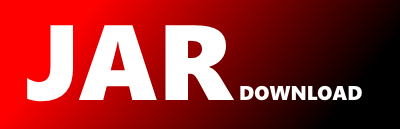
org.refcodes.security.ext.spring.TextObfuscaterUtility Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.security.ext.spring;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.jasypt.util.text.BasicTextEncryptor;
import org.refcodes.data.Prefix;
import org.refcodes.runtime.SystemContext;
/**
* The {@link TextObfuscaterUtility} is a mere in memory text obfuscater (as a
* dynamically determined {@link SystemContext#HOST_APPLICATION} password is
* used (which is depended on the current application and the host this
* application is processed on and may be acquired by accessing and manipulating
* alongside executing the application on the host or heuristically simulating
* the application with metrics gathered from the host).
*/
public final class TextObfuscaterUtility {
public static final int CIPHER_UID_TIMESTAMP_LENGTH = 14;
public static final int CIPHER_UID_LENGTH = 24;
public static final int CIPHER_LENGTH = 48;
public static final int MESSAGE_LENGTH = 256;
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static Logger LOGGER = Logger.getLogger( TextObfuscaterUtility.class.getName() );
private static BasicTextEncryptor TEXT_ENCRYPTOR;
static {
TEXT_ENCRYPTOR = new BasicTextEncryptor();
TEXT_ENCRYPTOR.setPasswordCharArray( SystemContext.HOST_APPLICATION.toContextSequence() );
}
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Private empty constructor to prevent instantiation as of being a utility
* with just static public methods.
*/
private TextObfuscaterUtility() {}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CAMOUFLAGE
// /////////////////////////////////////////////////////////////////////////
// /////////////////////////////////////////////////////////////////////////
// CAMOUFLAGE
// /////////////////////////////////////////////////////////////////////////
/**
* Decrypt the given text in case it has the prefix identifying the text as
* being encrypted, else the plain text is returned and a warning is printed
* out that the passed text is not encrypted (just the first two or three
* chars or zero chars are shown depending on the length of the text).
* -------------------------------------------------------------------------
* Attention: A unique system TID is used for decryption. Decryption with
* this method will only work on the same system used for encryption via
* {@link #toEncryptedText(String)}!
* -------------------------------------------------------------------------
* This method is most useful for encrypting passwords or authentication
* credentials in configuration files on live servers to provide at least a
* minimum of security.
*
* @param aText The text being decrypted.
*
* @return The decrypted text.
*/
public static String toDecryptedText( String aText ) {
if ( aText.toLowerCase().startsWith( Prefix.ENCRYPTED.getPrefix() ) ) {
String theText = aText.substring( Prefix.ENCRYPTED.getPrefix().length() );
return TEXT_ENCRYPTOR.decrypt( theText );
}
String theHint = "";
if ( aText.length() > 12 ) {
theHint = "and starting with first three characters \"" + aText.substring( 0, 3 ) + "...\" ";
}
LOGGER.log( Level.WARNING, "A text with length <" + aText.length() + "> " + theHint + "has been provided which is not encrypted. An encrypted text must start with \"" + Prefix.ENCRYPTED.getPrefix() + "\"!" );
return aText;
}
/**
* Decrypt the given text in case it has the prefix identifying the text as
* being encrypted, else the plain text is returned and a warning is printed
* out that the passed text is not encrypted (just the first two or three
* chars or zero chars are shown depending on the length of the text).
*
* @param aText The text being decrypted.
* @param aPassPharse The pass phrase for decrypting.
*
* @return The decrypt
*/
// public static String toDecryptedText( String aText, String aPassPharse ) {
// if ( aText.toLowerCase().startsWith( Prefix.ENCRYPTED.getPrefix() ) ) {
// BasicTextEncryptor theTextEncryptor = new BasicTextEncryptor();
// theTextEncryptor.setPassword( aPassPharse );
// String theText = aText.substring( Prefix.ENCRYPTED.getPrefix().length() );
// return theTextEncryptor.decrypt( theText );
// }
//
// String theHint = "";
// if ( aText.length() > 12 ) {
// theHint = "and starting with first three characters \"" + aText.substring( 0,
// 3 ) + "...\" ";
// }
// new TextObfuscaterUtility().warn( "A text with length <" + aText.length() +
// "> " + theHint + "has been provided which is not encrypted. An encrypted text
// must start with \"" + Prefix.ENCRYPTED.getPrefix() + "\"!" );
// return aText;
// }
/**
* Encrypts the given text and prepends a prefix identifying the text as
* being encrypted.
* -------------------------------------------------------------------------
* Attention: A unique system TID is used for encryption. Decryption with
* the {@link #toDecryptedText(String)} method will only work on the same
* system used for encryption via this method!
* -------------------------------------------------------------------------
* This method is most useful for encrypting passwords or authentication
* credentials in configuration files on live servers to provide at least a
* minimum of security.
*
* @param aText The text to be encrypted.
*
* @return The encrypted text with the encryption identifier prefixed.
*/
public static String toEncryptedText( String aText ) {
return Prefix.ENCRYPTED.getPrefix() + TEXT_ENCRYPTOR.encrypt( aText );
}
/**
* Encrypts the given text and prepends a prefix identifying the text as
* being encrypted.
*
* @param aText The text to be encrypted.
* @param aPassPhrase The pass phrase for the encrypted text.
*
* @return The encrypted text with the encryption identifier prefixed.
*/
// public static String toEncryptedText( String aText, String aPassPhrase ) {
// BasicTextEncryptor theTextEncryptor = new BasicTextEncryptor();
// theTextEncryptor.setPassword( aPassPhrase );
// return Prefix.ENCRYPTED.getPrefix() + theTextEncryptor.encrypt( aText );
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy