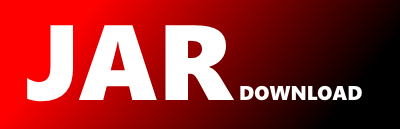
org.refcodes.properties.ResourceLoaderBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.text.ParseException;
import org.refcodes.runtime.ConfigLocator;
import org.refcodes.runtime.Execution;
/**
* This interface provides builder additions (as of the builder pattern for
* chained configuring method calls) for "dynamic" {@link ResourceProperties}:
* As {@link ResourceProperties} are immutable from an interface's point of
* view, there are no mutating methods provided. Sub-types of the
* {@link ResourceProperties} might need to load the properties after
* instantiation. Such types might implement this interface, providing means to
* load from resources after instantiation.
*
* @param the generic type
*/
public interface ResourceLoaderBuilder> {
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* method {@link ConfigLocator#getFolders()}.
*
* @param aFile The file of the properties to load.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default B withFile( File aFile ) throws IOException, ParseException {
return withFile( aFile, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* method {@link ConfigLocator#getFolders()}.
*
* @param aFile The file of the properties to load.
* @param aConfigLocator The {@link ConfigLocator} specifying where to seek
* for properties.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
B withFile( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException;
/**
* Reads the properties from the given {@link InputStream}.
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
B withInputStream( InputStream aInputStream ) throws IOException, ParseException;
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* method {@link ConfigLocator#getFolders()}.
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file of the properties file to load.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default B withResourceClass( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
return withResourceClass( aResourceClass, aFilePath, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* method {@link ConfigLocator#getFolders()}.
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file of the properties file to load.
* @param aConfigLocator The {@link ConfigLocator} specifying where to seek
* for properties.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
B withResourceClass( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException;
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* method {@link ConfigLocator#getFolders()}.
*
* @param aFilePath The file of the properties file to load.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default B withResourceClass( String aFilePath ) throws IOException, ParseException {
return withResourceClass( Execution.getMainClass(), aFilePath, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* method {@link ConfigLocator#getFolders()}.
*
* @param aFilePath The file of the properties file to load.
* @param aConfigLocator The {@link ConfigLocator} specifying where to seek
* for properties.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default B withResourceClass( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return withResourceClass( null, aFilePath, aConfigLocator );
}
/**
* Loads the properties from the given {@link URL}.
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @return This instance as of the builder pattern for chained method calls.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
B withUrl( URL aUrl ) throws IOException, ParseException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy