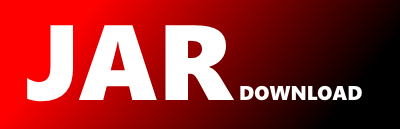
org.refcodes.properties.YamlPropertiesBuilder Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.properties;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.text.ParseException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.refcodes.data.Delimiter;
import org.refcodes.data.FilenameExtension;
import org.refcodes.properties.ResourcePropertiesFactory.ResourcePropertiesBuilderFactory;
import org.refcodes.runtime.ConfigLocator;
import org.refcodes.struct.ext.factory.CanonicalMapFactory;
import org.refcodes.struct.ext.factory.YamlCanonicalMapFactory;
/**
* Implementation of the {@link ResourcePropertiesBuilder} interface with
* support of so called "YAML properties" (or just "properties"). For YAML, see
* "https://en.wikipedia.org/wiki/YAML".
*/
public class YamlPropertiesBuilder extends AbstractResourcePropertiesBuilder {
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
public static final char COMMENT = '#';
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Create an empty {@link YamlPropertiesBuilder} instance using the default
* path delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*/
public YamlPropertiesBuilder() {}
/**
* Loads the YAML properties from the given file's path.
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( Class> aResourceClass, String aFilePath ) throws IOException, ParseException {
super( aResourceClass, aFilePath );
}
/**
* Loads the YAML properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file. Finally (if nothing else succeeds) the properties are
* loaded by the provided class's class loader which takes care of loading
* the properties (in case the file path is a relative path, also the
* absolute path with a prefixed path delimiter "/" is probed).
*
* @param aResourceClass The class which's class loader is to take care of
* loading the properties (from inside a JAR).
* @param aFilePath The file path of the class's resources from which to
* load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
super( aResourceClass, aFilePath, aConfigLocator );
}
/**
* Loads the YAML properties from the given {@link File}.
*
* @param aFile The {@link File} from which to load the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( File aFile ) throws IOException, ParseException {
super( aFile );
}
/**
* Loads or seeks the YAML properties from the given {@link File}. A
* provided {@link ConfigLocator} describes the locations to additional
* crawl for the desired file.
*
* @param aFile The {@link File} from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException {
super( aFile, aConfigLocator );
}
/**
* Reads the YAML properties from the given {@link InputStream}.
*
* @param aInputStream The {@link InputStream} from which to read the
* properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( InputStream aInputStream ) throws IOException, ParseException {
super( aInputStream );
}
/**
* Create a {@link YamlPropertiesBuilder} instance containing the elements
* of the provided {@link Map} instance using the default path delimiter "/"
* ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public YamlPropertiesBuilder( Map, ?> aProperties ) {
super( aProperties );
}
/**
* Create a {@link YamlPropertiesBuilder} instance containing the elements
* as of {@link MutablePathMap#insert(Object)} using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations:
* "Inspects the given object and adds all elements found in the given
* object. Elements of type {@link Map}, {@link Collection} and arrays are
* identified and handled as of their type: The path for each value in a
* {@link Map} is appended with its according key. The path for each value
* in a {@link Collection} or array is appended with its according index of
* occurrence (in case of a {@link List} or an array, its actual index). In
* case of reflection, the path for each member is appended with its
* according mamber's name. All elements (e.g. the members and values) are
* inspected recursively which results in the according paths of the
* terminating values."
*
* @param aObj The object from which the elements are to be added.
*/
public YamlPropertiesBuilder( Object aObj ) {
super( aObj );
}
/**
* Create a {@link YamlPropertiesBuilder} instance containing the elements
* of the provided {@link Properties} instance using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public YamlPropertiesBuilder( Properties aProperties ) {
super( aProperties );
}
/**
* Create a {@link YamlPropertiesBuilder} instance containing the elements
* of the provided {@link PropertiesBuilder} instance using the default path
* delimiter "/" ({@link Delimiter#PATH}) for the path declarations.
*
* @param aProperties the properties to be added.
*/
public YamlPropertiesBuilder( PropertiesBuilder aProperties ) {
super( aProperties );
}
/**
* Loads the YAML properties from the given file's path.
*
* @param aFilePath The path to the file from which to load the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( String aFilePath ) throws IOException, ParseException {
super( aFilePath );
}
/**
* Loads the YAML properties from the given file's path. A provided
* {@link ConfigLocator} describes the locations to additional crawl for the
* desired file.
*
* @param aFilePath The path to the file from which to load the properties.
* @param aConfigLocator The {@link ConfigLocator} describes the locations
* to additional crawl for the desired file.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
super( aFilePath, aConfigLocator );
}
/**
* Loads the YAML properties from the given {@link URL}.
*
* @param aUrl The {@link URL} from which to read the properties.
*
* @throws IOException thrown in case accessing or processing the properties
* file failed.
* @throws ParseException Signals that an error has been reached
* unexpectedly while parsing the data to be loaded.
*/
public YamlPropertiesBuilder( URL aUrl ) throws IOException, ParseException {
super( aUrl );
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
protected CanonicalMapFactory createCanonicalMapFactory() {
return new YamlCanonicalMapFactory();
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* The {@link YamlPropertiesBuilderFactory} represents a
* {@link ResourcePropertiesBuilderFactory} creating instances of type
* {@link YamlPropertiesBuilder}.
*/
public static class YamlPropertiesBuilderFactory implements ResourcePropertiesBuilderFactory {
/**
* {@inheritDoc}
*/
@Override
public String[] getFilenameSuffixes() {
return new String[] { FilenameExtension.YAML.getFilenameSuffix() };
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Class> aResourceClass, String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aResourceClass, aFilePath, aConfigLocator );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( File aFile, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aFile, aConfigLocator );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( InputStream aInputStream ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aInputStream );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Map, ?> aProperties ) {
return new YamlPropertiesBuilder( aProperties );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Object aObj ) {
return new YamlPropertiesBuilder( aObj );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( Properties aProperties ) {
return new YamlPropertiesBuilder( aProperties );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( PropertiesBuilder aProperties ) {
return new YamlPropertiesBuilder( aProperties );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( String aFilePath, ConfigLocator aConfigLocator ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aFilePath, aConfigLocator );
}
/**
* {@inheritDoc}
*/
@Override
public ResourcePropertiesBuilder toProperties( URL aUrl ) throws IOException, ParseException {
return new YamlPropertiesBuilder( aUrl );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy