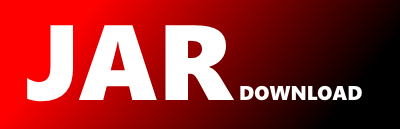
org.refcodes.serial.FloatArraySection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.util.Arrays;
import org.refcodes.numerical.Endianess;
import org.refcodes.textual.CaseStyleBuilder;
/**
* The {@link FloatArraySection} is an implementation of a {@link Section}
* carrying a float array as payload.
*/
public class FloatArraySection extends AbstractPayloadSection {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
public static final String ENDIANESS = "ENDIANESS";
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private Endianess _endianess;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public FloatArraySection( TransmissionMetrics aTransmissionMetrics ) {
this( aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link FloatArraySection}.
*/
public FloatArraySection( TransmissionMetrics aTransmissionMetrics, float... aValue ) {
this( aTransmissionMetrics.getEndianess(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link FloatArraySection}.
*/
public FloatArraySection( TransmissionMetrics aTransmissionMetrics, Float... aValue ) {
this( aTransmissionMetrics.getEndianess(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public FloatArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link FloatArraySection}.
*/
public FloatArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics, float... aValue ) {
this( aAlias, aTransmissionMetrics.getEndianess(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link FloatArraySection}.
*/
public FloatArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics, Float... aValue ) {
this( aAlias, aTransmissionMetrics.getEndianess(), aValue );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link FloatArraySection} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link Section}'s value.
*/
public FloatArraySection() {
this( CaseStyleBuilder.asCamelCase( FloatArraySection.class.getSimpleName() ) );
}
/**
* Constructs an empty {@link FloatArraySection} with the given
* {@link Endianess}.
*
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
public FloatArraySection( Endianess aEndianess ) {
this( CaseStyleBuilder.asCamelCase( FloatArraySection.class.getSimpleName() ), aEndianess );
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( float... aValue ) {
this( CaseStyleBuilder.asCamelCase( FloatArraySection.class.getSimpleName() ), aValue );
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( Float... aValue ) {
this( CaseStyleBuilder.asCamelCase( FloatArraySection.class.getSimpleName() ), aValue );
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( Endianess aEndianess, float... aValue ) {
this( CaseStyleBuilder.asCamelCase( FloatArraySection.class.getSimpleName() ), aEndianess, aValue );
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( Endianess aEndianess, Float... aValue ) {
this( CaseStyleBuilder.asCamelCase( FloatArraySection.class.getSimpleName() ), aEndianess, toPrimitiveArray( aValue ) );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link FloatArraySection} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link Section}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
*/
public FloatArraySection( String aAlias ) {
this( aAlias, TransmissionMetrics.DEFAULT_ENDIANESS, new float[0] );
}
/**
* Constructs an empty {@link FloatArraySection} with the given
* {@link Endianess}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
public FloatArraySection( String aAlias, Endianess aEndianess ) {
this( aAlias, aEndianess, new float[0] );
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( String aAlias, float... aValue ) {
this( aAlias, TransmissionMetrics.DEFAULT_ENDIANESS, aValue );
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( String aAlias, Float... aValue ) {
this( aAlias, TransmissionMetrics.DEFAULT_ENDIANESS, aValue );
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( String aAlias, Endianess aEndianess, float... aValue ) {
super( aAlias, aValue );
_endianess = aEndianess;
}
/**
* Constructs a {@link FloatArraySection} with the given float array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public FloatArraySection( String aAlias, Endianess aEndianess, Float... aValue ) {
super( aAlias, toPrimitiveArray( aValue ) );
_endianess = aEndianess;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Sequence toSequence() {
final byte[] theBytes = new byte[getPayload().length * Float.BYTES];
byte[] eRecord;
for ( int i = 0; i < getPayload().length; i++ ) {
eRecord = _endianess.toBytes( getPayload()[i] );
for ( int j = 0; j < eRecord.length; j++ ) {
theBytes[i * Float.BYTES + j] = eRecord[j];
}
}
return new ByteArraySequence( theBytes );
}
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( Sequence aSequence, int aOffset, int aLength ) throws TransmissionException {
if ( aLength % Float.BYTES != 0 ) {
throw new TransmissionSequenceException( aSequence, "The length dedicated <" + aLength + "> does not match a multiple float length <" + Float.BYTES + "> for retrieving an float array from the given sequence at the offset <" + aOffset + ">!" );
}
final float[] theValues = new float[aLength / Float.BYTES];
byte[] eRecord;
for ( int i = 0; i < theValues.length; i++ ) {
eRecord = aSequence.toBytes( aOffset + i * Float.BYTES, Float.BYTES );
theValues[i] = _endianess.toFloat( eRecord );
}
setPayload( theValues );
}
/**
* {@inheritDoc}
*/
@Override
public int getLength() {
return getPayload() != null ? getPayload().length * Float.BYTES : 0;
}
/**
* {@inheritDoc}
*/
@Override
public SerialSchema toSchema() {
final SerialSchema theSchema = new SerialSchema( getAlias(), getClass(), toSequence(), getLength(), "A body containing an float payload." );
theSchema.put( ENDIANESS, _endianess );
return theSchema;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getSimpleName() + " [alias=" + _alias + ", value=" + Arrays.toString( getPayload() ) + "]";
}
/**
* {@inheritDoc}
*/
@Override
public FloatArraySection withPayload( float[] aValue ) {
setPayload( aValue );
return this;
}
/**
* Convenience method to convert the array of wrapper types into its
* counterpart with primitive types before invoking
* {@link #setPayload(Object)}.
*
* @param aPayload The payload with the wrapper types.
*/
public void setPayload( Float[] aPayload ) {
setPayload( toPrimitiveArray( aPayload ) );
}
/**
* Convenience method to convert the array of wrapper types into its
* counterpart with primitive types before invoking
* {@link #withPayload(float[])}.
*
* @param aPayload The payload with the wrapper types.
*
* @return This instance as of the builder pattern.
*/
public FloatArraySection withPayload( Float[] aPayload ) {
setPayload( toPrimitiveArray( aPayload ) );
return this;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
private static float[] toPrimitiveArray( Float[] aPayload ) {
final float[] theResult = new float[aPayload.length];
for ( int i = 0; i < theResult.length; i++ ) {
theResult[i] = aPayload[i];
}
return theResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy