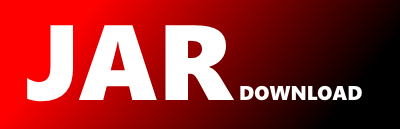
org.refcodes.serial.EnumSegment Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import org.refcodes.mixin.TypeAccessor;
import org.refcodes.numerical.Endianess;
import org.refcodes.textual.CaseStyleBuilder;
/**
* The {@link EnumSegment} is an implementation of a {@link Segment} carrying an
* enumeration value as payload.
*
* @param The type of the enumeration.
*/
public class EnumSegment> extends AbstractPayloadSegment> implements Segment, TypeAccessor {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
public static final String ENDIANESS = "ENDIANESS";
public static final int BYTES = Integer.BYTES;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private Endianess _endianess;
private Class _type;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aType the enumeratrion's type
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public EnumSegment( Class aType, TransmissionMetrics aTransmissionMetrics ) {
this( aType, aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aValue The payload to be contained by the
* {@link DoubleArraySection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public EnumSegment( Enum aValue, TransmissionMetrics aTransmissionMetrics ) {
this( aValue, aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aType the enumeratrion's type
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public EnumSegment( String aAlias, Class aType, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, aType, aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aValue The payload to be contained by the
* {@link DoubleArraySection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public EnumSegment( String aAlias, Enum aValue, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, aValue, aTransmissionMetrics.getEndianess() );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link EnumSegment} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link EnumSegment}'s value.
*
* @param aType the enumeratrion's type
*/
public EnumSegment( Class aType ) {
this( CaseStyleBuilder.asCamelCase( EnumSegment.class.getSimpleName() ), aType );
}
/**
* Constructs an empty {@link EnumSegment} with the given {@link Endianess}.
*
* @param aType the enumeratrion's type
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
public EnumSegment( Class aType, Endianess aEndianess ) {
this( CaseStyleBuilder.asCamelCase( EnumSegment.class.getSimpleName() ), aType, aEndianess );
}
// DON'T: There are situations where are mistaken as value:
// /**
// * Constructs a {@link EnumSegment} with the given enumeration value
// * (payload) and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian
// * representation of the {@link EnumSegment}'s value.
// *
// * @param aValue The value (payload) to be contained by the
// * {@link EnumSegment}.
// */
// public EnumSegment( Enum aValue ) {
// this( CaseStyleBuilder.asCamelCase( EnumSegment.class.getSimpleName() ), aValue );
// }
/**
* Constructs a {@link EnumSegment} with the given enumeration value
* (payload) and the given {@link Endianess} for the representation of the
* {@link EnumSegment}'s value.
*
* @param aValue The value (payload) to be contained by the
* {@link EnumSegment}.
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
public EnumSegment( Enum aValue, Endianess aEndianess ) {
this( CaseStyleBuilder.asCamelCase( EnumSegment.class.getSimpleName() ), aValue, aEndianess );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link EnumSegment} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link EnumSegment}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aType the enumeratrion's type
*/
public EnumSegment( String aAlias, Class aType ) {
this( aAlias, aType, TransmissionMetrics.DEFAULT_ENDIANESS );
}
/**
* Constructs an empty {@link EnumSegment} with the given {@link Endianess}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aType the enumeratrion's type
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
public EnumSegment( String aAlias, Class aType, Endianess aEndianess ) {
super( aAlias, null );
_endianess = aEndianess;
_type = aType;
}
// DON'T: There are situations where are mistaken as value:
// /**
// * Constructs a {@link EnumSegment} with the given enumeration value
// * (payload) and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian
// * representation of the {@link EnumSegment}'s value.
// *
// * @param aAlias The alias which identifies the content of this segment.
// * @param aValue The value (payload) to be contained by the
// * {@link EnumSegment}.
// */
// public EnumSegment( String aAlias, Enum aValue ) {
// this( aAlias, aValue, TransmissionMetrics.DEFAULT_ENDIANESS );
// }
/**
* Constructs a {@link EnumSegment} with the given enumeration value
* (payload) and the given {@link Endianess} for the representation of the
* {@link EnumSegment}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The value (payload) to be contained by the
* {@link EnumSegment}.
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
@SuppressWarnings("unchecked")
public EnumSegment( String aAlias, Enum aValue, Endianess aEndianess ) {
super( aAlias, aValue );
_endianess = aEndianess;
_type = (Class) aValue.getClass();
}
/**
* Constructs a {@link EnumSegment} with the given enumeration value
* (payload) and the given {@link Endianess} for the representation of the
* {@link EnumSegment}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aType the enumeration's type
* @param aValue The value (payload) to be contained by the
* {@link EnumSegment}.
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
@SuppressWarnings("unchecked")
public EnumSegment( String aAlias, Class aType, Enum aValue, Endianess aEndianess ) {
super( aAlias, aValue );
_endianess = aEndianess;
_type = aType;
if ( _type == null && aValue != null ) {
_type = (Class) aValue.getClass();
}
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Sequence toSequence() {
return new ByteArraySequence( _endianess.toBytes( getPayload() != null ? getPayload().ordinal() : 0, BYTES ) );
}
/**
* {@inheritDoc}
*/
@Override
public int fromTransmission( Sequence aSequence, int aOffset ) throws TransmissionException {
final byte[] theRecord = aSequence.toBytes( aOffset, BYTES );
setPayload( _type.getEnumConstants()[_endianess.toInteger( theRecord )] );
return aOffset + BYTES;
}
/**
* {@inheritDoc}
*/
@Override
public int getLength() {
return BYTES;
}
/**
* {@inheritDoc}
*/
@Override
public SerialSchema toSchema() {
final SerialSchema theSchema = new SerialSchema( getAlias(), getClass(), toSequence(), getLength(), "" + getPayload(), "A body containing an enumeration payload." );
theSchema.put( ENDIANESS, _endianess );
return theSchema;
}
/**
* {@inheritDoc}
*/
@Override
public EnumSegment withPayload( Enum aValue ) {
setPayload( aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public Class getType() {
return _type;
}
}