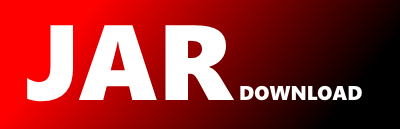
org.refcodes.rest.ext.eureka.EurekaDiscoverySidecarImpl Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.rest.ext.eureka;
import java.net.MalformedURLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Timer;
import java.util.TimerTask;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.ThreadLocalRandom;
import org.refcodes.component.Destroyable;
import org.refcodes.component.InitializeException;
import org.refcodes.component.LifeCycleStatus;
import org.refcodes.component.OpenException;
import org.refcodes.component.PauseException;
import org.refcodes.component.ResumeException;
import org.refcodes.component.StartException;
import org.refcodes.component.Startable;
import org.refcodes.component.StopException;
import org.refcodes.component.Stoppable;
import org.refcodes.exception.BugException;
import org.refcodes.exception.ExceptionUtility;
import org.refcodes.logger.RuntimeLogger;
import org.refcodes.logger.RuntimeLoggerFactorySingleton;
import org.refcodes.net.HttpBodyMap;
import org.refcodes.net.HttpStatusException;
import org.refcodes.net.LoadBalancingStrategy;
import org.refcodes.net.Url;
import org.refcodes.net.Url.UrlBuilder;
import org.refcodes.net.UrlBuilderImpl;
import org.refcodes.net.UrlImpl;
import org.refcodes.rest.AbstractHttpDiscoverySidecar;
import org.refcodes.rest.HttpRestClient;
import org.refcodes.rest.HttpRestClientImpl;
import org.refcodes.rest.RestResponse;
import org.refcodes.security.TrustStoreDescriptor;
/**
* The {@link EurekaDiscoverySidecarImpl} decorates a {@link HttpRestClient}
* with functionality such registering and unregistering from / to a Eureka
* discovery service.
*/
public class EurekaDiscoverySidecarImpl extends AbstractHttpDiscoverySidecar implements EurekaDiscoverySidecar {
private static RuntimeLogger LOGGER = RuntimeLoggerFactorySingleton.createRuntimeLogger();
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private ExecutorService _executorService;
private RefreshDaemon _refreshDaemon;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs a {@link EurekaDiscoverySidecar} with discovery functionality.
*/
public EurekaDiscoverySidecarImpl() {
this( null );
}
/**
* Constructs a {@link EurekaDiscoverySidecar} with discovery functionality.
*
* @param aExecutorService An executor service to be used when creating
* {@link Thread}s.
*/
public EurekaDiscoverySidecarImpl( ExecutorService aExecutorService ) {
_executorService = aExecutorService;
}
// /////////////////////////////////////////////////////////////////////////
// INJECTION:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// LIFE-CYCLE:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void initialize( Url aDiscoveryUrl, LoadBalancingStrategy aStrategy, TrustStoreDescriptor aStoreDescriptor ) throws InitializeException {
aDiscoveryUrl = toHttpDiscoveryUrl( aDiscoveryUrl, this );
aStrategy = toLoadBalancingStrategy( aStrategy, this );
super.initialize();
setLoadBalancingStrategy( aStrategy );
try {
_refreshDaemon = new RefreshDaemon( aDiscoveryUrl, aStoreDescriptor, this, _executorService );
}
catch ( Exception e ) {
_lifeCycleAutomaton.setLifeCycleStatus( LifeCycleStatus.ERROR );
throw new InitializeException( ExceptionUtility.toMessage( e ), e );
}
}
@Override
public synchronized void start() throws StartException {
try {
super.start();
_refreshDaemon.start();
}
catch ( Exception e ) {
_lifeCycleAutomaton.setLifeCycleStatus( LifeCycleStatus.ERROR );
throw new StartException( ExceptionUtility.toMessage( e ), e );
}
}
@Override
public synchronized void pause() throws PauseException {
super.pause();
try {}
catch ( Exception e ) {
_lifeCycleAutomaton.setLifeCycleStatus( LifeCycleStatus.ERROR );
throw new PauseException( ExceptionUtility.toMessage( e ), e );
}
}
@Override
public synchronized void stop() throws StopException {
super.stop();
try {
_refreshDaemon.stop();
}
catch ( Exception e ) {
_lifeCycleAutomaton.setLifeCycleStatus( LifeCycleStatus.ERROR );
throw new StopException( ExceptionUtility.toMessage( e ), e );
}
}
@Override
public synchronized void resume() throws ResumeException {
super.resume();
try {}
catch ( Exception e ) {
_lifeCycleAutomaton.setLifeCycleStatus( LifeCycleStatus.ERROR );
throw new ResumeException( ExceptionUtility.toMessage( e ), e );
}
}
@Override
public synchronized void destroy() {
super.destroy();
try {
_refreshDaemon.destroy();
}
catch ( Exception e ) {
LOGGER.warn( ExceptionUtility.toMessage( e ), e );
_lifeCycleAutomaton.setLifeCycleStatus( LifeCycleStatus.ERROR );
}
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Url toUrl( Url aUrl ) {
return toUrl( aUrl, this, _refreshDaemon );
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
protected static Url toUrl( Url aUrl, EurekaDiscovery> aDiscovery, RefreshDaemon aRefreshDaemon ) {
String theHost = aUrl.getHost();
if ( theHost != null ) {
theHost = theHost.toUpperCase();
}
List theInstances = aRefreshDaemon.getInstance( theHost );
if ( theInstances != null ) {
EurekaInstanceDescriptor[] theArray = theInstances.toArray( new EurekaInstanceDescriptor[theInstances.size()] );
if ( theArray.length > 0 ) {
switch ( aDiscovery.getLoadBalancingStrategy() ) {
case CUSTOM:
throw new IllegalStateException( "The load balancing satrategy <" + LoadBalancingStrategy.CUSTOM + "> is cunnrently not supported." );
case NONE:
break;
case RANDOM:
int theRnd = ThreadLocalRandom.current().nextInt( 0, theArray.length );
UrlBuilder theUrl = new UrlBuilderImpl( aUrl );
int[] theIAddr = theArray[theRnd].getIpAddress();
// Prefer IP over host, host may abused as instance ID |-->
if ( theIAddr != null ) {
theUrl.setIpAddress( theIAddr );
}
else {
theUrl.setHost( theArray[theRnd].getHost() );
}
// Prefer IP over host, host may abused as instance ID <--|
theUrl.setPort( theArray[theRnd].getPort() );
return theUrl;
case RANDOM_STICKY:
throw new IllegalStateException( "The load balancing satrategy <" + LoadBalancingStrategy.RANDOM_STICKY + "> is cunnrently not supported." );
case ROUND_ROBIN:
throw new IllegalStateException( "The load balancing satrategy <" + LoadBalancingStrategy.ROUND_ROBIN + "> is cunnrently not supported." );
default:
throw new BugException( "Missing case statement for <" + aDiscovery.getLoadBalancingStrategy() + "> in implementation!" );
}
}
}
return aUrl;
}
/**
* Resolves the property from the provided value and the provided property
* and sets the property in case the provided value is not null.
*
* @param aDiscoveryUrl The value to be used when not null.
* @param aProperty The property to be used when the value is null and which
* is to be set when the value is not null.
*
* @return The value when not null, else the value of the provided property.
*/
protected static Url toHttpDiscoveryUrl( Url aDiscoveryUrl, HttpDiscoveryUrlProperty aProperty ) {
if ( aDiscoveryUrl != null ) {
aProperty.setHttpDiscoveryUrl( aDiscoveryUrl );
}
else {
aDiscoveryUrl = aProperty.getHttpDiscoveryUrl();
}
if ( aDiscoveryUrl != null && aDiscoveryUrl.getPath() == null ) {
aDiscoveryUrl = new UrlImpl( aDiscoveryUrl, EurekaRestServer.EUREKA_BASE_PATH );
aProperty.setHttpDiscoveryUrl( aDiscoveryUrl );
}
return aDiscoveryUrl;
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* Attention: This class is package local! As it does some well known casts
* which are not obvious from the constructor's signature!
*
* !!! ONLY INTENDED TO BE USED BY THE MAINTAINER OF THIS CLASS !!!
*/
static class RefreshDaemon extends TimerTask implements Startable, Stoppable, Destroyable {
// /////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////
private Map> _instances;
private EurekaDiscovery> _discovery;
private ExecutorService _executorService;
private Timer _scheduler;
// /////////////////////////////////////////////////////////////////////
// CONSTRUCTIRS:
// /////////////////////////////////////////////////////////////////////
/**
* Attention: This constructor is package local! As it does some well
* known casts which are not obvious from the constructor's signature!
*
* !!! ONLY INTENDED TO BE USED BY THE MAINTAINER OF THIS CLASS !!!
*/
RefreshDaemon( Url aDiscoveryUrl, TrustStoreDescriptor aStoreDescriptor, EurekaDiscovery> aDiscovery, ExecutorService aExecutorService ) throws HttpStatusException, OpenException, MalformedURLException {
_executorService = aExecutorService;
_discovery = aDiscovery;
_instances = loadRegisteredServices( aDiscoveryUrl, aStoreDescriptor );
}
// /////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////
/**
* Returns all instances retrieved from the targeted Eureka service.
*
* @return The instances as retrieved from the Eureka service.
*/
public Map> getInstances() {
return _instances;
}
/**
* Returns the instance represented by the given alias or null if none
* such instance was found.
*
* @param aAlias The alias for which to resolve the instance.
*
* @return The according instance or null if none such instance was
* found for the given alias.
*/
public List getInstance( String aAlias ) {
return _instances.get( aAlias );
}
// /////////////////////////////////////////////////////////////////////
// SCHEDULER:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void start() {
_scheduler = new Timer( true );
_scheduler.schedule( this, EurekaLoopSleepTime.DISCOVERY_SERVICE_REFRESH.getMillis(), EurekaLoopSleepTime.DISCOVERY_SERVICE_REFRESH.getMillis() );
}
/**
* {@inheritDoc}
*/
@Override
public void stop() {
_scheduler.cancel();
}
/**
* {@inheritDoc}
*/
@Override
public void destroy() {
_scheduler.cancel();
_discovery = null;
_executorService = null;
_instances.clear();
_instances = null;
}
// /////////////////////////////////////////////////////////////////////
// DAEMON:
// /////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void run() {
if ( _discovery.isRunning() ) {
LOGGER.info( "Refreshing clients from <" + _discovery.getHttpDiscoveryUrl().toHttpUrl() + ">..." );
try {
Map> theNewInstances = loadRegisteredServices( _discovery.getHttpDiscoveryUrl(), ((TrustStoreDescriptorProperty) _discovery).getTrustStoreDescriptor() );
List eInstances;
for ( String eInstanceId : theNewInstances.keySet() ) {
synchronized ( this ) {
eInstances = toInstancesUpdate( theNewInstances.get( eInstanceId ), _instances.get( eInstanceId ) );
_instances.put( eInstanceId, eInstances );
}
}
}
catch ( HttpStatusException | OpenException | MalformedURLException e ) {
LOGGER.warn( "Encountered an exception of type <" + e.getClass().getName() + "> while refreshing the clients from discovery registry <" + _discovery.getHttpDiscoveryUrl().toHttpUrl() + ">: " + ExceptionUtility.toMessage( e ) );
}
}
}
// /////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////
private Map> loadRegisteredServices( Url aDiscoveryUrl, TrustStoreDescriptor aStoreDescriptor ) throws OpenException, HttpStatusException, MalformedURLException {
Map> theInstances = new HashMap<>();
aDiscoveryUrl = toHttpDiscoveryUrl( aDiscoveryUrl, _discovery );
aStoreDescriptor = toTrustStoreDescriptor( aStoreDescriptor, (TrustStoreDescriptorProperty) _discovery );
HttpRestClient theClient = new HttpRestClientImpl( _executorService );
theClient.open( aStoreDescriptor );
theClient.setBaseUrl( aDiscoveryUrl );
LOGGER.info( "Requesting discoverable services at <" + aDiscoveryUrl.toHttpUrl() + "> Eureka service registry..." );
RestResponse theResponse = theClient.doGet( "/" );
if ( theResponse.getHttpStatusCode().isErrorStatus() ) {
throw theResponse.getHttpStatusCode().toHttpStatusException( "Cannot retrieve any discoverable services with service discovery <" + aDiscoveryUrl.toHttpUrl() + "> due to HTTP-Status-Code " + theResponse.getHttpStatusCode() + "<" + theResponse.getHttpStatusCode().getStatusCode() + ">: " + theResponse.getHttpBody() );
}
HttpBodyMap theHttpBody = theResponse.getResponse();
HttpBodyMap theCloud = theHttpBody.retrieveFrom( theHttpBody.toPath( "applications", "application" ) );
Set theAppsListing = theCloud.dirs();
Set eInstancesListing;
HttpBodyMap eApp;
List eDescriptors;
EurekaInstanceDescriptor eDescriptor;
String eAlias;
for ( String eAppIndex : theAppsListing ) {
eApp = theCloud.retrieveFrom( theCloud.toPath( eAppIndex, "instance" ) );
eInstancesListing = eApp.dirs();
for ( String eInstanceIndex : eInstancesListing ) {
eDescriptor = new EurekaInstanceDescriptorImpl( eApp.retrieveFrom( eInstanceIndex ) );
eAlias = eDescriptor.getAlias();
if ( eAlias != null ) {
eAlias = eAlias.toUpperCase();
}
eDescriptors = theInstances.get( eAlias );
if ( eDescriptors == null ) {
synchronized ( _discovery ) {
eDescriptors = theInstances.get( eAlias );
if ( eDescriptors == null ) {
eDescriptors = new ArrayList<>();
theInstances.put( eAlias, eDescriptors );
}
}
}
eDescriptors.add( eDescriptor );
}
}
return theInstances;
}
private List toInstancesUpdate( List aRemoteInstances, List aLocalInstances ) {
List theInstances = new ArrayList<>( aLocalInstances );
EurekaInstanceDescriptor eLocalInstance;
if ( aRemoteInstances != null ) {
for ( EurekaInstanceDescriptor eRemoteInstance : aRemoteInstances ) {
eLocalInstance = toInstance( eRemoteInstance, theInstances );
if ( eLocalInstance != null ) {
theInstances.remove( eLocalInstance );
}
if ( EurekaServiceStatus.UP == EurekaServiceStatus.toStatus( eRemoteInstance.getStatus() ) ) {
theInstances.add( eRemoteInstance );
}
}
}
return theInstances;
}
private EurekaInstanceDescriptor toInstance( EurekaInstanceDescriptor aInstance, List aInstances ) {
if ( aInstances != null ) {
for ( EurekaInstanceDescriptor eInstance : aInstances ) {
if ( eInstance.getHost().equals( aInstance.getHost() ) ) {
return eInstance;
}
}
}
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy