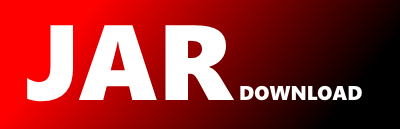
org.refcodes.remoting.RemoteClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-remoting Show documentation
Show all versions of refcodes-remoting Show documentation
Artifact with proxy functionality for plain remote procedure calls
(RPC), a POJO alternative to RMI.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.remoting;
import java.io.IOException;
import java.lang.reflect.InvocationHandler;
import java.util.Iterator;
import org.refcodes.mixin.BusyAccessor;
import org.refcodes.mixin.Disposable.Disposedable;
import org.refcodes.mixin.Lockable;
/**
* Remote control providing subjects to be operated on by a
* {@link RemoteServer}.
*/
public interface RemoteClient extends Remote {
/**
* Returns true if the provided proxy is contained inside the
* {@link RemoteClient}.
*
* @param aProxy The proxy to be tested if it is contained inside the
* {@link RemoteClient}.
*
* @return True if the given proxy is contained inside the
* {@link RemoteClient}.
*/
boolean hasProxy( Object aProxy );
/**
* Returns a read-only iterator containing all the proxy objects previously
* being published. Use the sign-off methods in order to remove a published
* proxy object.
*
* @return An iterator containing the published proxy objects.
*/
Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy