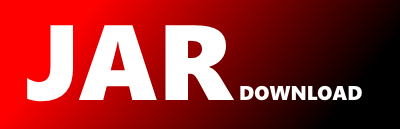
org.refcodes.serial.CrossoverLoopbackPortHub Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.io.IOException;
/**
* A {@link CrossoverLoopbackPortHub} is an in-memory implementation of a
* {@link PortHub} which's {@link Port} instances loop their output directly to
* the "connected" {@link LoopbackPortHub} counterpart {@link Port} instances
* ("other end of the wire"). The counterpart {@link Port} instances have the
* same alias so it is easy to retrieve the crossover {@link LoopbackPort}
* instances. This is suitable for cases using a some kind of frequent handshake
* between a transmitter and a receiver on the same line where we have to
* simulate a bidirectional in-memory communication between two ports.
*/
public class CrossoverLoopbackPortHub extends LoopbackPortHub {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private final LoopbackPortHub _crossoverPortHub;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs a {@link CrossoverLoopbackPortHub} with the given
* {@link LoopbackPortHub} as counterpart.
*
* @param aCrossoverPortHub The {@link CrossoverLoopbackPortHub}'s
* counterpart {@link LoopbackPortHub}.
*/
public CrossoverLoopbackPortHub( LoopbackPortHub aCrossoverPortHub ) {
_crossoverPortHub = aCrossoverPortHub;
}
/**
* Constructs a {@link CrossoverLoopbackPortHub} with the given
* {@link LoopbackPortHub} as counterpart and the given ports (e.g. ports
* with the given port aliases).
*
* @param aCrossoverPortHub The {@link CrossoverLoopbackPortHub}'s
* counterpart {@link LoopbackPortHub}.
* @param aPorts The ports to be created initially.
*/
public CrossoverLoopbackPortHub( LoopbackPortHub aCrossoverPortHub, String... aPorts ) {
super( aPorts );
_crossoverPortHub = aCrossoverPortHub;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public LoopbackPort toPort( String aAlias ) throws IOException {
final LoopbackPort theCrossoverPort = super.toPort( aAlias );
final LoopbackPort thePort = _crossoverPortHub.toPort( aAlias );
theCrossoverPort.setCrossoverPort( thePort );
thePort.setCrossoverPort( theCrossoverPort );
return theCrossoverPort;
}
/**
* {@inheritDoc}
*/
@Override
public LoopbackPort[] ports() throws IOException {
final LoopbackPort[] theCrossoverPorts = super.ports();
LoopbackPort ePort;
for ( LoopbackPort eCrossoverPort : theCrossoverPorts ) {
try {
ePort = _crossoverPortHub.toPort( eCrossoverPort.getAlias() );
ePort.setCrossoverPort( eCrossoverPort );
eCrossoverPort.setCrossoverPort( ePort );
}
catch ( IOException ignore ) {}
}
return theCrossoverPorts;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy