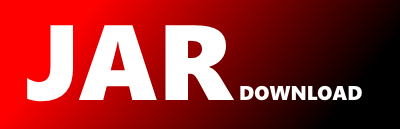
org.refcodes.serial.IntArraySection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.util.Arrays;
import org.refcodes.numerical.Endianess;
import org.refcodes.textual.CaseStyleBuilder;
/**
* The {@link IntArraySection} is an implementation of a {@link Section}
* carrying an integer array as payload.
*/
public class IntArraySection extends AbstractPayloadSection {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
public static final String ENDIANESS = "ENDIANESS";
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private Endianess _endianess;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public IntArraySection( TransmissionMetrics aTransmissionMetrics ) {
this( aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the {@link IntArraySection}.
*/
public IntArraySection( TransmissionMetrics aTransmissionMetrics, int... aValue ) {
this( aTransmissionMetrics.getEndianess(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the {@link IntArraySection}.
*/
public IntArraySection( TransmissionMetrics aTransmissionMetrics, Integer... aValue ) {
this( aTransmissionMetrics.getEndianess(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public IntArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the {@link IntArraySection}.
*/
public IntArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics, int... aValue ) {
this( aAlias, aTransmissionMetrics.getEndianess(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the {@link IntArraySection}.
*/
public IntArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics, Integer... aValue ) {
this( aAlias, aTransmissionMetrics.getEndianess(), aValue );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link IntArraySection} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link Section}'s value.
*/
public IntArraySection() {
this( CaseStyleBuilder.asCamelCase( IntArraySection.class.getSimpleName() ), new int[0] );
}
/**
* Constructs an empty {@link IntArraySection} with the given
* {@link Endianess}.
*
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
public IntArraySection( Endianess aEndianess ) {
this( CaseStyleBuilder.asCamelCase( IntArraySection.class.getSimpleName() ), aEndianess, new int[0] );
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( int... aValue ) {
this( CaseStyleBuilder.asCamelCase( IntArraySection.class.getSimpleName() ), aValue );
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( Integer... aValue ) {
this( CaseStyleBuilder.asCamelCase( IntArraySection.class.getSimpleName() ), aValue );
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( Endianess aEndianess, int... aValue ) {
this( CaseStyleBuilder.asCamelCase( IntArraySection.class.getSimpleName() ), aEndianess, aValue );
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( Endianess aEndianess, Integer... aValue ) {
this( CaseStyleBuilder.asCamelCase( IntArraySection.class.getSimpleName() ), aEndianess, toPrimitiveArray( aValue ) );
_endianess = aEndianess;
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link IntArraySection} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link Section}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
*/
public IntArraySection( String aAlias ) {
this( aAlias, TransmissionMetrics.DEFAULT_ENDIANESS, new int[0] );
}
/**
* Constructs an empty {@link IntArraySection} with the given
* {@link Endianess}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aEndianess The {@link Endianess} to be used for payload values.
*/
public IntArraySection( String aAlias, Endianess aEndianess ) {
this( aAlias, aEndianess, new int[0] );
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( String aAlias, int... aValue ) {
this( aAlias, TransmissionMetrics.DEFAULT_ENDIANESS, aValue );
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation
* of the {@link Section}'s value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( String aAlias, Integer... aValue ) {
this( aAlias, TransmissionMetrics.DEFAULT_ENDIANESS, aValue );
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( String aAlias, Endianess aEndianess, int... aValue ) {
super( aAlias, aValue );
_endianess = aEndianess;
}
/**
* Constructs a {@link IntArraySection} with the given integer array payload
* and {@link Endianess} for the representation of the {@link Section}'s
* value.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aEndianess The {@link Endianess} to be used for payload values.
* @param aValue The payload to be contained by the {@link Section}.
*/
public IntArraySection( String aAlias, Endianess aEndianess, Integer... aValue ) {
super( aAlias, toPrimitiveArray( aValue ) );
_endianess = aEndianess;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Sequence toSequence() {
final byte[] theBytes = new byte[getPayload().length * Integer.BYTES];
byte[] eRecord;
for ( int i = 0; i < getPayload().length; i++ ) {
eRecord = _endianess.toBytes( getPayload()[i], Integer.BYTES );
for ( int j = 0; j < eRecord.length; j++ ) {
theBytes[i * Integer.BYTES + j] = eRecord[j];
}
}
return new ByteArraySequence( theBytes );
}
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( Sequence aSequence, int aOffset, int aLength ) throws TransmissionException {
if ( aLength % Integer.BYTES != 0 ) {
throw new TransmissionSequenceException( aSequence, "The length dedicated <" + aLength + "> does not match a multiple integer length <" + Integer.BYTES + "> for retrieving an integer array from the given sequence at the offset <" + aOffset + ">!" );
}
final int[] theInts = new int[aLength / Integer.BYTES];
byte[] eRecord;
for ( int i = 0; i < theInts.length; i++ ) {
eRecord = aSequence.toBytes( aOffset + i * Integer.BYTES, Integer.BYTES );
theInts[i] = _endianess.toInteger( eRecord );
}
setPayload( theInts );
}
/**
* {@inheritDoc}
*/
@Override
public int getLength() {
return getPayload() != null ? getPayload().length * Integer.BYTES : 0;
}
/**
* {@inheritDoc}
*/
@Override
public SerialSchema toSchema() {
final SerialSchema theSchema = new SerialSchema( getAlias(), getClass(), toSequence(), getLength(), "A body containing an integer payload." );
theSchema.put( ENDIANESS, _endianess );
return theSchema;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getSimpleName() + " [alias=" + _alias + ", value=" + Arrays.toString( getPayload() ) + "]";
}
/**
* {@inheritDoc}
*/
@Override
public IntArraySection withPayload( int[] aValue ) {
setPayload( aValue );
return this;
}
/**
* Convenience method to convert the array of wrapper types into its
* counterpart with primitive types before invoking
* {@link #setPayload(Object)}.
*
* @param aPayload The payload with the wrapper types.
*/
public void setPayload( Integer[] aPayload ) {
setPayload( toPrimitiveArray( aPayload ) );
}
/**
* Convenience method to convert the array of wrapper types into its
* counterpart with primitive types before invoking
* {@link #withPayload(int[])}.
*
* @param aPayload The payload with the wrapper types.
*
* @return This instance as of the builder pattern.
*/
public IntArraySection withPayload( Integer[] aPayload ) {
setPayload( toPrimitiveArray( aPayload ) );
return this;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
private static int[] toPrimitiveArray( Integer[] aPayload ) {
final int[] theResult = new int[aPayload.length];
for ( int i = 0; i < theResult.length; i++ ) {
theResult[i] = aPayload[i];
}
return theResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy