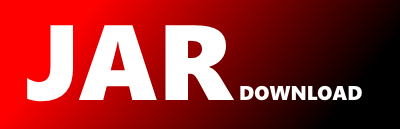
org.refcodes.serial.MagicBytes Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import org.refcodes.data.Ascii;
/**
* The {@link MagicBytes} enumeration provides various predefined magic bytes
* for common use cases. Some magic bytes may be combined using the
* {@link #toMagicBytes()} method to create magic bytes with more semantic
* information.
*
*/
public enum MagicBytes implements MagicBytesAccessor {
/**
* Magic bytes identifying a transmission acknowledge, same as
* {@link Ascii#ACK}.
*/
ACKNOWLEDGE((byte) Ascii.ACK.getCode()),
/**
* Magic bytes identifying a Clear-to-Send transmission, same as
* {@link Ascii#ACK}.
*/
CLEAR_TO_SEND(Ascii.ACK.getCode()),
/**
* Magic bytes identifying a last transmission package, same as
* {@link Ascii#ETX}.
*/
LAST_PACKET(Ascii.ETX.getCode()),
/**
* Magic bytes identifying an transmission package, same as
* {@link Ascii#STX}.
*/
PACKET(Ascii.STX.getCode()),
/**
* Magic bytes identifying a Ready-to-Receive transmission, same as
* {@link Ascii#ENQ}.
*/
READY_TO_RECEIVE(Ascii.ENQ.getCode()),
/**
* Magic bytes identifying a Ready-to-Send transmission, same as
* {@link Ascii#ENQ}.
*/
READY_TO_SEND(Ascii.ENQ.getCode()),
/**
* Identifies a fire-and-forget transmission (no acknowledge sent).
*/
TRANSMISSION(Ascii.NULL.getCode()),
/**
* Identifies a request expecting a fire-and-forget response (no acknowledge
* to be sent for the succeeding response).
*/
REQUEST(Ascii.ENQ.getCode()),
/**
* Identifies a fire-and-forget response in reply to a request (no
* acknowledge to be sent for the response).
*/
RESPONSE(Ascii.EOT.getCode()),
/**
* Identifies an acknowledge in reply to a response.
*/
RESPONSE_ACKNOWLEDGE(Ascii.EM.getCode()),
/**
* Identifies an acknowledgeable transmission (acknowledge to be sent).
*/
ACKNOWLEDGEABLE_TRANSMISSION(Ascii.SYN.getCode()),
/**
* Identifies a request expecting an acknowledgeable response (acknowledge
* to be sent sent for the succeeding response).
*/
ACKNOWLEDGEABLE_REQUEST(Ascii.STX.getCode()),
/**
* Identifies an acknowledgeable response in reply to a request (acknowledge
* to be sent for the response).
*/
ACKNOWLEDGEABLE_RESPONSE(Ascii.ETX.getCode()),
/**
* Sent in case a transmission was received and dismissed by a receiver.
*/
TRANSMISSION_DISMISSED(Ascii.NAK.getCode()),
/**
* Sent when a request was received but dismissed by a receiver .
*/
REQUEST_DISMISSED(Ascii.CAN.getCode()),
/**
* Identifies a simple ping request to be responded by a fire-and-forget
* {@link #PONG} (not to be acknowledged).
*/
PING("?"),
/**
* Identifies a a fire-and-forget pong (not to be acknowledged) reply in
* response to a simple {@link #PING} request.
*/
PONG("!");
private byte[] _magicBytes;
private MagicBytes( byte... aMagicBytes ) {
_magicBytes = aMagicBytes;
}
private MagicBytes( String aMagicBytes ) {
_magicBytes = aMagicBytes.getBytes( StandardCharsets.US_ASCII );
}
private MagicBytes( String aMagicBytes, Charset aCharset ) {
_magicBytes = aMagicBytes.getBytes( aCharset );
}
/**
* {@inheritDoc}
*/
@Override
public byte[] getMagicBytes() {
return _magicBytes;
}
/**
* Constructs magic bytes from the provided {@link MagicBytes} elements to
* be concatenated to a single magic bytes sequence.
*
* @param aMagicBytes The {@link MagicBytes} elements from which to create
* the magic bytes.
*
* @return The accordingly created magic bytes.
*/
public static byte[] toMagicBytes( MagicBytes... aMagicBytes ) {
final Sequence theMagicBytes = new ByteArraySequence();
for ( MagicBytes eMagicBytes : aMagicBytes ) {
theMagicBytes.append( eMagicBytes.getMagicBytes() );
}
return theMagicBytes.toBytes();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy