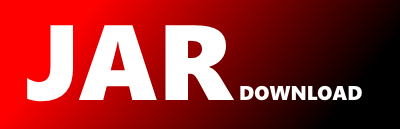
org.refcodes.rest.ext.eureka.EurekaServerDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-rest-ext-eureka Show documentation
Show all versions of refcodes-rest-ext-eureka Show documentation
Artifact for providing Spring Boot's Eureka microservice registry/
discovery support.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.rest.ext.eureka;
import java.net.MalformedURLException;
import java.util.Map;
import org.refcodes.exception.BugException;
import org.refcodes.mixin.AliasAccessor.AliasProperty;
import org.refcodes.mixin.PortAccessor.PortProperty;
import org.refcodes.net.HostAccessor.HostProperty;
import org.refcodes.net.HttpBodyMap;
import org.refcodes.net.IpAddress;
import org.refcodes.net.IpAddressAccessor.IpAddressProperty;
import org.refcodes.net.Url;
import org.refcodes.net.UrlImpl;
import org.refcodes.rest.HomeUrlAccessor.HomeUrlProperty;
import org.refcodes.rest.HttpServerDescriptor;
import org.refcodes.rest.PingUrlAccessor.PingUrlProperty;
import org.refcodes.rest.StatusUrlAccessor.StatusUrlProperty;
import org.refcodes.rest.ext.eureka.AmazonMetaDataAccessor.AmazonMetaDataProperty;
import org.refcodes.rest.ext.eureka.EurekaDataCenterTypeAccessor.EurekaDataCenterTypeProperty;
import org.refcodes.rest.ext.eureka.EurekaServiceStatusAccessor.EurekaServiceStatusProperty;
/**
* The {@link EurekaServerDescriptor} refines the {@link HttpServerDescriptor}
* for use with an Eureka Service-Registry.
*/
public interface EurekaServerDescriptor extends HttpServerDescriptor, AliasProperty, PingUrlProperty, StatusUrlProperty, HomeUrlProperty, HostProperty, IpAddressProperty, PortProperty, HttpBodyMap, EurekaDataCenterTypeProperty, EurekaServiceStatusProperty, AmazonMetaDataProperty {
/**
* Returns the length of lease - default if 90 seconds.
*
* @return The lease eviction duration in seconds.
*/
default Integer getLeaseEvictionDurationInSecs() {
return getInteger( "instance/leaseInfo/evictionDurationInSecs" );
}
/**
* Optional, if you want to change the length of lease - default if 90
* seconds.
*
* @param aLeaseEvictionDurationInSecs The lease eviction duration in
* seconds.
*/
default void setLeaseEvictionDurationInSecs( Integer aLeaseEvictionDurationInSecs ) {
putInteger( "instance/leaseInfo/evictionDurationInSecs", aLeaseEvictionDurationInSecs );
}
/**
* {@inheritDoc}
*/
@Override
default String getAlias() {
return get( "instance/app" );
}
/**
* {@inheritDoc}
*/
@Override
default void setAlias( String aAlias ) {
put( "instance/app", aAlias );
}
/**
* {@inheritDoc}
*/
@Override
default Url getPingUrl() {
String theHealthCheckUrl = get( "instance/healthCheckUrl" );
if ( theHealthCheckUrl == null ) {
return null;
}
try {
return new UrlImpl( theHealthCheckUrl );
}
catch ( MalformedURLException e ) {
throw new BugException( "Cannot parse the Health-Check URL <" + theHealthCheckUrl + ">, please use the according setter to ensure correct URL format: " + e.getMessage(), e );
}
}
/**
* {@inheritDoc}
*/
@Override
default void setPingUrl( Url aUrl ) {
put( "instance/healthCheckUrl", aUrl.toHttpUrl() );
}
/**
* {@inheritDoc}
*/
@Override
default Url getStatusUrl() {
String theStatusPageUrl = get( "instance/statusPageUrl" );
if ( theStatusPageUrl == null ) {
return null;
}
try {
return new UrlImpl( theStatusPageUrl );
}
catch ( MalformedURLException e ) {
throw new BugException( "Cannot parse the Health-Check URL <" + theStatusPageUrl + ">, please use the according setter to ensure correct URL format: " + e.getMessage(), e );
}
}
/**
* {@inheritDoc}
*/
@Override
default void setStatusUrl( Url aUrl ) {
put( "instance/statusPageUrl", aUrl.toHttpUrl() );
}
/**
* {@inheritDoc}
*/
@Override
default Url getHomeUrl() {
String theHomePageUrl = get( "instance/homePageUrl" );
if ( theHomePageUrl == null ) {
return null;
}
try {
return new UrlImpl( theHomePageUrl );
}
catch ( MalformedURLException e ) {
throw new BugException( "Cannot parse the Health-Check URL <" + theHomePageUrl + ">, please use the according setter to ensure correct URL format: " + e.getMessage(), e );
}
}
/**
* {@inheritDoc}
*/
@Override
default void setHomeUrl( Url aUrl ) {
put( "instance/homePageUrl", aUrl.toHttpUrl() );
}
/**
* {@inheritDoc}
*/
@Override
default String getHost() {
return get( "instance/hostName" );
}
/**
* {@inheritDoc}
*/
@Override
default void setHost( String aHost ) {
put( "instance/hostName", aHost );
}
/**
* {@inheritDoc}
*/
@Override
default int getPort() {
return getInteger( "instance/port" );
}
/**
* {@inheritDoc}
*/
@Override
default void setPort( int aPort ) {
putInteger( "instance/port", aPort );
}
/**
* {@inheritDoc}
*/
@Override
default int[] getIpAddress() {
return IpAddress.fromAnyCidrNotation( get( "instance/ipAddr" ) );
}
/**
* {@inheritDoc}
*/
@Override
default void setIpAddress( int[] aIpAddress ) {
put( "instance/ipAddr", IpAddress.toString( aIpAddress ) );
}
/**
* Retrieves the virtual host as of Eureka(?).
*
* @return The virtual host.
*/
default String getVirtualHost() {
return get( "instance/vipAddress" );
}
/**
* Sets the virtual host as of Eureka(?).
*
* @param aVirtualHost The virtual host.
*/
default void setVirtualHost( String aVirtualHost ) {
put( "instance/vipAddress", aVirtualHost );
}
/**
* {@inheritDoc}
*/
@Override
default EurekaDataCenterType getEurekaDataCenterType() {
return EurekaDataCenterType.fromName( get( "instance/dataCenterInfo/name" ) );
}
/**
* {@inheritDoc}
*/
@Override
default void setEurekaDataCenterType( EurekaDataCenterType aDataCenterType ) {
put( "instance/dataCenterInfo/name", aDataCenterType.getName() );
}
/**
* {@inheritDoc}
*/
@Override
default AmazonMetaData getAmazonMetaData() {
return toType( "instance/dataCenterInfo/metadata", AmazonMetaData.class );
}
/**
* {@inheritDoc}
*/
@Override
default void setAmazonMetaData( AmazonMetaData aDataCenterType ) {
insertTo( "instance/dataCenterInfo/metadata", aDataCenterType );
}
/**
* {@inheritDoc}
*/
@Override
default EurekaServiceStatus getEurekaServiceStatus() {
return EurekaServiceStatus.valueOf( get( "instance/status" ) );
}
/**
* {@inheritDoc}
*/
@Override
default void setEurekaServiceStatus( EurekaServiceStatus aServiceStatus ) {
put( "instance/status", aServiceStatus.name() );
}
/**
* Retrieves the application's Meta-Data.
*
* @return The {@link Map} representing the applications's Meta-Data.
*/
default Map getMetaData() {
return retrieveFrom( "instance/metadata" );
}
/**
* Sets the application's Meta-Data.
*
* @param aMetaData The {@link Map} representing the applications's
* Meta-Data.
*/
default void setMetaData( Map aMetaData ) {
for ( String eKey : aMetaData.keySet() ) {
put( toPath( "instance/metadata", eKey ), aMetaData.get( eKey ) );
}
}
/**
* Adds a key-value pair to the application's Meta-Data.
*
* @param aKey The Key of the Meta-Data entry.
* @param aValue The value for the Meta-Data entry.
*
* @return The previously set value for the given Meta-Data entry or null if
* none was set before.
*/
default String putMetaData( String aKey, String aValue ) {
return put( toPath( "instance/metadata", aKey ), aValue );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy