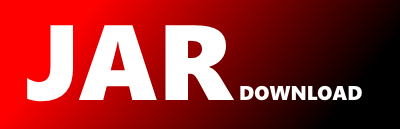
org.refcodes.serial.CharSection Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.nio.charset.Charset;
import org.refcodes.mixin.EncodingAccessor;
import org.refcodes.textual.CaseStyleBuilder;
/**
* The {@link CharSection} is an implementation of a {@link Section} carrying a
* char value as payload.
*/
public class CharSection extends AbstractPayloadSection implements Section, EncodingAccessor {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _charset = TransmissionMetrics.DEFAULT_ENCODING.name();
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public CharSection( TransmissionMetrics aTransmissionMetrics ) {
this( aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public CharSection( char aValue, TransmissionMetrics aTransmissionMetrics ) {
this( aValue, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public CharSection( Character aValue, TransmissionMetrics aTransmissionMetrics ) {
this( aValue, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public CharSection( String aAlias, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public CharSection( String aAlias, char aValue, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, aValue, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public CharSection( String aAlias, Character aValue, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, aValue, aTransmissionMetrics.getEncoding() );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link CharSection}.
*/
public CharSection() {
this( CaseStyleBuilder.asCamelCase( CharSection.class.getSimpleName() ) );
}
/**
* Constructs an empty {@link CharSection} with the given {@link Charset}.
*
* @param aCharset The charset to be used for encoding.
*/
public CharSection( Charset aCharset ) {
this( CaseStyleBuilder.asCamelCase( CharSection.class.getSimpleName() ), aCharset );
}
/**
* Constructs a {@link CharSection} with the given char payload.
*
* @param aPayload The payload to be contained by the {@link Section}.
*/
public CharSection( Character aPayload ) {
this( CaseStyleBuilder.asCamelCase( CharSection.class.getSimpleName() ), aPayload );
}
/**
* Constructs a {@link CharSection} with the given char payload and the
* given {@link Charset}.
*
* @param aPayload The payload to be contained by the {@link Section}.
* @param aCharset The charset to be used for encoding.
*/
public CharSection( Character aPayload, Charset aCharset ) {
this( CaseStyleBuilder.asCamelCase( CharSection.class.getSimpleName() ), aPayload, aCharset );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link CharSection}.
*
* @param aAlias The alias which identifies the content of this segment.
*/
public CharSection( String aAlias ) {
this( aAlias, (char) 0, TransmissionMetrics.DEFAULT_ENCODING );
}
/**
* Constructs an empty {@link CharSection} with the given {@link Charset}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aCharset The charset to be used for encoding.
*/
public CharSection( String aAlias, Charset aCharset ) {
this( aAlias, (char) 0, aCharset );
}
/**
* Constructs a {@link CharSection} with the given char payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
*/
public CharSection( String aAlias, Character aPayload ) {
this( aAlias, aPayload, TransmissionMetrics.DEFAULT_ENCODING );
}
/**
* Constructs a {@link CharSection} with the given char payload and the
* given {@link Charset}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
* @param aCharset The charset to be used for encoding.
*/
public CharSection( String aAlias, Character aPayload, Charset aCharset ) {
super( aAlias, aPayload );
_charset = aCharset != null ? aCharset.name() : TransmissionMetrics.DEFAULT_ENCODING.name();
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Sequence toSequence() {
return new ByteArraySequence( Character.toString( getPayload() ).getBytes( getEncoding() ) );
}
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( Sequence aSequence, int aOffset, int aLength ) throws TransmissionException {
final byte[] theRecord = aSequence.toBytes( aOffset, aLength );
final String theTemp;
theTemp = new String( theRecord, getEncoding() );
if ( theTemp.length() != 1 ) {
throw new TransmissionSequenceException( aSequence, "The sequence with length <" + aLength + "> at the offset <" + aOffset + "> results in <" + theTemp + ">, though a single character is expected!" );
}
setPayload( theTemp.charAt( 0 ) );
}
/**
* {@inheritDoc}
*/
@Override
public int getLength() {
return toSequence().getLength();
}
/**
* {@inheritDoc}
*/
@Override
public SerialSchema toSchema() {
return new SerialSchema( getAlias(), getClass(), toSequence(), getLength(), "" + getPayload(), "A body containing an byte payload." );
}
/**
* {@inheritDoc}
*/
@Override
public CharSection withPayload( Character aValue ) {
setPayload( aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public Charset getEncoding() {
return _charset != null ? Charset.forName( _charset ) : TransmissionMetrics.DEFAULT_ENCODING;
}
}