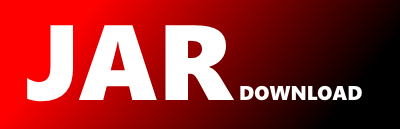
org.refcodes.security.ext.spring.TextDecrypterBean Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.security.ext.spring;
import org.springframework.beans.factory.FactoryBean;
/**
* The sole purpose of the bean is not to store any passwords in clear text in a
* text file. It decrypts any encrypted passwords with a hard coded secret
* (which is depended on the current application and the host this application
* is processed on and may be acquired by accessing and manipulating alongside
* executing the application on the host or heuristically simulating the
* application with metrics gathered from the host). This means that any hacker
* having access to the host and decompiling the code will be able to gain
* knowledge on how to create plain text from the encrypted passwords in a
* configuration file. Additional measures have to be undertaken in order to
* restrict access to the program codes or configuration files by any intruder.
* The password itself is stored as a byte array, so it is not stored as clear
* text in the class files neither. Any further obfuscation has not been
* approached as in the end it will not provide any further security due to the
* fact that the password must be accessible somehow and automatically be
* decryptable by program code.
*
* ATTENTION: This bean just provides support for not storing any passwords in
* clear text!
*/
public class TextDecrypterBean implements FactoryBean {
private String encryptedText;
/**
* Sets the encrypted string to be decrypted and passed the the consuming
* bean.
*
* @param aEncryptedText the encrypted string to be decrypted.
*/
public void setEncryptedText( String aEncryptedText ) {
this.encryptedText = aEncryptedText;
}
/**
* {@inheritDoc}
*/
@Override
public String getObject() {
return TextObfuscaterUtility.toDecryptedText( this.encryptedText );
}
/**
* {@inheritDoc}
*/
@Override
public Class> getObjectType() {
return String.class;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isSingleton() {
return false;
}
// -------------------------------------------------------------------------
// HELPER:
// -------------------------------------------------------------------------
/**
* Helper method to encrypt a string which can be used in a configuration
* file.
*
* @param aText the text string to be encrypted.
*
* @return the string
*/
public static String toEncryptedText( String aText ) {
return TextObfuscaterUtility.toEncryptedText( aText );
}
}