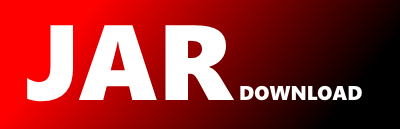
org.refcodes.serial.PropertiesSection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.nio.charset.Charset;
import java.text.ParseException;
import org.refcodes.data.Literal;
import org.refcodes.mixin.EncodingAccessor;
import org.refcodes.properties.Properties;
import org.refcodes.properties.Properties.PropertiesBuilder;
import org.refcodes.properties.PropertiesBuilderImpl;
import org.refcodes.properties.PropertiesImpl;
import org.refcodes.struct.SimpleTypeMap;
import org.refcodes.struct.SimpleTypeMapImpl;
import org.refcodes.textual.CaseStyleBuilder;
import org.refcodes.textual.EscapeTextBuilder;
/**
* The {@link PropertiesSection} is an implementation of a {@link Section}
* carrying a {@link String} as payload.
*/
public class PropertiesSection extends AbstractPayloadSection implements Section, EncodingAccessor {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _charset; // <--| "String" instead of "Charset" in order to be serializable
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs a {@link PropertiesSection} with the given {@link Properties}
* payload being encoded with the given {@link Charset}. The properties from
* the payload are copied into the {@link PropertiesSection}. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aPayload The payload to be contained by the {@link Section}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public PropertiesSection( Properties aPayload, TransmissionMetrics aTransmissionMetrics ) {
this( CaseStyleBuilder.asCamelCase( PropertiesSection.class.getSimpleName() ), new PropertiesImpl( aPayload ), aTransmissionMetrics.getEncoding() );
}
/**
* Constructs a {@link PropertiesSection} with the given {@link Properties}
* payload being encoded with the given {@link Charset}. The properties from
* the payload are copied into the {@link PropertiesSection}. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*/
public PropertiesSection( String aAlias, Properties aPayload, TransmissionMetrics aTransmissionMetrics ) {
this( aAlias, new PropertiesImpl( aPayload ), aTransmissionMetrics.getEncoding() );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link PropertiesSection} using UTF-8 encoding for
* the payload.
*/
public PropertiesSection() {
this( CaseStyleBuilder.asCamelCase( PropertiesSection.class.getSimpleName() ), new PropertiesImpl() );
}
/**
* Constructs a {@link PropertiesSection} with the given {@link Properties}
* payload being UTF-8 encoded. The properties from the payload are copied
* into the {@link PropertiesSection}.
*
* @param aPayload The payload to be contained by the {@link Section}.
*/
public PropertiesSection( Properties aPayload ) {
this( CaseStyleBuilder.asCamelCase( PropertiesSection.class.getSimpleName() ), aPayload );
}
/**
* Constructs a {@link PropertiesSection} with a payload expected to be
* encoded with the given {@link Charset}.
*
* @param aCharset The {@link Charset} to be used for encoding the
* {@link String}.
*/
public PropertiesSection( Charset aCharset ) {
this( CaseStyleBuilder.asCamelCase( PropertiesSection.class.getSimpleName() ), new PropertiesImpl(), aCharset );
}
/**
* Constructs a {@link PropertiesSection} with the given {@link Properties}
* payload being encoded with the given {@link Charset}. The properties from
* the payload are copied into the {@link PropertiesSection}.
*
* @param aPayload The payload to be contained by the {@link Section}.
* @param aCharset The {@link Charset} to be used for encoding the
* {@link String}.
*/
public PropertiesSection( Properties aPayload, Charset aCharset ) {
this( CaseStyleBuilder.asCamelCase( PropertiesSection.class.getSimpleName() ), new PropertiesImpl( aPayload ), aCharset );
}
// -------------------------------------------------------------------------
/**
* Constructs an empty {@link PropertiesSection} using UTF-8 encoding for
* the payload.
*
* @param aAlias The alias which identifies the content of this segment.
*/
public PropertiesSection( String aAlias ) {
this( aAlias, new PropertiesImpl(), TransmissionMetrics.DEFAULT_ENCODING );
}
/**
* Constructs a {@link PropertiesSection} with the given {@link Properties}
* payload being UTF-8 encoded. The properties from the payload are copied
* into the {@link PropertiesSection}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
*/
public PropertiesSection( String aAlias, Properties aPayload ) {
this( aAlias, aPayload, TransmissionMetrics.DEFAULT_ENCODING );
}
/**
* Constructs a {@link PropertiesSection} with a payload expected to be
* encoded with the given {@link Charset}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aCharset The {@link Charset} to be used for encoding the
* {@link String}.
*/
public PropertiesSection( String aAlias, Charset aCharset ) {
this( aAlias, new PropertiesImpl(), aCharset );
}
/**
* Constructs a {@link PropertiesSection} with the given {@link Properties}
* payload being encoded with the given {@link Charset}. The properties from
* the payload are copied into the {@link PropertiesSection}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
* @param aCharset The {@link Charset} to be used for encoding the
* {@link String}.
*/
public PropertiesSection( String aAlias, Properties aPayload, Charset aCharset ) {
super( aAlias, new PropertiesImpl( aPayload ) );
_charset = aCharset != null ? aCharset.name() : TransmissionMetrics.DEFAULT_ENCODING.name();
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public Sequence toSequence() {
final String serialized = toSerialized();
return new ByteArraySequence( serialized.getBytes( getEncoding() ) );
}
/**
* {@inheritDoc}
*/
@Override
public void fromTransmission( Sequence aSequence, int aOffset, int aLength ) throws TransmissionException {
final byte[] theRecord = aSequence.toBytes( aOffset, aLength );
try {
parseFrom( new String( theRecord, getEncoding() ) );
}
catch ( ParseException e ) {
throw new TransmissionSequenceException( aSequence, "Cannot parse the sequence <" + aSequence.toHexString() + "> at offset <" + aOffset + ">!", e );
}
}
/**
* {@inheritDoc}
*/
@Override
public int getLength() {
return getPayload() != null ? toSerialized().length() : 0;
}
/**
* {@inheritDoc}
*/
@Override
public SerialSchema toSchema() {
final String theSerialized = toSerialized();
return new SerialSchema( getAlias(), getClass(), toSequence(), theSerialized.length(), theSerialized.replaceAll( "\n", "\\\\n" ).replaceAll( "\r", "\\\\r" ), "A body containing a string payload." );
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
final int prime = 31;
int result = super.hashCode();
result = prime * result + ( ( _charset == null ) ? 0 : _charset.hashCode() );
return result;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) {
return true;
}
if ( !super.equals( obj ) ) {
return false;
}
if ( getClass() != obj.getClass() ) {
return false;
}
final PropertiesSection other = (PropertiesSection) obj;
if ( _charset == null ) {
if ( other._charset != null ) {
return false;
}
}
else if ( !_charset.equals( other._charset ) ) {
return false;
}
return true;
}
/**
* {@inheritDoc}
*/
@Override
public PropertiesSection withPayload( Properties aValue ) {
setPayload( aValue );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SimpleTypeMap toSimpleTypeMap() {
return new SimpleTypeMapImpl( _payload );
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
private String toSerialized() {
final StringBuilder theBuilder = new StringBuilder();
String eValue;
for ( String eKey : getPayload().sortedKeys() ) {
eValue = getPayload().get( eKey );
eValue = EscapeTextBuilder.asEscaped( eValue );
theBuilder.append( eKey );
theBuilder.append( Literal.EQUALS.getValue() );
theBuilder.append( eValue );
theBuilder.append( '\n' );
}
return theBuilder.toString();
}
private void parseFrom( String aString ) throws ParseException {
if ( aString != null && aString.length() != 0 ) {
final PropertiesBuilder theProperties = new PropertiesBuilderImpl();
final String[] thePairs = aString.split( "\n" );
String[] eTupel;
for ( String eValue : thePairs ) {
eTupel = eValue.split( "=", 2 );
theProperties.put( eTupel[0], EscapeTextBuilder.asUnEscaped( eTupel[1] ) );
}
setPayload( new PropertiesImpl( (Properties) theProperties ) );
}
else {
setPayload( new PropertiesImpl() );
}
}
/**
* {@inheritDoc}
*/
@Override
public Charset getEncoding() {
return _charset != null ? Charset.forName( _charset ) : TransmissionMetrics.DEFAULT_ENCODING;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy