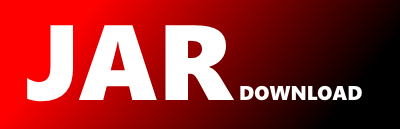
org.refcodes.rest.ext.eureka.EurekaRestServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-rest-ext-eureka Show documentation
Show all versions of refcodes-rest-ext-eureka Show documentation
Artifact for providing Spring Boot's Eureka microservice registry/
discovery support.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.rest.ext.eureka;
import org.refcodes.component.InitializeException;
import org.refcodes.component.LifeCycleComponent;
import org.refcodes.data.Scheme;
import org.refcodes.net.Url;
import org.refcodes.rest.HomeRequestObserver;
import org.refcodes.rest.HomeRequestObserverAccessor;
import org.refcodes.rest.HomeRequestObserverAccessor.HomeRequestObserverBuilder;
import org.refcodes.rest.HttpRegistryRestServer;
import org.refcodes.rest.RestRequestObserver;
import org.refcodes.rest.StatusRequestObserver;
import org.refcodes.rest.StatusRequestObserverAccessor;
import org.refcodes.rest.StatusRequestObserverAccessor.StatusRequestObserverBuilder;
import org.refcodes.security.TrustStoreDescriptor;
/**
* The {@link EurekaRestServer} refines the {@link HttpRegistryRestServer} for
* use with an Eureka Service-Registry. The Lifecycle-States are used to control
* the registry process.
*
*
* - {@link #initialize()}: (or the like
initialize
methods)
* Initialize this service by registering it with the status
* {@link EurekaServiceStatus#STARTING} to the attached registry server.
* - {@link #start()}: Starts this service by registering it with the status
* {@link EurekaServiceStatus#UP} to the attached registry server.
*
- {@link #pause()}: Pauses this service by updating the status
* {@link EurekaServiceStatus#DOWN} to the attached registry server.
*
- {@link #resume()}:
*
- {@link #stop()}: Stops this service by updating the status
* {@link EurekaServiceStatus#OUT_OF_SERVICE} to the attached registry server.
*
- {@link #destroy()}: Removes the server from the registry.
*
*
* The {@link EurekaRestServer} implements the {@link LifeCycleComponent}, the
* above mentioned lifecycle states behave as of the definition for the
* {@link LifeCycleComponent} interface.
*/
public interface EurekaRestServer extends HttpRegistryRestServer, EurekaRegistry, HomeRequestObserver, StatusRequestObserver, StatusRequestObserverAccessor, StatusRequestObserverBuilder, HomeRequestObserverAccessor, HomeRequestObserverBuilder, EurekaServerDescriptorFactory {
/**
* Initializes the {@link HttpRegistryRestServer} by registering it at the
* Eureka service registry with a status such as "starting" or
* "initializing" or "not-ready-yet".
*
* {@inheritDoc}
*
* @param aRegistryContext The context providing the descriptor of the
* server to be registered and the {@link Url} of the service
* registry to be used as well as the required truststore.
*
* @throws InitializeException thrown in case initializing a component
* caused problems. Usually a method similar to "initialize()"
* throws such an exception.
*/
default void initialize( EurekaRegistryContext aRegistryContext ) throws InitializeException {
initialize( aRegistryContext.getHttpServerDescriptor(), aRegistryContext.getPingRequestObserver(), aRegistryContext.getStatusRequestObserver(), aRegistryContext.getHomeRequestObserver(), aRegistryContext.getHttpRegistryUrl(), aRegistryContext.getTrustStoreDescriptor() );
}
/**
*
* Initializes the {@link HttpRegistryRestServer} by registering it at the
* service registry with a status such as "starting" or "initializing" or
* "not-ready-yet".
*
* {@inheritDoc}
*
* @param aAlias The name ("alias") which identifies the server in the
* registry.
* @param aInstanceId The ID for the instance when being registered at the
* service registry. If omitted, then the host name is used.
* @param aScheme The {@link Scheme} to which this server is being attached
* (HTTP or HTTPS).
* @param aHost The host name to be used to address this server. If omitted,
* then the system's host name should be used.
* @param aVirtualHost The virtual host name to be used for resolving.
* @param aIpAddress The IP-Address identifying the host.
* @param aPort The port of your service being registered.
* @param aPingPath The path to use as health-check end-point by this
* server.
* @param aPingRequestObserver The {@link RestRequestObserver} hooking into
* a ping request.
* @param aRegistryUrl The registry server where to register.
* @throws InitializeException thrown in case initializing a component
* caused problems. Usually a method similar to "initialize()"
* throws such an exception.
*/
@Override
default void initialize( String aAlias, String aInstanceId, Scheme aScheme, String aHost, String aVirtualHost, int[] aIpAddress, int aPort, String aPingPath, RestRequestObserver aPingRequestObserver, Url aRegistryUrl ) throws InitializeException {
initialize( aAlias, aInstanceId, aScheme, aHost, aVirtualHost, aIpAddress, aPort, aPingPath, aPingRequestObserver, null, null, null, null, null, aRegistryUrl, null );
}
/**
*
* Initializes the {@link HttpRegistryRestServer} by registering it at the
* service registry.
*
* @param aAlias The name ("alias") which identifies the server in the
* registry.
* @param aInstanceId The ID for the instance when being registered at the
* service registry. If omitted, then the host name is used.
* @param aScheme The {@link Scheme} to which this server is being attached
* (HTTP or HTTPS).
* @param aHost The host name to be used to address this server. If omitted,
* then the system's host name should be used.
* @param aVirtualHost The virtual host name to be used for resolving.
* @param aIpAddress The IP-Address identifying the host.
* @param aPort The port of your service being registered. Make sure, you do
* not
* @param aPingPath The path to use as health-check end-point by this
* server.
* @param aPingRequestObserver The health-check request observer hook.
* @param aStatusPath The path to use as status-page end-point by this
* @param aStatusRequestObserver The status-page request observer hook.
* @param aHomePath The path to use as home-page end-point by this
* @param aHomeRequestObserver The home-page request observer hook.
* @param aDataCenterType The type of the data center your
* {@link EurekaRestServer} is running in.
* @param aRegistryUrl The registry server where to register.
* @param aStoreDescriptor The descriptor describing the truststore for
* (optionally) opening an HTTPS connection to the registry server.
*
* @throws InitializeException thrown in case initializing a component
* caused problems. Usually a method similar to "initialize()"
* throws such an exception.
*/
default void initialize( String aAlias, String aInstanceId, Scheme aScheme, String aHost, String aVirtualHost, int[] aIpAddress, int aPort, String aPingPath, RestRequestObserver aPingRequestObserver, String aStatusPath, RestRequestObserver aStatusRequestObserver, String aHomePath, RestRequestObserver aHomeRequestObserver, EurekaDataCenterType aDataCenterType, Url aRegistryUrl, TrustStoreDescriptor aStoreDescriptor ) throws InitializeException {
initialize( toHttpServerDescriptor( aAlias, aInstanceId, aScheme, aHost, aVirtualHost, aIpAddress, aPort, aPingPath, aStatusPath, aHomePath, aDataCenterType ), aPingRequestObserver, aStatusRequestObserver, aHomeRequestObserver, aRegistryUrl, aStoreDescriptor );
}
/**
* Initializes the {@link HttpRegistryRestServer} by registering it at the
* service registry.
*
* @param aServerDescriptor The descriptor describing the server.
* @param aPingRequestObserver The health-check request observer hook.
* @param aStatusRequestObserver The status-page request observer hook.
* @param aHomeRequestObserver The home-page request observer hook.
* @param aRegistryUrl The registry server where to register.
* @param aStoreDescriptor The descriptor describing the truststore for
* (optionally) opening an HTTPS connection to the registry server.
*
* @throws InitializeException thrown in case initializing a component
* caused problems. Usually a method similar to "initialize()"
* throws such an exception.
*/
void initialize( EurekaServerDescriptor aServerDescriptor, RestRequestObserver aPingRequestObserver, RestRequestObserver aStatusRequestObserver, RestRequestObserver aHomeRequestObserver, Url aRegistryUrl, TrustStoreDescriptor aStoreDescriptor ) throws InitializeException;
/**
* {@inheritDoc}
*/
@Override
default void initialize( EurekaServerDescriptor aServerDescriptor, Url aRegistryUrl, TrustStoreDescriptor aStoreDescriptor ) throws InitializeException {
initialize( aServerDescriptor, null, null, null, aRegistryUrl, aStoreDescriptor );
}
/**
* {@inheritDoc}
*/
@Override
default EurekaRestServer withStatusRequestObserver( RestRequestObserver aRequestObserver ) {
onStatusRequest( aRequestObserver );
return this;
}
/**
* {@inheritDoc}
*/
@Override
default EurekaRestServer withHomeRequestObserver( RestRequestObserver aRequestObserver ) {
onHomeRequest( aRequestObserver );
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy