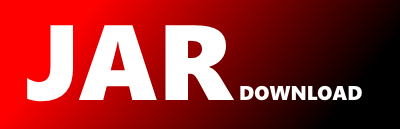
org.refcodes.security.Decrypter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-security Show documentation
Show all versions of refcodes-security Show documentation
Artifact with commonly used security functionality such as message
digester and provider for the java.security framework. The
"refcodes-forwardsecrecy" framework settels on top of the
"refcodes-security" artifact.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.security;
import javax.crypto.CipherSpi;
import org.refcodes.mixin.Disposable;
/**
* Plain interface for providing straight forward decryption functionality as of
* {@link #toDecrypted(Object)} and for forcing your plain functionality to
* provide a bridge to the Java Cryptographic Extension (JCE) framework's
* {@link CipherSpi} as of {@link #toDecrypted(byte[], int, int, byte[], int)}.
* This way you can use your algorithms outside the JCE framework. This may be
* necessary when your Java's security settings prevent running your own JCE
* extensions from inside an (Oracle-) unsigned JAR.
*
* @param The type of the decrypted data.
* @param The type of the encrypted data.
* @param The specific type of the {@link DecryptionException} being
* thrown.
*/
public interface Decrypter extends Disposable {
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* You pass in data of a given type and you get decrypted data of (another)
* given type.
*
* @param aInput The input data to be decrypted.
*
* @return The decrypted output data.
*
* @throws EXC Thrown in case something went wrong upon decryption.
*/
DEC toDecrypted( ENC aInput ) throws EXC;
/**
* For compatibility with the java.security framework,
* ehttps://www.metacodes.proly to be integrated in a sub-class of the
* {@link CipherSpi}.
*
* @param aBuffer The input to be decrypted.
* @param aOffset The offset to start decryption.
* @param aLength The length to be decrypted
* @param aOutBuffer The output where to decrypt to.
* @param aOutOffset The offset where to start writing the decrypted output.
*
* @return The number of bytes decrypted.
*
* @throws DecryptionException Thrown in case a decryption issue occurred
* regarding the {@link Decrypter}. Probably the configuration of
* your {@link Encrypter} does not fit the one of the
* {@link Decrypter}.
* @throws ArrayIndexOutOfBoundsException In case you provided lengths and
* offsets not fitting with the provided arrays.
*/
int toDecrypted( byte[] aBuffer, int aOffset, int aLength, byte[] aOutBuffer, int aOutOffset ) throws DecryptionException;
/**
* This method decrypts the provided buffer beginning at the given offset
* and the given number of bytes.
*
* @param aBuffer The input to be decrypted.
* @param aOffset The offset to start decryption.
* @param aLength The length to be decrypted
*
* @return The number of bytes decrypted.
*
* @throws DecryptionException Thrown in case a decryption issue occurred
* regarding the {@link Decrypter}. Probably the configuration of
* your {@link Encrypter} does not fit the one of the
* {@link Decrypter}.
* @throws ArrayIndexOutOfBoundsException In case you provided lengths and
* offsets not fitting with the provided arrays.
*/
default int decrypt( byte[] aBuffer, int aOffset, int aLength ) throws DecryptionException {
return toDecrypted( aBuffer, aOffset, aLength, aBuffer, aOffset );
}
/**
* This method decrypts the provided buffer.
*
* @param aBuffer The input to be decrypted.
*
* @return The number of bytes decrypted.
*
* @throws DecryptionException Thrown in case a decryption issue occurred
* regarding the {@link Decrypter}. Probably the configuration of
* your {@link Encrypter} does not fit the one of the
* {@link Decrypter}.
* @throws ArrayIndexOutOfBoundsException In case you provided lengths and
* offsets not fitting with the provided arrays.
*/
default int decrypt( byte[] aBuffer ) throws DecryptionException {
return toDecrypted( aBuffer, 0, aBuffer.length, aBuffer, 0 );
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy