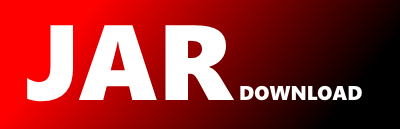
org.refcodes.serial.ext.handshake.SerialHandshakeSugar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial-ext-handshake Show documentation
Show all versions of refcodes-serial-ext-handshake Show documentation
The refcodes-serial-ext-handshake artifact extends the refcodes-serial artifact
with handshake functionality.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial.ext.handshake;
import java.util.concurrent.ExecutorService;
import org.refcodes.numerical.ChecksumValidationMode;
import org.refcodes.numerical.CrcAlgorithm;
import org.refcodes.numerical.Endianess;
import org.refcodes.serial.Port;
import org.refcodes.serial.PortMetrics;
import org.refcodes.serial.Section;
import org.refcodes.serial.Segment;
import org.refcodes.serial.TransmissionMetrics;
/**
* Declarative syntactic sugar which may be statically imported in order to
* allow declarative definitions for the construction of various serial types
* such as {@link Segment} or {@link Section} type instances (and the like).
*/
public class SerialHandshakeSugar {
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new serial sugar.
*/
protected SerialHandshakeSugar() {}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
// ------------------------------------------------------------------------
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort ) {
return new HandshakePortController<>( aPort );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aTransmissionMagicBytes The magic bytes identifying a regular
* transmission (as of
* {@link HandshakePortController#transmitSegment(Segment)} or the
* like).
* @param aNoAcknowledgeMagicBytes the No-Acknowledge magic bytes
* @param aRequestForAcknowledgeMagicBytes the Request-for-Acknowledge magic
* bytes
* @param aAcknowledgeMagicBytes The ACK character(s) to be used by the
* return channel to transmit an ACK (acknowledge) response after
* successful receiving a transmission.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, byte[] aTransmissionMagicBytes, byte[] aNoAcknowledgeMagicBytes, byte[] aRequestForAcknowledgeMagicBytes, byte[] aAcknowledgeMagicBytes, CrcAlgorithm aCrcAlgorithm ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aTransmissionMagicBytes The magic bytes identifying a regular
* transmission (as of
* {@link HandshakePortController#transmitSegment(Segment)} or the
* like).
* @param aNoAcknowledgeMagicBytes the No-Acknowledge magic bytes
* @param aRequestForAcknowledgeMagicBytes the Request-for-Acknowledge magic
* bytes
* @param aAcknowledgeMagicBytes The ACK character(s) to be used by the
* return channel to transmit an ACK (acknowledge) response after
* successful receiving a transmission.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, byte[] aTransmissionMagicBytes, byte[] aNoAcknowledgeMagicBytes, byte[] aRequestForAcknowledgeMagicBytes, byte[] aAcknowledgeMagicBytes, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm, aChecksumValidationMode );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aTransmissionMagicBytes The magic bytes identifying a regular
* transmission (as of
* {@link HandshakePortController#transmitSegment(Segment)} or the
* like).
* @param aNoAcknowledgeMagicBytes the No-Acknowledge magic bytes
* @param aRequestForAcknowledgeMagicBytes the Request-for-Acknowledge magic
* bytes
* @param aAcknowledgeMagicBytes The ACK character(s) to be used by the
* return channel to transmit an ACK (acknowledge) response after
* successful receiving a transmission.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
* @param aExecutorService The {@link ExecutorService} to be used when
* creating {@link Thread} instances for handling input and output
* data simultaneously.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, byte[] aTransmissionMagicBytes, byte[] aNoAcknowledgeMagicBytes, byte[] aRequestForAcknowledgeMagicBytes, byte[] aAcknowledgeMagicBytes, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode, ExecutorService aExecutorService ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm, aChecksumValidationMode );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aTransmissionMagicBytes The magic bytes identifying a regular
* transmission (as of
* {@link HandshakePortController#transmitSegment(Segment)} or the
* like).
* @param aNoAcknowledgeMagicBytes the No-Acknowledge magic bytes
* @param aRequestForAcknowledgeMagicBytes the Request-for-Acknowledge magic
* bytes
* @param aAcknowledgeMagicBytes The ACK character(s) to be used by the
* return channel to transmit an ACK (acknowledge) response after
* successful receiving a transmission.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aExecutorService The {@link ExecutorService} to be used when
* creating {@link Thread} instances for handling input and output
* data simultaneously.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, byte[] aTransmissionMagicBytes, byte[] aNoAcknowledgeMagicBytes, byte[] aRequestForAcknowledgeMagicBytes, byte[] aAcknowledgeMagicBytes, CrcAlgorithm aCrcAlgorithm, ExecutorService aExecutorService ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm, aExecutorService );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, HandshakeTransmissionMetrics aTransmissionMetrics ) {
return new HandshakePortController<>( aPort, aTransmissionMetrics );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aExecutorService The {@link ExecutorService} to be used when
* creating {@link Thread} instances for handling input and output
* data simultaneously.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, HandshakeTransmissionMetrics aTransmissionMetrics, ExecutorService aExecutorService ) {
return new HandshakePortController<>( aPort, aTransmissionMetrics, aExecutorService );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, CrcAlgorithm aCrcAlgorithm ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm, aChecksumValidationMode );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
* @param aExecutorService The {@link ExecutorService} to be used when
* creating {@link Thread} instances for handling input and output
* data simultaneously.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode, ExecutorService aExecutorService ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm, aChecksumValidationMode, aExecutorService );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality as of the given arguments.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aExecutorService The {@link ExecutorService} to be used when
* creating {@link Thread} instances for handling input and output
* data simultaneously.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, Endianess aEndianess, CrcAlgorithm aCrcAlgorithm, ExecutorService aExecutorService ) {
return new HandshakePortController<>( aPort, aEndianess, aCrcAlgorithm, aExecutorService );
}
/**
* Decorates the given {@link Port} with full duplex {@link Segment}
* multiplexer functionality.
*
* @param The actual {@link PortMetrics} type to use.
* @param aPort The {@link Port} to be decorated.
* @param aExecutorService The {@link ExecutorService} to be used when
* creating {@link Thread} instances for handling input and output
* data simultaneously.
*
* @return The accordingly created {@link HandshakePortController}.
*/
public static HandshakePortController handshakePortController( Port aPort, ExecutorService aExecutorService ) {
return new HandshakePortController<>( aPort, aExecutorService );
}
/**
* Constructs a builder to build an according
* {@link HandshakePortController} instance.
*
* @param the generic type
*
* @return The accordingly created {@link HandshakePortController.Builder}.
*/
public static HandshakePortController.Builder handshakePortControllerBuilder() {
return HandshakePortController.builder();
}
// -------------------------------------------------------------------------
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy