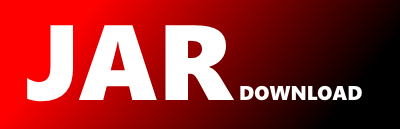
org.refcodes.properties.ResourceProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.text.ParseException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.refcodes.component.Flushable;
import org.refcodes.component.Flushable.FlushBuilder;
import org.refcodes.data.Text;
import org.refcodes.exception.BugException;
import org.refcodes.runtime.ConfigLocator;
import org.refcodes.struct.PathMap;
import org.refcodes.struct.Property;
import org.refcodes.struct.Relation;
import org.refcodes.struct.Table;
/**
* The {@link ResourceProperties} are a https://www.metacodes.proization of the
* {@link Table}. Properties represent a collection of {@link String} key and
* {@link String} value pairs as properties usually occur in pure text form, to
* be converted to the required data types. For this reason, the
* {@link ResourceProperties} interface provides additional conversion methods.
* When parsing properties from notations which do not support nodes to have
* values as well as child elements, then please support one of the identifiers
* contained in the THIS_ATTRIBUTES
array.
*/
public interface ResourceProperties extends Properties {
/**
* Reloads the {@link ResourceProperties} from the resource to which the
* {@link ResourceProperties} are attached to (such as a {@link File} as of
* {@link ResourcePropertiesBuilder#loadFrom(File)} or
* {@link ResourcePropertiesBuilder#saveTo(File)}). In case the resource
* (such as an {@link InputStream}) does not support reloading, then an
* {@link IllegalStateException} is thrown. Properties existing in the
* attached resource as well in the {@link Properties} itself are replaced.
* Properties existing in the attached resource but not(!) in the
* {@link Properties} itself are not(!) removed. Use
* {@link #reload(ReloadMode)} with an argument of true
(~
* orphan removal) to remove properties not existing in the resource.
*
* @return The {@link Properties} as loaded from the resource and applied to
* this instance.
*
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
Properties reload() throws IOException, ParseException;
/**
* Reloads the {@link ResourceProperties} from the resource to which the
* {@link ResourceProperties} are attached to (such as a {@link File} as of
* {@link ResourcePropertiesBuilder#loadFrom(File)} or
* {@link ResourcePropertiesBuilder#saveTo(File)}). In case the resource
* (such as an {@link InputStream}) does not support reloading, then an
* {@link IllegalStateException} is thrown. Properties existing in the
* attached resource as well in the {@link Properties} itself are replaced.
* When "orphan removal" is set to false
, then properties
* existing in the attached resource but not(!) in the {@link Properties}
* itself are not(!) removed. When "orphan removal" is set to
* true
, then properties existing in the attached resource but
* not(!) in the {@link Properties} itself are(!) removed.
*
* @param aReloadMode when set to {@link ReloadMode#ORPHAN_REMOVAL}, then
* properties existing in the attached resource but not(!) in the
* {@link Properties} itself are(!) removed. Else properties not
* existing in the attached resource are kept.
*
* @return The {@link Properties} as loaded from the resource and applied to
* this instance.
*
* @throws IOException thrown in case accessing the resource encountered an
* I/O problem.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
* @throws IllegalStateException in case the attached resource does not
* support reloading.
*/
Properties reload( ReloadMode aReloadMode ) throws IOException, ParseException;
/**
* Produces the external representation of the properties as of the
* serialized format supported by the implementing class.
*
* @return The external (serialized) representation of the properties.
*/
default String toSerialized() {
return toSerialized( getDelimiter() );
}
/**
* Produces the external representation of the properties as of the
* serialized format supported by the implementing class.
*
* @param aDelimiter The path delimiter to be used when serializing the
* properties (in case the the serialized format supports / requires
* the such).
*
* @return The external (serialized) representation of the properties.
*/
default String toSerialized( char aDelimiter ) {
return toSerialized( DEFAULT_COMMENT, aDelimiter );
}
/**
* Produces the external representation of the properties as of the
* serialized format supported by the implementing class.
*
* @param aComment The comment to be used.
*
* @return The external (serialized) representation of the properties.
*/
default String toSerialized( String aComment ) {
return toSerialized( aComment );
}
/**
* Produces the external representation of the properties as of the
* serialized format supported by the implementing class.
*
* @param aComment The comment to be used.
* @param aDelimiter The path delimiter to be used when serializing the
* properties (in case the the serialized format supports / requires
* the such).
*
* @return The external (serialized) representation of the properties.
*/
String toSerialized( String aComment, char aDelimiter );
// /////////////////////////////////////////////////////////////////////////
// MUTATOR:
// /////////////////////////////////////////////////////////////////////////
/**
* The interface {@link MutableResoureProperties} defines "dirty" methods
* allowing to modify ("mutate") the {@link ResourceProperties}.
*/
public interface MutableResoureProperties extends ResourceProperties, MutableProperties, Flushable {
String DEFAULT_COMMENT = "Generated by <" + Text.REFCODES_ORG + "> (http://www.refcodes.org)";
/**
* Files (writes) the properties to the given {@link File}. The
* properties are filed to the first folder detected by the method
* {@link ConfigLocator#getFolders(File...)}. Load them via
* {@link ResourcePropertiesBuilder#seekFrom(File)}. The default
* implementation uses the hook method
* {@link #fileTo(File, String, char)} to finally format and write the
* properties.
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( File aFile ) throws IOException {
return fileTo( aFile, DEFAULT_COMMENT );
}
/**
* Files (writes) the properties to the given {@link File} using the
* provided delimiter as the destination's path delimiter. The
* properties are filed to the first folder detected by the method
* {@link ConfigLocator#getFolders(File...)}. Load them via
* {@link ResourcePropertiesBuilder#seekFrom(File)}. The default
* implementation uses the hook method
* {@link #fileTo(File, String, char)} to finally format and write the
* properties.
*
* @param aFile The {@link File} from which to load the properties.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( File aFile, char aDelimiter ) throws IOException {
return fileTo( aFile, DEFAULT_COMMENT, aDelimiter );
}
/**
* Files (writes) the properties to the given {@link File}. The
* properties are filed to the first folder detected by the method
* {@link ConfigLocator#getFolders(File...)}. Load them via
* {@link ResourcePropertiesBuilder#seekFrom(File)}. The default
* implementation uses the hook method
* {@link #fileTo(File, String, char)} to finally format and write the
* properties.
*
* @param aFile The {@link File} from which to load the properties.
* @param aComment The description for the properties file.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( File aFile, String aComment ) throws IOException {
return fileTo( aFile, aComment, getDelimiter() );
}
/**
* Files (writes) the properties to the given {@link File} using the
* provided delimiter as the destination's path delimiter. The
* properties are filed to the first folder detected by the method
* {@link ConfigLocator#getFolders(File...)}. Load them via
* {@link ResourcePropertiesBuilder#seekFrom(File)}.
*
* @param aFile The {@link File} to which to file the properties to.
* @param aComment The description for the properties file.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( File aFile, String aComment, char aDelimiter ) throws IOException {
final File[] theBaseDirs;
theBaseDirs = ConfigLocator.ALL.getFolders( aFile );
if ( theBaseDirs == null || theBaseDirs.length == 0 ) {
throw new IOException( "Unable to file the properties to <" + aFile.toString() + "> as no approbriate folder could be detected!" );
}
final File theFile = new File( theBaseDirs[0], aFile.getName() );
return saveTo( theFile, aComment, aDelimiter );
}
/**
* Files (writes) the properties to the given {@link File}. The
* properties are filed to the first folder detected by the method
* {@link ConfigLocator#getFolders(File...)}. Load them via
* {@link ResourcePropertiesBuilder#seekFrom(File)} The default
* implementation uses the hook method
* {@link #fileTo(String, String, char)} to finally format and write the
* properties.
*
* @param aFilePath The file path from which to load the properties.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( String aFilePath ) throws IOException {
return fileTo( new File( aFilePath ) );
}
/**
* Files (writes) the properties to the given {@link File} using the
* provided delimiter as the destination's path delimiter. The
* properties are filed to the first folder detected by the method
* {@link ConfigLocator#getFolders(File...)}. Load them via
* {@link ResourcePropertiesBuilder#seekFrom(String)} The default
* implementation uses the hook method
* {@link #fileTo(File, String, char)} to finally format and write the
* properties.
*
* @param aFilePath The file path from which to load the properties.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( String aFilePath, char aDelimiter ) throws IOException {
return fileTo( new File( aFilePath ), aDelimiter );
}
/**
* Files (writes) the properties to the {@link File} represented by the
* given file path. The properties are filed to the first folder
* detected by the method {@link ConfigLocator#getFolders(File...)}.
* Load them via {@link ResourcePropertiesBuilder#seekFrom(String)}. The
* default implementation uses the hook method
* {@link #fileTo(File, String, char)} to finally format and write the
* properties.
*
* @param aFilePath The file path from which to load the properties.
* @param aComment The description for the properties file.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( String aFilePath, String aComment ) throws IOException {
return fileTo( new File( aFilePath ), aComment );
}
/**
* Files (writes) the properties to the {@link File} represented by the
* given file path using the provided delimiter as the destination's
* path delimiter. The properties are filed to the first folder detected
* by the method {@link ConfigLocator#getFolders(File...)}. Load them
* via {@link ResourcePropertiesBuilder#seekFrom(File)}. The default
* implementation uses the hook method
* {@link #fileTo(File, String, char)} to finally format and write the
* properties.
*
* @param aFilePath The file path from which to load the properties.
* @param aComment The description for the properties file.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File fileTo( String aFilePath, String aComment, char aDelimiter ) throws IOException {
return fileTo( new File( aFilePath ), aComment, aDelimiter );
}
/**
* Flushes {@link MutableResoureProperties} to the resource to which the
* {@link ResourceProperties} are attached to (such as a {@link File} as
* of {@link ResourcePropertiesBuilder#loadFrom(File)} or
* {@link #saveTo(File)}). {@inheritDoc}
*/
@Override
void flush() throws IOException;
/**
* Loads the properties from the given class resource (from inside a
* JAR). The default implementation uses the hook method
* {@link #loadFrom(InputStream)} to finally load and parse the
* properties.
*
* @param aResourceClass The class which's class loader is to take care
* of loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case loading the properties failed
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default Properties loadFrom( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
return loadFrom( aResourceClass.getResourceAsStream( aFilePath ) );
}
/**
* Loads the properties from the given {@link File}. Save them using the
* method {@link #saveTo(File)}. The default implementation uses the
* hook method {@link #loadFrom(InputStream)} to finally load and parse
* the properties.
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case loading the properties failed
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
Properties loadFrom( File aFile ) throws IOException, ParseException;
/**
* Loads the properties from the given {@link InputStream}. Save them
* using the method {@link #saveTo(OutputStream)}. The default
* implementation uses the hook method {@link #loadFrom(InputStream)} to
* finally load and parse the properties.
*
* @param aInputStream The {@link InputStream} from which to load the
* properties.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case loading the properties failed
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
Properties loadFrom( InputStream aInputStream ) throws IOException, ParseException;
/**
* Loads the properties from the given file path's {@link File}. Save
* them using the method {@link #saveTo(String)}. The default
* implementation uses the hook method {@link #loadFrom(InputStream)} to
* finally load and parse the properties.
*
* @param aFilePath The file path from which to load the properties.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case loading the properties failed
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded..
*/
default Properties loadFrom( String aFilePath ) throws IOException, ParseException {
return loadFrom( new File( aFilePath ) );
}
/**
* Loads the properties from the given {@link URL}. The default
* implementation uses the hook method {@link #loadFrom(InputStream)} to
* finally load and parse the properties.
*
* @param aUrl The {@link URL} from which to load the properties.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case loading the properties failed
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default Properties loadFrom( URL aUrl ) throws IOException, ParseException {
return loadFrom( aUrl.openStream() );
}
/**
* Inspects the given serialized representation and adds all declared
* elements found. Unmarshaled elements of type {@link Map},
* {@link Collection} and arrays are identified and handled as of their
* type: The path for each value in a {@link Map} is appended with its
* according key. The path for each value in a {@link Collection} or
* array is appended with its according index of occurrence (in case of
* a {@link List} or an array, its actual index). The default
* implementation uses the hook method {@link #loadFrom(InputStream)} to
* finally load and parse the properties.
*
* @param aSerialized The serialized representation which is to be
* parsed for the therein declared elements being added with
* their according determined paths.
*
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default void parseFrom( String aSerialized ) throws ParseException {
final ByteArrayInputStream theInputStream = new ByteArrayInputStream( aSerialized.getBytes() );
try {
loadFrom( theInputStream );
}
catch ( IOException e ) {
throw new BugException( "Encountered bug while creating a stream without any I/O merely in memory!", e );
}
}
/**
* Saves the properties to the given {@link File}. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(File)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File saveTo( File aFile ) throws IOException {
return saveTo( aFile, null );
}
/**
* Saves the properties to the given {@link File} using the provided
* delimiter as the destination's path delimiter. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(File)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFile The {@link File} from which to load the properties.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File saveTo( File aFile, char aDelimiter ) throws IOException {
return saveTo( aFile, null, aDelimiter );
}
/**
* Saves the properties to the given {@link File}. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(File)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFile The {@link File} from which to load the properties.
* @param aComment The description for the properties file.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File saveTo( File aFile, String aComment ) throws IOException {
return saveTo( aFile, aComment, getDelimiter() );
}
/**
* Saves the properties to the given {@link File} using the provided
* delimiter as the destination's path delimiter. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(File)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFile The {@link File} from which to load the properties.
* @param aComment The description for the properties file.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
File saveTo( File aFile, String aComment, char aDelimiter ) throws IOException;
/**
* Saves the properties to the given {@link OutputStream}. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(InputStream)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aOutputStream the output stream
*
* @throws IOException thrown in case saving the properties failed
*/
default void saveTo( OutputStream aOutputStream ) throws IOException {
saveTo( aOutputStream, DEFAULT_COMMENT );
}
/**
* Saves the properties to the given {@link OutputStream}. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(InputStream)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aOutputStream the {@link OutputStream} where to write the
* properties to.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @throws IOException thrown in case saving the properties failed
*/
default void saveTo( OutputStream aOutputStream, char aDelimiter ) throws IOException {
saveTo( aOutputStream, DEFAULT_COMMENT, aDelimiter );
}
/**
* Saves the properties to the given {@link OutputStream}. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(InputStream)} The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aOutputStream The {@link OutputStream} to which to save the
* properties to.
* @param aComment The description for the properties file.
*
* @throws IOException thrown in case saving the properties failed
*/
default void saveTo( OutputStream aOutputStream, String aComment ) throws IOException {
saveTo( aOutputStream, aComment, getDelimiter() );
}
/**
* Saves the properties to the given {@link OutputStream} using the
* provided delimiter as the destination's path delimiter. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(InputStream)} This is the
* hook-method of the default implementation for writing (saving) the
* properties. In case you want to implement {@link ResourceProperties}
* which support other notations than the properties notation
* (path=value
), then you overwrite this method in your
* implementation accordingly.
*
* @param aOutputStream The {@link OutputStream} to which to save the
* properties to.
* @param aComment The description for the properties file.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @throws IOException thrown in case saving the properties failed
*/
void saveTo( OutputStream aOutputStream, String aComment, char aDelimiter ) throws IOException;
/**
* Saves the properties to the given {@link File}. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(File)} The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFilePath The file path from which to load the properties.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File saveTo( String aFilePath ) throws IOException {
return saveTo( new File( aFilePath ) );
}
/**
* Saves the properties to the given {@link File} using the provided
* delimiter as the destination's path delimiter. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(String)} The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFilePath The file path from which to load the properties.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File saveTo( String aFilePath, char aDelimiter ) throws IOException {
return saveTo( new File( aFilePath ), aDelimiter );
}
/**
* Saves the properties to the {@link File} represented by the given
* file path. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(String)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFilePath The file path from which to load the properties.
* @param aComment The description for the properties file.
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File saveTo( String aFilePath, String aComment ) throws IOException {
return saveTo( new File( aFilePath ), aComment );
}
/**
* Saves the properties to the {@link File} represented by the given
* file path using the provided delimiter as the destination's path
* delimiter. Load them via
* {@link ResourcePropertiesBuilder#loadFrom(File)}. The default
* implementation uses the hook method
* {@link #saveTo(OutputStream, String, char)} to finally format and
* write the properties.
*
* @param aFilePath The file path from which to load the properties.
* @param aComment The description for the properties file.
* @param aDelimiter The path delimiter to be used when writing out the
* properties to the destination (in case the the serialized
* format supports / requires the such).
*
* @return The {@link File} representing the actual location where the
* properties have been saved to.
*
* @throws IOException thrown in case saving the properties failed
*/
default File saveTo( String aFilePath, String aComment, char aDelimiter ) throws IOException {
return saveTo( new File( aFilePath ), aComment, aDelimiter );
}
/**
* Loads a properties file from the file path directly or (if not found)
* from the first folder containing such a file as of the specification
* for the {@link ConfigLocator} (if not provided, then
* {@link ConfigLocator#ALL} is assumed). Finally (if nothing else
* succeeds) the properties are loaded by the provided class's class
* loader which takes care of loading the properties (in case the file
* path is a relative path, also the absolute path with a prefixed path
* delimiter "/" is probed). The default implementation uses the hook
* method {@link #loadFrom(InputStream)} to finally load and parse the
* properties.
*
* @param aResourceClass The class which's class loader is to take care
* of loading the properties (from inside a JAR).
* @param aFilePath The file path from which to load the properties.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default Properties seekFrom( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
return seekFrom( aResourceClass, aFilePath, ConfigLocator.ALL );
}
/**
* Loads a properties file from the file path directly or (if not found)
* from the first folder containing such a file as of the
* {@link ConfigLocator} configuration passed. Finally (if nothing else
* succeeds) the properties are loaded by the provided class's class
* loader which takes care of loading the properties (in case the file
* path is a relative path, also the absolute path with a prefixed path
* delimiter "/" is probed). The default implementation uses the hook
* method {@link #loadFrom(InputStream)} to finally load and parse the
* properties.
*
* @param aResourceClass The class which's class loader is to take care
* of loading the properties (from inside a JAR).
* @param aFilePath The file path from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the
* locations to additional crawl for the desired file.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
Properties seekFrom( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException;
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* for the {@link ConfigLocator} (if not provided, then
* {@link ConfigLocator#ALL} is assumed). The default implementation
* uses the hook method {@link #loadFrom(InputStream)} to finally load
* and parse the properties.
*
* @param aFile The file of the properties file to load.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default Properties seekFrom( File aFile ) throws IOException, ParseException {
return seekFrom( aFile.getPath() );
}
/**
* Loads a properties file from the file directly or (if not found) from
* first folder containing such a file as of the specification for the
* for the {@link ConfigLocator} (if not provided, then
* {@link ConfigLocator#ALL} is assumed). The default implementation
* uses the hook method {@link #loadFrom(InputStream)} to finally load
* and parse the properties.
*
* @param aFile The file of the properties file to load.
* @param aConfigLocator The {@link ConfigLocator} describes the
* locations to additional crawl for the desired file.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default Properties seekFrom( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return seekFrom( aFile.getPath(), aConfigLocator );
}
/**
* Loads a properties file from the file path directly or (if not found)
* from the first folder containing such a file as of the specification
* for the {@link ConfigLocator} (if not provided, then
* {@link ConfigLocator#ALL} is assumed). The default implementation
* uses the hook method {@link #loadFrom(InputStream)} to finally load
* and parse the properties.
*
* @param aFilePath The file path from which to load the properties.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default Properties seekFrom( String aFilePath ) throws IOException, ParseException {
return seekFrom( null, aFilePath );
}
/**
* Loads a properties file from the file path directly or (if not found)
* from the first folder containing such a file as of the specification
* for the {@link ConfigLocator} (if not provided, then
* {@link ConfigLocator#ALL} is assumed). The default implementation
* uses the hook method {@link #loadFrom(InputStream)} to finally load
* and parse the properties.
*
* @param aFilePath The file path from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the
* locations to additional crawl for the desired file.
*
* @return The {@link Properties} as loaded from the resource and
* applied to this instance.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default Properties seekFrom( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return seekFrom( null, aFilePath, aConfigLocator );
}
/**
* {@inheritDoc}
*/
@Override
default String toSerialized( String aComment, char aDelimiter ) {
final ByteArrayOutputStream theOutputStream = new ByteArrayOutputStream();
try {
saveTo( theOutputStream, aComment, aDelimiter );
theOutputStream.flush();
}
catch ( IOException e ) {
throw new BugException( "Encountered bug while creating a stream without any I/O merely in memory!", e );
}
return theOutputStream.toString();
}
// /////////////////////////////////////////////////////////////////////
// SUB-TYPED:
// /////////////////////////////////////////////////////////////////////
}
// /////////////////////////////////////////////////////////////////////////
// BUILDER:
// /////////////////////////////////////////////////////////////////////////
/**
* The interface {@link ResourcePropertiesBuilder} defines builder
* functionality on top of the {@link MutableResoureProperties}.
*/
public interface ResourcePropertiesBuilder extends PropertiesBuilder, MutableResoureProperties, FlushBuilder {
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsert( Object aObj ) {
insert( aObj );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsert( PathMap aFrom ) {
insert( aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( Collection> aToPathElements, Object aFrom, Collection> aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( Collection> aToPathElements, PathMap aFrom, Collection> aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( Object aToPath, Object aFrom, Object aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( Object aToPath, PathMap aFrom, Object aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( Object[] aToPathElements, Object aFrom, Object[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( Object[] aToPathElements, PathMap aFrom, Object[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( String aToPath, Object aFrom, String aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( String aToPath, PathMap aFrom, String aFromPath ) {
insertBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( String[] aToPathElements, Object aFrom, String[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertBetween( String[] aToPathElements, PathMap aFrom, String[] aFromPathElements ) {
insertBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
// /**
// * {@inheritDoc}
// */
// @Override
// default ResourcePropertiesBuilder withPut( Object aPath, String aValue ) {
// put( toPath( aPath ), aValue );
// return this;
// }
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( Object aFrom, Collection> aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( Object aFrom, Object aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( Object aFrom, Object... aFromPathElements ) {
withInsertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( Object aFrom, String aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( Object aFrom, String... aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( PathMap aFrom, Collection> aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( PathMap aFrom, Object aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( PathMap aFrom, Object... aFromPathElements ) {
withInsertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( PathMap aFrom, String aFromPath ) {
insertFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertFrom( PathMap aFrom, String... aFromPathElements ) {
insertFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( Collection> aToPathElements, Object aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( Collection> aToPathElements, PathMap aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( Object aToPath, Object aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( Object aToPath, PathMap aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( Object[] aToPathElements, Object aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( Object[] aToPathElements, PathMap aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( String aToPath, Object aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( String aToPath, PathMap aFrom ) {
insertTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( String[] aToPathElements, Object aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withInsertTo( String[] aToPathElements, PathMap aFrom ) {
insertTo( aToPathElements, aFrom );
return this;
}
/**
* Builder method for {@link #loadFrom(Class, String)}.
*
* @param aResourceClass The class which's class loader is to take care
* of loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withLoadFrom( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
loadFrom( aResourceClass, aFilePath );
return this;
}
/**
* Builder method for {@link #loadFrom(File)}.
*
* @param aFile The according file.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withLoadFrom( File aFile ) throws IOException, ParseException {
loadFrom( aFile );
return this;
}
/**
* Builder method for {@link #loadFrom(InputStream)}.
*
* @param aInputStream The {@link InputStream} from which to load the
* properties.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withLoadFrom( InputStream aInputStream ) throws IOException, ParseException {
loadFrom( aInputStream );
return this;
}
/**
* Builder method for {@link #loadFrom(String)}.
*
* @param aFilePath The according file path.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withLoadFrom( String aFilePath ) throws IOException, ParseException {
loadFrom( aFilePath );
return this;
}
/**
* Builder method for {@link #loadFrom(URL)}.
*
* @param aUrl The {@link URL} from which to load the properties.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withLoadFrom( URL aUrl ) throws IOException, ParseException {
loadFrom( aUrl );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMerge( Object aObj ) {
merge( aObj );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMerge( PathMap aFrom ) {
merge( aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( Collection> aToPathElements, Object aFrom, Collection> aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( Collection> aToPathElements, PathMap aFrom, Collection> aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( Object aToPath, Object aFrom, Object aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( Object aToPath, PathMap aFrom, Object aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( Object[] aToPathElements, Object aFrom, Object[] aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( Object[] aToPathElements, PathMap aFrom, Object[] aFromPathElements ) {
mergeBetween( aToPathElements, aFromPathElements, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( String aToPath, Object aFrom, String aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( String aToPath, PathMap aFrom, String aFromPath ) {
mergeBetween( aToPath, aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( String[] aToPathElements, Object aFrom, String[] aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeBetween( String[] aToPathElements, PathMap aFrom, String[] aFromPathElements ) {
mergeBetween( aToPathElements, aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( Object aFrom, Collection> aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( Object aFrom, Object aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( Object aFrom, Object... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( Object aFrom, String aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( Object aFrom, String... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( PathMap aFrom, Collection> aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( PathMap aFrom, Object aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( PathMap aFrom, Object... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( PathMap aFrom, String aFromPath ) {
mergeFrom( aFrom, aFromPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeFrom( PathMap aFrom, String... aFromPathElements ) {
mergeFrom( aFrom, aFromPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( Collection> aToPathElements, Object aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( Collection> aToPathElements, PathMap aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( Object aToPath, Object aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( Object aToPath, PathMap aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( Object[] aToPathElements, Object aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( Object[] aToPathElements, PathMap aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( String aToPath, Object aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( String aToPath, PathMap aFrom ) {
mergeTo( aToPath, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( String[] aToPathElements, Object aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withMergeTo( String[] aToPathElements, PathMap aFrom ) {
mergeTo( aToPathElements, aFrom );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPut( Collection> aPathElements, String aValue ) {
put( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPut( Object[] aPathElements, String aValue ) {
put( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPut( Property aProperty ) {
put( aProperty );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPut( Relation aProperty ) {
put( aProperty );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPut( String aKey, String aValue ) {
put( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPut( String[] aKey, String aValue ) {
put( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutBoolean( Collection> aPathElements, Boolean aValue ) {
putBoolean( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutBoolean( Object aKey, Boolean aValue ) {
putBoolean( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutBoolean( Object[] aPathElements, Boolean aValue ) {
putBoolean( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutBoolean( String aKey, Boolean aValue ) {
putBoolean( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutBoolean( String[] aPathElements, Boolean aValue ) {
putBoolean( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutByte( Collection> aPathElements, Byte aValue ) {
putByte( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutByte( Object aKey, Byte aValue ) {
putByte( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutByte( Object[] aPathElements, Byte aValue ) {
putByte( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutByte( String aKey, Byte aValue ) {
putByte( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutByte( String[] aPathElements, Byte aValue ) {
putByte( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutChar( Collection> aPathElements, Character aValue ) {
putChar( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutChar( Object aKey, Character aValue ) {
putChar( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutChar( Object[] aPathElements, Character aValue ) {
putChar( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutChar( String aKey, Character aValue ) {
putChar( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutChar( String[] aPathElements, Character aValue ) {
putChar( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutClass( Collection> aPathElements, Class aValue ) {
putClass( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutClass( Object aKey, Class aValue ) {
putClass( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutClass( Object[] aPathElements, Class aValue ) {
putClass( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutClass( String aKey, Class aValue ) {
putClass( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutClass( String[] aPathElements, Class aValue ) {
putClass( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( Collection> aPathElements, int aIndex, Object aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( Collection> aPathElements, int aIndex, PathMap aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( int aIndex, Object aDir ) {
putDirAt( aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( int aIndex, PathMap aDir ) {
putDirAt( aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( Object aPath, int aIndex, Object aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( Object aPath, int aIndex, PathMap aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( Object[] aPathElements, int aIndex, Object aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( Object[] aPathElements, int aIndex, PathMap aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( String aPath, int aIndex, Object aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( String aPath, int aIndex, PathMap aDir ) {
putDirAt( aPath, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( String[] aPathElements, int aIndex, Object aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDirAt( String[] aPathElements, int aIndex, PathMap aDir ) {
putDirAt( aPathElements, aIndex, aDir );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDouble( Collection> aPathElements, Double aValue ) {
putDouble( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDouble( Object aKey, Double aValue ) {
putDouble( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDouble( Object[] aPathElements, Double aValue ) {
putDouble( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDouble( String aKey, Double aValue ) {
putDouble( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutDouble( String[] aPathElements, Double aValue ) {
putDouble( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ResourcePropertiesBuilder withPutEnum( Collection> aPathElements, E aValue ) {
putEnum( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ResourcePropertiesBuilder withPutEnum( Object aKey, E aValue ) {
putEnum( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ResourcePropertiesBuilder withPutEnum( Object[] aPathElements, E aValue ) {
putEnum( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ResourcePropertiesBuilder withPutEnum( String aKey, E aValue ) {
putEnum( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default > ResourcePropertiesBuilder withPutEnum( String[] aPathElements, E aValue ) {
putEnum( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutFloat( Collection> aPathElements, Float aValue ) {
putFloat( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutFloat( Object aKey, Float aValue ) {
putFloat( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutFloat( Object[] aPathElements, Float aValue ) {
putFloat( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutFloat( String aKey, Float aValue ) {
putFloat( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutFloat( String[] aPathElements, Float aValue ) {
putFloat( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutInt( Collection> aPathElements, Integer aValue ) {
putInt( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutInt( Object aKey, Integer aValue ) {
putInt( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutInt( Object[] aPathElements, Integer aValue ) {
putInt( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutInt( String aKey, Integer aValue ) {
putInt( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutInt( String[] aPathElements, Integer aValue ) {
putInt( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutLong( Collection> aPathElements, Long aValue ) {
putLong( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutLong( Object aKey, Long aValue ) {
putLong( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutLong( Object[] aPathElements, Long aValue ) {
putLong( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutLong( String aKey, Long aValue ) {
putLong( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutLong( String[] aPathElements, Long aValue ) {
putLong( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutShort( Collection> aPathElements, Short aValue ) {
putShort( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutShort( Object aKey, Short aValue ) {
putShort( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutShort( Object[] aPathElements, Short aValue ) {
putShort( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutShort( String aKey, Short aValue ) {
putShort( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutShort( String[] aPathElements, Short aValue ) {
putShort( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutString( Collection> aPathElements, String aValue ) {
putString( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutString( Object aKey, String aValue ) {
putString( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutString( Object[] aPathElements, String aValue ) {
putString( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutString( String aKey, String aValue ) {
putString( aKey, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withPutString( String[] aPathElements, String aValue ) {
putString( aPathElements, aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withRemoveFrom( Collection> aPathElements ) {
removeFrom( aPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withRemoveFrom( Object aPath ) {
removeFrom( aPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withRemoveFrom( Object... aPathElements ) {
removeFrom( aPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withRemoveFrom( String aPath ) {
removeFrom( aPath );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withRemoveFrom( String... aPathElements ) {
removeFrom( aPathElements );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder withRemovePaths( String... aPathElements ) {
removeFrom( aPathElements );
return this;
}
/**
* Builder method for {@link #seekFrom(Class, String)}.
*
* @param aResourceClass The class which's class loader is to take care
* of loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withSeekFrom( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
seekFrom( aResourceClass, aFilePath );
return this;
}
/**
* Builder method for {@link #seekFrom(File)}.
*
* @param aFile The according file path.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withSeekFrom( File aFile ) throws IOException, ParseException {
seekFrom( aFile );
return this;
}
/**
* Builder method for {@link #seekFrom(String)}.
*
* @param aFilePath The according file path.
*
* @return The implementing instance as of the builder pattern.
*
* @throws IOException thrown in case accessing or processing the
* properties file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourcePropertiesBuilder withSeekFrom( String aFilePath ) throws IOException, ParseException {
seekFrom( aFilePath );
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy