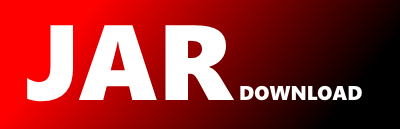
org.refcodes.properties.ResourcePropertiesFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.text.ParseException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.refcodes.data.Delimiter;
import org.refcodes.mixin.FilenameSuffixesAccessor;
import org.refcodes.properties.Properties.PropertiesBuilder;
import org.refcodes.properties.ResourceProperties.ResourcePropertiesBuilder;
import org.refcodes.runtime.ConfigLocator;
import org.refcodes.struct.PathMap.MutablePathMap;
/**
* Factory interface for creating {@link ResourceProperties} instances.
*/
public interface ResourcePropertiesFactory extends FilenameSuffixesAccessor {
/**
* Determines whether this factory may be responsible for a given file.
*
* @param aFile The file which's suffix is to be tested.
*
* @return True in case this factory may be responsible, else false.
*/
default boolean hasFilenameSuffix( File aFile ) {
if ( aFile != null ) {
return hasFilenameSuffix( aFile.getAbsolutePath() );
}
return false;
}
/**
* Determines whether this factory may be responsible for a given file.
*
* @param aFilePath The path of the file which's suffix is to be tested.
*
* @return True in case this factory may be responsible, else false.
*/
default boolean hasFilenameSuffix( String aFilePath ) {
if ( getFilenameSuffixes() != null && aFilePath != null ) {
final String theLowerCase = aFilePath.toLowerCase();
for ( String eSuffix : getFilenameSuffixes() ) {
if ( eSuffix != null ) {
if ( theLowerCase.endsWith( eSuffix.toLowerCase() ) ) {
return true;
}
}
}
}
return false;
}
/**
* Loads the properties from the given file's path.
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourceProperties toProperties( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
return toProperties( aResourceClass, aFilePath, null );
}
/**
* Loads the properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file. Finally (if nothing else succeeds) the properties are
* loaded by the provided class's class loader which takes care of loading
* the properties (in case the file path is a relative path, also the
* absolute path with a prefixed path delimiter "/" is probed).
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
ResourceProperties toProperties( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException;
/**
* Loads the properties from the given {@link File}.
*
* @param aFile The {@link File} from which to load the properties.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourceProperties toProperties( File aFile ) throws IOException, ParseException {
return toProperties( aFile, null );
}
/**
* Loads or seeks the properties from the given {@link File}. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file.
*
* @param aFile The {@link File} from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
ResourceProperties toProperties( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException;
// /////////////////////////////////////////////////////////////////////////
// DEFAULT:
// /////////////////////////////////////////////////////////////////////////
/**
* Reads the properties from the given {@link InputStream}.
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
ResourceProperties toProperties( InputStream aInputStream ) throws IOException, ParseException;
/**
* Create a {@link ResourceProperties} instance containing the elements of
* the provided {@link Map} instance using the path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*
* @return The accordingly constructed {@link ResourceProperties}.
*/
ResourceProperties toProperties( Map, ?> aProperties );
/**
* Create a {@link ResourceProperties} instance containing the elements as
* of {@link MutablePathMap#insert(Object)} using the path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations: "Inspects the given
* object and adds all elements found in the given object. Elements of type
* {@link Map}, {@link Collection} and arrays are identified and handled as
* of their type: The path for each value in a {@link Map} is appended with
* its according key. The path for each value in a {@link Collection} or
* array is appended with its according index of occurrence (in case of a
* {@link List} or an array, its actual index). In case of reflection, the
* path for each member is appended with its according mamber's name. All
* elements (e.g. the members and values) are inspected recursively which
* results in the according paths of the terminating values."
*
* @param aObj The object from which the elements are to be added.
*
* @return The accordingly constructed {@link ResourceProperties}.
*/
ResourceProperties toProperties( Object aObj );
/**
* Create a {@link ResourceProperties} instance containing the elements of
* the provided {@link Properties} instance using the path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*
* @return The accordingly constructed {@link ResourceProperties}.
*/
ResourceProperties toProperties( Properties aProperties );
/**
* Create a {@link ResourceProperties} instance containing the elements of
* the provided {@link PropertiesBuilder} instance using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*
* @return The accordingly constructed {@link ResourceProperties}.
*/
ResourceProperties toProperties( PropertiesBuilder aProperties );
/**
* Loads the properties from the given file's path.
*
* @param aFilePath The path to the file from which to load the properties.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourceProperties toProperties( String aFilePath ) throws IOException, ParseException {
return toProperties( null, aFilePath, null );
}
/**
* Loads the properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file.
*
* @param aFilePath The path to the file from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
default ResourceProperties toProperties( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return toProperties( null, aFilePath, aConfigLocator );
}
/**
* Loads the properties from the given {@link URL}.
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @return The accordingly constructed {@link ResourceProperties}.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
ResourceProperties toProperties( URL aUrl ) throws IOException, ParseException;
// /////////////////////////////////////////////////////////////////////////
// BUILDER FACTORY:
// /////////////////////////////////////////////////////////////////////////
/**
* Factory interface for creating {@link ResourcePropertiesBuilder}
* instances.
*/
public interface ResourcePropertiesBuilderFactory extends ResourcePropertiesFactory {
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder toProperties( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
return toProperties( aResourceClass, aFilePath, null );
}
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException;
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder toProperties( File aFile ) throws IOException, ParseException {
return toProperties( aFile, null );
}
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException;
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( InputStream aInputStream ) throws IOException, ParseException;
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( Map, ?> aProperties );
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( Object aObj );
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( Properties aProperties );
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( PropertiesBuilder aProperties );
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder toProperties( String aFilePath ) throws IOException, ParseException {
return toProperties( null, aFilePath, null );
}
/**
* {@inheritDoc}
*/
@Override
default ResourcePropertiesBuilder toProperties( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return toProperties( null, aFilePath, aConfigLocator );
}
/**
* {@inheritDoc}
*/
@Override
ResourcePropertiesBuilder toProperties( URL aUrl ) throws IOException, ParseException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy