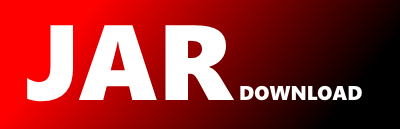
org.refcodes.serial.AbstractReferenceeLengthSegment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Objects;
import org.refcodes.numerical.Endianess;
import org.refcodes.numerical.EndianessAccessor;
import org.refcodes.serial.Transmission.TransmissionMixin;
import org.refcodes.struct.SimpleTypeMap;
import org.refcodes.struct.SimpleTypeMapImpl;
/**
* The {@link AbstractReferenceeLengthSegment} represents a allocated length
* value as of the referenced {@link Transmission} element's length.
*
* @param the generic type
*/
public abstract class AbstractReferenceeLengthSegment implements Segment, TransmissionMixin, LengthWidthAccessor, EndianessAccessor, AllocLengthAccessor {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
public static final String ALLOC_LENGTH = "ALLOC_LENGTH";
public static final String ALLOC_LENGTH_WIDTH = "ALLOC_LENGTH_WIDTH";
public static final String ENDIANESS = "ENDIANESS";
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
protected Endianess _endianess;
protected int _lengthWidth;
protected int _allocLength = -1;
protected REFERENCEE _referencee = null; // Either referencee or decoratee!
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs an according instance. The configuration attributes are taken
* from the {@link TransmissionMetrics} configuration object, though only
* those attributes are supported which are also supported by the other
* constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public AbstractReferenceeLengthSegment( TransmissionMetrics aTransmissionMetrics ) {
this( aTransmissionMetrics.getLengthWidth(), aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance. The configuration attributes are taken
* from the {@link TransmissionMetrics} configuration object, though only
* those attributes are supported which are also supported by the other
* constructors!
*
* @param aReferencee The referenced {@link Transmission} which's length is
* to be used.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public AbstractReferenceeLengthSegment( REFERENCEE aReferencee, TransmissionMetrics aTransmissionMetrics ) {
this( aReferencee, aTransmissionMetrics.getLengthWidth(), aTransmissionMetrics.getEndianess() );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty allocation value with a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the decoratee's length and a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the decoratee's length.
*/
public AbstractReferenceeLengthSegment() {
this( TransmissionMetrics.DEFAULT_LENGTH_WIDTH, TransmissionMetrics.DEFAULT_ENDIANESS );
}
/**
* Constructs an empty allocation value with a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the decoratee's length and the provided {@link Endianess} representation
* of the decoratee's length.
*
* @param aEndianess The {@link Endianess} to be used for length values.
*/
public AbstractReferenceeLengthSegment( Endianess aEndianess ) {
this( TransmissionMetrics.DEFAULT_LENGTH_WIDTH, aEndianess );
}
/**
* Constructs an empty allocation value with the given number of bytes used
* to specify the decoratee's length and a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the decoratee's length.
*
* @param aLengthWidth The width (in bytes) to be used for length values.
*/
public AbstractReferenceeLengthSegment( int aLengthWidth ) {
this( aLengthWidth, TransmissionMetrics.DEFAULT_ENDIANESS );
}
/**
* Constructs an empty allocation value with the given number of bytes used
* to specify the decoratee's length and the provided {@link Endianess}
* representation of the decoratee's length.
*
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for length values.
*/
public AbstractReferenceeLengthSegment( int aLengthWidth, Endianess aEndianess ) {
this( null, aLengthWidth, aEndianess );
}
/**
* Constructs the allocation value with the given decoratee and a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the decoratee's length a {@link TransmissionMetrics#DEFAULT_ENDIANESS}
* endian representation of the decoratee's length.
*
* @param aReferencee The decoratee used for this allocation value.
*/
public AbstractReferenceeLengthSegment( REFERENCEE aReferencee ) {
this( aReferencee, TransmissionMetrics.DEFAULT_LENGTH_WIDTH, TransmissionMetrics.DEFAULT_ENDIANESS );
}
/**
* Constructs the allocation value with the given decoratee and a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the decoratee's length and the provided {@link Endianess} representation
* of the decoratee's length.
*
* @param aReferencee The decoratee used for this allocation value.
* @param aEndianess The {@link Endianess} to be used for length values.
*/
public AbstractReferenceeLengthSegment( REFERENCEE aReferencee, Endianess aEndianess ) {
this( aReferencee, TransmissionMetrics.DEFAULT_LENGTH_WIDTH, aEndianess );
}
/**
* Constructs the allocation value with the given decoratee and with the
* given number of bytes used to specify the decoratee's length and a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the decoratee's length.
*
* @param aReferencee The decoratee used for this allocation value.
* @param aLengthWidth The width (in bytes) to be used for length values.
*/
public AbstractReferenceeLengthSegment( REFERENCEE aReferencee, int aLengthWidth ) {
this( aReferencee, aLengthWidth, TransmissionMetrics.DEFAULT_ENDIANESS );
}
/**
* Constructs the allocation value with the given decoratee and with the
* given number of bytes used to specify the decoratee's length and the
* provided {@link Endianess} representation of the decoratee's length.
*
* @param aReferencee The decoratee used for this allocation value.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for length values.
*/
public AbstractReferenceeLengthSegment( REFERENCEE aReferencee, int aLengthWidth, Endianess aEndianess ) {
_endianess = aEndianess;
_lengthWidth = aLengthWidth;
_referencee = aReferencee;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public int getLengthWidth() {
return _lengthWidth;
}
/**
* {@inheritDoc}
*/
@Override
public Sequence toSequence() {
final int theAllocLength = _allocLength != -1 ? _allocLength : _referencee != null ? _referencee.getLength() : 0;
if ( _allocLength == -1 ) {
_allocLength = theAllocLength;
}
return new ByteArraySequence( _endianess.toBytes( theAllocLength, _lengthWidth ) );
}
/**
* {@inheritDoc}
*/
@Override
public int fromTransmission( Sequence aSequence, int aOffset ) throws TransmissionException {
final byte[] theBuffer = aSequence.toBytes( aOffset, _lengthWidth );
_allocLength = _endianess.toUnsignedInteger( theBuffer );
return aOffset + _lengthWidth;
}
/**
* {@inheritDoc}
*/
@Override
public void receiveFrom( InputStream aInputStream, OutputStream aReturnStream ) throws IOException {
final byte[] theBuffer = new byte[_lengthWidth];
aInputStream.read( theBuffer, 0, _lengthWidth );
_allocLength = _endianess.toUnsignedInteger( theBuffer );
}
/**
* {@inheritDoc}
*/
@Override
public int getLength() {
return _lengthWidth;
}
/**
* {@inheritDoc}
*/
@Override
public Endianess getEndianess() {
return _endianess;
}
/**
* Returns the allocated length in bytes declared by this instance.
*
* @return The allocated length in bytes.
*/
@Override
public int getAllocLength() {
return _allocLength;
}
/**
* Provides means to manipulate the length of a transmission in terms of
* {@link #toTransmission()} and
* {@link #transmitTo(OutputStream, InputStream)} (or the like sending
* operations).
*
* CAUTION: The provided allocation length gets overwritten as soon as a
* transmission is being received in terms of
* {@link #fromTransmission(Sequence)} (or the like receiving operations)!
*
* This method is meant to manipulate the number of bytes to be sent from a
* buffer (a buffer such as a {@link SequenceSection} might reserve a given
* number of bytes to be manipulated, though not all of them are to be
* sent).
*
* @return the schema
*/
// @Override
// public void setAllocLength( int aAllocLength ) {
// _allocLength = aAllocLength;
// }
/**
* {@inheritDoc}
*/
@Override
public void reset() {
_referencee.reset();
}
/**
* {@inheritDoc}
*/
@Override
public SerialSchema toSchema() {
final SerialSchema theSchema = new SerialSchema( getClass(), toSequence(), Integer.toString( getAllocLength() ), "An allocation value referencing a segment which's length is to be allocated and allocating that length in bytes.", getLength() );
theSchema.put( ENDIANESS, _endianess );
theSchema.put( ALLOC_LENGTH_WIDTH, _lengthWidth );
theSchema.put( ALLOC_LENGTH, _allocLength );
return theSchema;
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
return Objects.hash( _referencee, _endianess, _lengthWidth, _allocLength );
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) {
return true;
}
if ( !( obj instanceof AbstractReferenceeLengthSegment ) ) {
return false;
}
final AbstractReferenceeLengthSegment> other = (AbstractReferenceeLengthSegment>) obj;
return Objects.equals( _referencee, other._referencee ) && _endianess == other._endianess && _lengthWidth == other._lengthWidth && _allocLength == other._allocLength;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getSimpleName() + " [endianess=" + _endianess + ", lengthWidth=" + _lengthWidth + ", value=" + _allocLength + "]";
}
/**
* {@inheritDoc}
*/
@Override
public SimpleTypeMap toSimpleTypeMap() {
return _referencee != null ? _referencee.toSimpleTypeMap() : new SimpleTypeMapImpl();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy