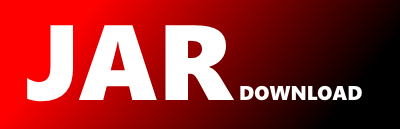
org.refcodes.security.alt.chaos.ChaosOptionsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-security-alt-chaos Show documentation
Show all versions of refcodes-security-alt-chaos Show documentation
Artifact for providing chaos symmetric encryption.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.security.alt.chaos;
import org.refcodes.numerical.NumericalUtility;
/**
* The {@link ChaosOptionsImpl} provides means to create a {@link ChaosOptions}
* instance.
*/
public class ChaosOptionsImpl implements ChaosOptions {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private boolean _isXorNext;
private boolean _isMutateS;
private boolean _isSalted;
private short _rndPrefixSize;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates the {@link ChaosOptions} by reversing the result of the
* {@link #getEncoded()} operation where the byte array contains the values
* of the options. The values use a big endian representation. The byte
* array being passed is to be of the size as returned by
* {@link ChaosOptions#getEncodedLength()}. The meaning of the bits in the
* encoded byte are defined as in {@link #OPTIONS_BIT_MUTATE_S},
* {@link #OPTIONS_BIT_XOR_NEXT} and {@link #OPTIONS_BIT_SALTED}.
*
* @param aEncoded The encoded representation of the options.
*/
public ChaosOptionsImpl( byte[] aEncoded ) {
if ( !NumericalUtility.isBitSetAt( aEncoded[0], OPTIONS_BIT_RND_PREFIX ) && Byte.toUnsignedInt( aEncoded[1] ) != 0 ) {
throw new IllegalArgumentException( "The PREFIX option has been set to false though the prefix size is set to <" + Byte.toUnsignedInt( aEncoded[1] ) + "> which is ambigous!" );
}
_isMutateS = NumericalUtility.isBitSetAt( aEncoded[0], OPTIONS_BIT_MUTATE_S );
_isXorNext = NumericalUtility.isBitSetAt( aEncoded[0], OPTIONS_BIT_XOR_NEXT );
_isSalted = NumericalUtility.isBitSetAt( aEncoded[0], OPTIONS_BIT_SALTED );
_rndPrefixSize = NumericalUtility.isBitSetAt( aEncoded[0], OPTIONS_BIT_RND_PREFIX ) ? (short) ( Byte.toUnsignedInt( aEncoded[1] ) + 1 ) : 0;
}
/**
* Constructs the {@link ChaosOptionsImpl} with the given values.
*
* @param isMutateS Enables or disables the {@link #isMutateS()} option.
* @param isXorNext Enables or disables the {@link #isXorNext()} option.
* @param isSalted Enables or disables the {@link #isSalted()} option.
*/
public ChaosOptionsImpl( boolean isMutateS, boolean isXorNext, boolean isSalted ) {
_isMutateS = isMutateS;
_isXorNext = isXorNext;
_isSalted = isSalted;
_rndPrefixSize = 0;
}
/**
* Constructs the {@link ChaosOptionsImpl} with the given values.
*
* @param isMutateS Enables or disables the {@link #isMutateS()} option.
* @param isXorNext Enables or disables the {@link #isXorNext()} option.
* @param isSalted Enables or disables the {@link #isSalted()} option.
* @param aRndPrefixSize The number of bytes to prefix with random bytes.
*/
public ChaosOptionsImpl( boolean isMutateS, boolean isXorNext, boolean isSalted, short aRndPrefixSize ) {
_isMutateS = isMutateS;
_isXorNext = isXorNext;
_isSalted = isSalted;
_rndPrefixSize = aRndPrefixSize;
}
private ChaosOptionsImpl( Builder builder ) {
_isMutateS = builder.isMutateS;
_isXorNext = builder.isXorNext;
_isSalted = builder.isSalted;
_rndPrefixSize = builder.rndPrefixSize;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public boolean isXorNext() {
return _isXorNext;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isMutateS() {
return _isMutateS;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isSalted() {
return _isSalted;
}
/**
* {@inheritDoc}
*/
@Override
public short getRndPrefixSize() {
return _rndPrefixSize;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getSimpleName() + " [isMutateS=" + _isMutateS + ", isXorNext=" + _isXorNext + ", ( isSalted=" + _isSalted + " | hasRndPrefix=" + hasRndPrefix() + " [rndPrefixSize=" + _rndPrefixSize + "]) := isFixedLength=" + isFixedLength() + "]";
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ( _isMutateS ? 1231 : 1237 );
result = prime * result + ( _isSalted ? 1231 : 1237 );
result = prime * result + ( _isXorNext ? 1231 : 1237 );
result = prime * result + _rndPrefixSize;
return result;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) {
return true;
}
if ( obj == null ) {
return false;
}
if ( !( obj instanceof ChaosOptions ) ) {
return false;
}
final ChaosOptions other = (ChaosOptions) obj;
if ( _isMutateS != other.isMutateS() ) {
return false;
}
if ( _isSalted != other.isSalted() ) {
return false;
}
if ( _isXorNext != other.isXorNext() ) {
return false;
}
if ( _rndPrefixSize != other.getRndPrefixSize() ) {
return false;
}
return true;
}
/**
* Creates builder to build {@link ChaosOptionsImpl}.
*
* @return created builder
*/
public static Builder builder() {
return new Builder();
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* Builder to build {@link ChaosOptions} instances.
*/
public static final class Builder {
private boolean isXorNext;
private boolean isMutateS;
private boolean isSalted;
public short rndPrefixSize;
private Builder() {}
/**
* Enables or disables the {@link #isXorNext()} option.
*
* @param isXorNext True in case XOR obfuscation is to be applied.
*
* @return This builder as of the builder pattern.
*/
public Builder withXorNext( boolean isXorNext ) {
this.isXorNext = isXorNext;
return this;
}
/**
* Enables or disables the {@link #isMutateS()} option.
*
* @param isMutateS True in case MUTATION obfuscation is to be applied.
*
* @return This builder as of the builder pattern.
*/
public Builder withMutateS( boolean isMutateS ) {
this.isMutateS = isMutateS;
return this;
}
/**
* Enables or disables the {@link #isSalted()} option.
*
* @param isSalted True in case MUTATION obfuscation is to be applied.
*
* @return This builder as of the builder pattern.
*/
public Builder withSalted( boolean isSalted ) {
this.isSalted = isSalted;
return this;
}
/**
* Sets the prefix size for the {@link #getRndPrefixSize} option. Valid
* values range from {1..256} representable by one byte as of
* {0x00..0xFF}.
*
* @param aRndPrefixSize The size of the random bytes in case the PREFIX
* option is to be applied.
*
* @return This builder as of the builder pattern.
*/
public Builder withRndPrefixSize( short aRndPrefixSize ) {
if ( aRndPrefixSize < 1 || aRndPrefixSize > 256 ) {
throw new IllegalArgumentException( "The RND prefix size <" + aRndPrefixSize + "> is out of range, valid values range from {1..256} representable by one byte as of {0x00..0xFF}." );
}
this.rndPrefixSize = aRndPrefixSize;
return this;
}
/**
* Determines whether an ADD operation of the {@link ChaosKey}'s S value
* is to be applied with the current byte value (using signed bytes -128
* to +127).
*
* @return the chaos metrics builder
*/
public ChaosOptionsImpl build() {
return new ChaosOptionsImpl( this );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy