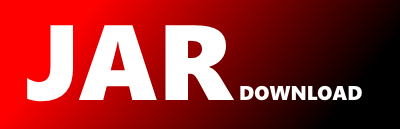
org.refcodes.serial.MagicBytesSectionMultiplexer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Collection;
/**
* THe {@link MagicBytesSectionMultiplexer} dispatches a transmission to one of
* the aggregated {@link Section} instances depending on the magic number
* provided by the transmission. A transmission is passed to each of the
* aggregated {@link Section} instances till one {@link Section} accepts the
* transmission, e.g. until a {@link Section} does not throw a
* {@link BadMagicBytesException}. To enforce throwing a
* {@link BadMagicBytesException} use a magic bytes {@link Segment} or
* {@link Section} which enforces the magic bytes to of a transmission to match
* its own magic bytes, e.e. use one of the following:
* {@link AssertMagicBytesSectionDecorator}, {@link AssertMagicBytesSegment},
* {@link AssertMagicBytesSegmentDecorator} Attention: A {@link Section}
* throwing a {@link TransmissionException} other than a
* {@link BadMagicBytesException} is considered to be responsible for the
* transmission so that dispatching is *not* continued with the succeeding
* {@link Section}! The last {@link Section} which was responsible for a
* transmission's magic bytes will be the responsible {@link Section} till
* another {@link Section} claims responsibility for a transmsision's magic
* bytes. Initially the first {@link Section} passed to this instance is the
* responsible {@link Section}.
*/
public class MagicBytesSectionMultiplexer extends AbstractMagicBytesTransmissionMultiplexer implements Section {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
public MagicBytesSectionMultiplexer() {}
/**
* {@inheritDoc}
*/
public MagicBytesSectionMultiplexer( Collection aSections, int aReadLimit ) {
super( aSections, aReadLimit );
}
/**
* {@inheritDoc}
*/
public MagicBytesSectionMultiplexer( Collection aSections ) {
super( aSections );
}
/**
* {@inheritDoc}
*/
public MagicBytesSectionMultiplexer( int aReadLimit, Section... aSections ) {
super( aReadLimit, aSections );
}
/**
* {@inheritDoc}
*/
public MagicBytesSectionMultiplexer( Section... aSections ) {
super( aSections );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( byte[] aChunk, int aLength ) throws TransmissionException {
fromTransmission( new ByteArraySequence( aChunk ), 0, aLength );
}
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( Sequence aSequence, int aLength ) throws TransmissionException {
fromTransmission( aSequence, 0, aLength );
}
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( byte[] aChunk, int aOffset, int aLength ) throws TransmissionException {
fromTransmission( new ByteArraySequence( aChunk ), aOffset, aLength );
}
/**
* {@inheritDoc}
*/
@Override
public void receiveFrom( InputStream aInputStream, int aLength ) throws IOException {
receiveFrom( aInputStream, aLength, null );
}
/**
* {@inheritDoc}
*/
@Override
public void receiveFrom( SerialTransceiver aSerialTransceiver, int aLength ) throws IOException {
receiveFrom( aSerialTransceiver.getInputStream(), aLength, aSerialTransceiver.getOutputStream() );
}
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( Sequence aSequence, int aOffset, int aLength ) throws TransmissionException {
if ( _children != null ) {
for ( Section a_children : _children ) {
try {
a_children.fromTransmission( aSequence, aOffset, aLength );
_responsibility = a_children;
return;
}
catch ( BadCrcChecksumException | BadMagicBytesException ignore ) {}
}
}
throw new TransmissionSequenceException( aSequence, aOffset, "There are no segments to which to transmit the given sequence!" );
}
/**
* {@inheritDoc}
*/
@Override
public void receiveFrom( InputStream aInputStream, int aLength, OutputStream aReturnStream ) throws IOException {
if ( _children != null ) {
final BufferedInputStream theInputStream = new BufferedInputStream( aInputStream );
theInputStream.mark( _readLimit );
for ( Section a_children : _children ) {
try {
a_children.receiveFrom( theInputStream, aLength, aReturnStream );
_responsibility = a_children;
return;
}
catch ( BadCrcChecksumException | BadMagicBytesException ignore ) {
theInputStream.reset();
}
}
}
throw new TransmissionException( "There are no segments to which to transmit the given transmission to!" );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy