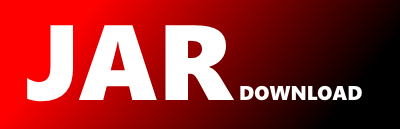
org.refcodes.properties.TomlPropertiesBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-properties Show documentation
Show all versions of refcodes-properties Show documentation
This artifact provides means to read configuration data from various
different locations such as properties from JAR files, file system
files or remote HTTP addresses or GIT repositories.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.text.ParseException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.refcodes.data.Delimiter;
import org.refcodes.data.FilenameExtension;
import org.refcodes.properties.ResourcePropertiesFactory.ResourcePropertiesBuilderFactory;
import org.refcodes.runtime.ConfigLocator;
import org.refcodes.struct.ext.factory.CanonicalMapFactory;
import org.refcodes.struct.ext.factory.TomlCanonicalMapFactory;
import org.refcodes.struct.ext.factory.XmlCanonicalMapFactory;
/**
* Implementation of the {@link ResourcePropertiesBuilder} interface with
* support of so called "TOML properties". In addition, nested sections are
* supported: A section represents the first portion of a path and looks like
* such:
* [SectionA]
* ValueA=A
*
This results in key/value property of:
* SectionA/ValueA=A
*
* [SectionA]
* ValueA=A
*
* [[SectionB]]
* ValueB=B
*
* SectionA/ValueA=A
* SectionA/SectionB/ValueB=B
*
For TOML properties, see "https://en.wikipedia.org/wiki/TOML"
*/
public class TomlPropertiesBuilder extends AbstractResourcePropertiesBuilder {
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Create an empty {@link TomlPropertiesBuilder} instance using the default
* path delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*/
public TomlPropertiesBuilder() {}
/**
* Loads the TOML properties from the given file's path.
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
super( aResourceClass, aFilePath );
}
/**
* Loads the TOML properties from the given file's path.
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( Class> aResourceClass, String aFilePath, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aResourceClass, aFilePath, aDocumentMetrics );
}
/**
* Loads the TOML properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file. Finally (if nothing else succeeds) the properties are
* loaded by the provided class's class loader which takes care of loading
* the properties (in case the file path is a relative path, also the
* absolute path with a prefixed path delimiter "/" is probed).
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
super( aResourceClass, aFilePath, aConfigLocator );
}
/**
* Loads the TOML properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file. Finally (if nothing else succeeds) the properties are
* loaded by the provided class's class loader which takes care of loading
* the properties (in case the file path is a relative path, also the
* absolute path with a prefixed path delimiter "/" is probed). *
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aResourceClass, aFilePath, aConfigLocator, aDocumentMetrics );
}
/**
* Loads the TOML properties from the given {@link File}.
*
* @param aFile The {@link File} from which to load the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( File aFile ) throws IOException, ParseException {
super( aFile );
}
/**
* Loads the TOML properties from the given {@link File}.
*
* @param aFile The {@link File} from which to load the properties.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( File aFile, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aFile, aDocumentMetrics );
}
/**
* Loads or seeks the TOML properties from the given {@link File}. A
* provided {@link ConfigLocator} describes the locations to additional
* crawl for the desired file.
*
* @param aFile The {@link File} from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException {
super( aFile, aConfigLocator );
}
/**
* Loads or seeks the TOML properties from the given {@link File}. A
* provided {@link ConfigLocator} describes the locations to additional
* crawl for the desired file.
*
* @param aFile The {@link File} from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( File aFile, ConfigLocator aConfigLocator, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aFile, aConfigLocator, aDocumentMetrics );
}
/**
* Reads the TOML properties from the given {@link InputStream}.
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( InputStream aInputStream ) throws IOException, ParseException {
super( aInputStream );
}
/**
* Reads the TOML properties from the given {@link InputStream}.
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( InputStream aInputStream, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aInputStream, aDocumentMetrics );
}
/**
* Create a {@link TomlPropertiesBuilder} instance containing the elements
* of the provided {@link Map} instance using the default path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public TomlPropertiesBuilder( Map, ?> aProperties ) {
super( aProperties );
}
/**
* Create a {@link TomlPropertiesBuilder} instance containing the elements
* of the provided {@link Map} instance using the default path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
* @param aDocumentMetrics the document metrics
*/
public TomlPropertiesBuilder( Map, ?> aProperties, DocumentMetrics aDocumentMetrics ) {
super( aProperties, aDocumentMetrics );
}
/**
* Create a {@link TomlPropertiesBuilder} instance containing the elements
* as of {@link MutablePathMap#insert(Object)} using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations:
* "Inspects the given object and adds all elements found in the given
* object. Elements of type {@link Map}, {@link Collection} and arrays are
* identified and handled as of their type: The path for each value in a
* {@link Map} is appended with its according key. The path for each value
* in a {@link Collection} or array is appended with its according index of
* occurrence (in case of a {@link List} or an array, its actual index). In
* case of reflection, the path for each member is appended with its
* according mamber's name. All elements (e.g. the members and values) are
* inspected recursively which results in the according paths of the
* terminating values."
*
* @param aObj The object from which the elements are to be added.
*/
public TomlPropertiesBuilder( Object aObj ) {
super( aObj );
}
/**
* Create a {@link TomlPropertiesBuilder} instance containing the elements
* as of {@link MutablePathMap#insert(Object)} using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations:
* "Inspects the given object and adds all elements found in the given
* object. Elements of type {@link Map}, {@link Collection} and arrays are
* identified and handled as of their type: The path for each value in a
* {@link Map} is appended with its according key. The path for each value
* in a {@link Collection} or array is appended with its according index of
* occurrence (in case of a {@link List} or an array, its actual index). In
* case of reflection, the path for each member is appended with its
* according mamber's name. All elements (e.g. the members and values) are
* inspected recursively which results in the according paths of the
* terminating values."
*
* @param aObj The object from which the elements are to be added.
* @param aDocumentMetrics the document metrics
*/
public TomlPropertiesBuilder( Object aObj, DocumentMetrics aDocumentMetrics ) {
super( aObj, aDocumentMetrics );
}
/**
* Create a {@link TomlPropertiesBuilder} instance containing the elements
* of the provided {@link Properties} instance using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public TomlPropertiesBuilder( Properties aProperties ) {
super( aProperties );
}
/**
* /** Create a {@link TomlPropertiesBuilder} instance containing the
* elements of the provided {@link PropertiesBuilder} instance using the
* default path delimiter "/" ({@link Delimiter#PATH}) for the path
* declarations.
*
* @param aProperties the properties to be added.
* @param aDocumentMetrics the document metrics
*/
public TomlPropertiesBuilder( Properties aProperties, DocumentMetrics aDocumentMetrics ) {
super( aProperties, aDocumentMetrics );
}
/**
* Create a {@link TomlPropertiesBuilder} instance containing the elements
* of the provided {@link PropertiesBuilder} instance using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public TomlPropertiesBuilder( PropertiesBuilder aProperties ) {
super( aProperties );
}
/**
* Create a {@link TomlPropertiesBuilder} instance containing the elements
* of the provided {@link PropertiesBuilder} instance using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
* @param aDocumentMetrics the document metrics
*/
public TomlPropertiesBuilder( PropertiesBuilder aProperties, DocumentMetrics aDocumentMetrics ) {
super( aProperties, aDocumentMetrics );
}
/**
* Loads the TOML properties from the given file's path.
*
* @param aFilePath The path to the file from which to load the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( String aFilePath ) throws IOException, ParseException {
super( aFilePath );
}
/**
* Loads the TOML properties from the given file's path.
*
* @param aFilePath The path to the file from which to load the properties.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( String aFilePath, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aFilePath, aDocumentMetrics );
}
/**
* Loads the TOML properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file.
*
* @param aFilePath The path to the file from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
super( aFilePath, aConfigLocator );
}
/**
* Loads the TOML properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file.
*
* @param aFilePath The path to the file from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( String aFilePath, ConfigLocator aConfigLocator, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aFilePath, aConfigLocator, aDocumentMetrics );
}
/**
* Loads the TOML properties from the given {@link URL}.
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( URL aUrl ) throws IOException, ParseException {
super( aUrl );
}
/**
* Loads the TOML properties from the given {@link URL}.
*
* @param aUrl The {@link URL} from which to read the properties.
* @param aDocumentMetrics the document metrics
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public TomlPropertiesBuilder( URL aUrl, DocumentMetrics aDocumentMetrics ) throws IOException, ParseException {
super( aUrl, aDocumentMetrics );
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
protected CanonicalMapFactory createCanonicalMapFactory() {
return new TomlCanonicalMapFactory();
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* The {@link TomlPropertiesBuilderFactory} represents a
* {@link ResourcePropertiesBuilderFactory} creating instances of type
* {@link TomlPropertiesBuilder}.
*/
public static class TomlPropertiesBuilderFactory implements ResourcePropertiesBuilderFactory {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private final DocumentMetrics _documentMetrics;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////
/**
* Constructs an {@link XmlCanonicalMapFactory} for creating
* {@link XmlPropertiesBuilder} instances.
*/
public TomlPropertiesBuilderFactory() {
this( DocumentNotation.DEFAULT );
}
/**
* Constructs an {@link XmlCanonicalMapFactory} for creating
* {@link XmlPropertiesBuilder} instances.
*
* @param aDocumentMetrics the document metrics
*/
public TomlPropertiesBuilderFactory( DocumentMetrics aDocumentMetrics ) {
_documentMetrics = aDocumentMetrics;
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String[] getFilenameSuffixes() {
return new String[] { FilenameExtension.TOML.getFilenameSuffix(), FilenameExtension.INI.getFilenameSuffix() };
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aResourceClass, aFilePath, aConfigLocator, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aFile, aConfigLocator, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( InputStream aInputStream ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aInputStream, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Map, ?> aProperties ) {
return new TomlPropertiesBuilder( aProperties, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Object aObj ) {
return new TomlPropertiesBuilder( aObj, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Properties aProperties ) {
return new TomlPropertiesBuilder( aProperties, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( PropertiesBuilder aProperties ) {
return new TomlPropertiesBuilder( aProperties, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aFilePath, aConfigLocator, _documentMetrics );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( URL aUrl ) throws IOException, ParseException {
return new TomlPropertiesBuilder( aUrl, _documentMetrics );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy