PROPERTIES:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with setting the given poll
* loop time and orphan removal (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with
* {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts polling after
* construction of this instance for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aScheduleTimeMillis The time in milliseconds between polling for
* new properties.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties, int aScheduleTimeMillis, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties, aScheduleTimeMillis, aThreadMode );
}
// /////////////////////////////////////////////////////////////////////////
// ENVIRONMENT VARIABLES:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with setting the the default
* poll loop time of 10 seconds (as of {@link IoPollLoopTime#NORM}) and the
* given orphan removal strategy (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with your
* argument). Immediately starts polling after construction of this instance
* for new properties. The scheduling {@link Thread} is started as daemon
* thread (see {@link ThreadMode#DAEMON}).
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties, ReloadMode aReloadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties, aReloadMode );
}
// /////////////////////////////////////////////////////////////////////////
// PROFILE:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with setting the the default
* poll loop time of 10 seconds (as of {@link IoPollLoopTime#NORM}) and the
* given orphan removal strategy (as of
* {@link ResourceProperties#reload(ReloadMode)} being called with your
* argument). Immediately starts polling after construction of this instance
* for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties, ReloadMode aReloadMode, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties, aReloadMode, aThreadMode );
}
/**
* Creates a {@link ScheduledResourcePropertiesBuilderDecorator} wrapping
* the given {@link ResourcePropertiesBuilder} with default settings being a
* poll loop time of 10 seconds (as of {@link IoPollLoopTime#NORM}) and
* orphan removal (as of {@link ResourceProperties#reload(ReloadMode)} being
* called with {@link ReloadMode#ORPHAN_REMOVAL}). Immediately starts
* polling after construction of this instance for new properties.
*
* @param aProperties The properties from which the properties are to be
* reloaded periodically.
* @param aThreadMode The {@link ThreadMode} mode of operation regarding the
* {@link Thread} doing the scheduling job.
* @return the {@link ScheduledResourcePropertiesBuilder} encapsulating the
* provided {@link ResourcePropertiesBuilder}.
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
public static ScheduledResourcePropertiesBuilder schedule( ResourcePropertiesBuilder aProperties, ThreadMode aThreadMode ) throws IOException, ParseException {
return new ScheduledResourcePropertiesBuilderDecorator( aProperties, aThreadMode );
}
// /////////////////////////////////////////////////////////////////////////
// SCHEDULE:
// /////////////////////////////////////////////////////////////////////////
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For Java properties, see
* "https://en.wikipedia.org/wiki/.properties".
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromJavaProperties( File aFile ) throws IOException, ParseException {
return new JavaPropertiesBuilder( aFile, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For Java properties, see
* "https://en.wikipedia.org/wiki/.properties".
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromJavaProperties( String aFilePath ) throws IOException, ParseException {
return new JavaPropertiesBuilder( aFilePath, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For JSON properties, see
* "https://en.wikipedia.org/wiki/JSON"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromJsonProperties( File aFile ) throws IOException, ParseException {
return new JsonPropertiesBuilder( aFile, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For JSON properties, see
* "https://en.wikipedia.org/wiki/JSON"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromJsonProperties( String aFilePath ) throws IOException, ParseException {
return new JsonPropertiesBuilder( aFilePath, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For TOML properties, see
* "https://en.wikipedia.org/wiki/TOML"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromTomlProperties( File aFile ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aFile, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For TOML properties, see
* "https://en.wikipedia.org/wiki/TOML"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromTomlProperties( String aFilePath ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aFilePath, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For XML properties, see
* "https://en.wikipedia.org/wiki/XML"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromXmlProperties( File aFile ) throws IOException, ParseException {
return new XmlPropertiesBuilder( aFile, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For XML properties, see
* "https://en.wikipedia.org/wiki/XML"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromXmlProperties( String aFilePath ) throws IOException, ParseException {
return new XmlPropertiesBuilder( aFilePath, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For YAML properties, see
* "https://en.wikipedia.org/wiki/YAML"
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromYamlProperties( File aFile ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aFile, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification of the
* {@link ConfigLocator#ALL} configuration. For YAML properties, see
* "https://en.wikipedia.org/wiki/YAML"
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The according {@link Properties} instance.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public static ResourcePropertiesBuilder seekFromYamlProperties( String aFilePath ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aFilePath, ConfigLocator.ALL );
}
/**
* Decorates the provided {@link Properties} with additional behavior by
* normalizing the key representing the path pointing to the according
* value. You provide {@link Properties} with a path delimiter different
* from the default delimiter and you get {@link Properties} which act as if
* the delimiter was the default delimiter: Paths containing the namespace
* delimiter "." (as of {@link Delimiter#NAMESPACE} are converted to paths'
* with delimiter "/" (as of {@link Delimiter#PATH}. Changes applied to the
* provided {@link Properties} affect the decorator.
*
* @param aProperties The {@link Properties} to be decorated.
*
* @return A {@link Properties} decorator normalizing the the provided
* {@link Properties}' paths.
*/
public static Properties toNormalized( Properties aProperties ) {
return new NormalizedPropertiesDecorator( aProperties );
}
/**
* Decorates the provided {@link Properties} with additional behavior by
* normalizing the key representing the path pointing to the according
* value. You provide {@link Properties} with a path delimiter different
* from the default delimiter and you get {@link Properties} which act as if
* the delimiter was the default delimiter: Paths containing the provided
* delimiters are converted to the paths' with delimiter "/" (as of
* {@link Delimiter#PATH}. Changes applied to the provided
* {@link Properties} affect the decorator.
*
* @param aProperties The {@link Properties} to be decorated.
* @param aDelimiters The delimiters to be converted forth and back
*
* @return A {@link Properties} decorator normalizing the the provided
* {@link Properties}' paths.
*/
public static Properties toNormalized( Properties aProperties, char[] aDelimiters ) {
return new NormalizedPropertiesDecorator( aProperties, aDelimiters );
}
/**
* Creates a {@link Properties} composite querying the provided
* {@link Properties} in the given order. Queried properties of the first
* {@link Properties} instance containing them are returned.
* {@link Properties} before have a higher precedence over
* {@link Properties} provided next.
*
* @param aProperties The {@link Properties} to be queried in the provided
* order.
*
* @return A {@link Properties} composite querying the provided properties
* in the given order.
*/
public static Properties toPrecedence( Properties... aProperties ) {
return new PropertiesPrecedenceComposite( aProperties );
}
/**
* Creates an empty {@link PropertiesBuilder}.
*
* @return The newly created empty {@link PropertiesBuilder}.
*/
public static PropertiesBuilder toPropertiesBuilder() {
return new PropertiesBuilderImpl();
}
/**
* Creates a new {@link PropertiesBuilder} from the {@link Properties}.
* Succeeding provided {@link Properties} overrule provided preceding
* {@link Properties}.
*
* @param aProperties The {@link Properties} to be added to the resulting
* {@link PropertiesBuilder}. The later the {@link Properties} in the
* varargs chain, the more do they overrule the earlier
* {@link Properties} from the varargs chain.
*
* @return The {@link PropertiesBuilder} containing the provided properties.
*/
public static PropertiesBuilder toPropertiesBuilder( Properties... aProperties ) {
final PropertiesBuilder theBuilder = new PropertiesBuilderImpl();
for ( Properties eProperties : aProperties ) {
theBuilder.insert( eProperties );
}
return theBuilder;
}
/**
* Creates a {@link Property} from the provided key and the provided value.
*
* @param aKey The name of the property.
* @param aValue The value of the property.
*
* @return he {@link Property} representing the key/value-pair.
*/
public static Property toProperty( String aKey, String aValue ) {
return new PropertyImpl( aKey, aValue );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy