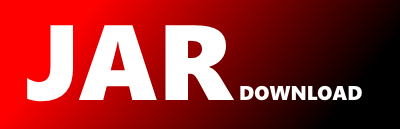
org.refcodes.serial.SerialReceiver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.io.IOException;
import java.io.InputStream;
import org.refcodes.component.LinkComponent.LinkAutomaton;
import org.refcodes.io.BytesReceiver;
import org.refcodes.io.Skippable;
/**
* A {@link SerialReceiver} is used to read data from a serial port or the like.
*/
public interface SerialReceiver extends BytesReceiver, SegmentReceiver, LinkAutomaton, Skippable {
/**
* Returns the number of bytes which can be read directly e.g. which are
* already been stored in an internal buffer. May return (0 or) a value less
* than 0 (-1) when it is not supported. Similar to
* {@link InputStream#available()}: "Returns an estimate of the number of
* bytes that can be read (or skipped over) from this input stream without
* blocking by the next invocation of a method for this input stream. The
* next invocation might be the same thread or another thread. A single read
* or skip of this many bytes will not block, but may read or skip fewer
* bytes. Note that while some implementations of InputStream will return
* the total number of bytes in the stream, many will not. It is never
* correct to use the return value of this method to allocate a buffer
* intended to hold all data in this stream. A subclass' implementation of
* this method may choose to throw an IOException if this input stream has
* been closed by invoking the close() method. The available method for
* class InputStream always returns 0. This method should be overridden by
* subclasses."
*
* @return The number of available bytes: An estimate of the number of bytes
* that can be read (or skipped over) from this input stream without
* blocking or 0 when it reaches the end of the input stream.
* Throws:
*
* @throws IOException - if an I/O error occurs.
*/
@Override
int available() throws IOException;
/**
* {@inheritDoc}
*/
@Override
default byte receiveByte() throws IOException {
return receiveBytes( 1 )[0];
}
/**
* {@inheritDoc}
*/
@Override
default byte[] receiveBytes( int aLength ) throws IOException {
final byte[] theChunk = new byte[aLength];
receiveBytes( theChunk, 0, aLength );
return theChunk;
}
// /**
// * Receives a byte array with the number of bytes specified inserted at the
// * given offset. This method blocks till a byte is available.
// *
// * @param aChunk The byte array where to store the bytes at.
// *
// * @param aOffset The offset where to start storing the received bytes.
// *
// * @param aLength The number of bytes to receive.
// *
// * @throws IOException thrown in case of I/O issues (e.g. a timeout) while
// * receiving.
// */
// void receive( byte[] aChunk, int aOffset, int aLength ) throws IOException;
/**
* Receives a {@link Sequence} with the number of bytes specified. This
* method blocks till all bytes are read or the timeout has been reached.
*
* @param aTimeoutMillis The default timeout for read operations not
* explicitly called with a timeout argument. With a value of -1
* timeout handling is disabled (blocking mode) or a technical
* timeout occurs (implementation depended).
* @param aLength The number of bytes to receive.
*
* @return A {@link Sequence} containing the accordingly received bytes.
*
* @throws IOException thrown in case of I/O issues (e.g. a timeout) while
* receiving.
*/
default Sequence receiveSequenceWithin( long aTimeoutMillis, int aLength ) throws IOException {
return new ByteArraySequence( receiveBytesWithin( aTimeoutMillis, aLength ) );
}
/**
* Receives a {@link Sequence} with the number of bytes specified.This
* method blocks till all bytes are read.
*
* @param aLength The number of bytes to receive.
*
* @return A {@link Sequence} containing the accordingly received bytes.
*
* @throws IOException thrown in case of I/O issues (e.g. a timeout) while
* receiving.
*/
default Sequence receiveSequence( int aLength ) throws IOException {
return new ByteArraySequence( receiveBytes( aLength ) );
}
/**
* {@inheritDoc}
*/
@Override
default void receiveSegmentWithin( long aTimeoutMillis, SEGMENT aSegment ) throws IOException {
aSegment.receiveFrom( getInputStream( aTimeoutMillis ), null );
}
/**
* {@inheritDoc}
*/
@Override
default void receiveSegment( SEGMENT aSegment ) throws IOException {
aSegment.receiveFrom( getInputStream(), null );
}
/**
* {@inheritDoc} Attention: Implementations of this method should do a
* {@link Object#notifyAll()} in order to terminate any pending asynchronous
* operations such as {@link #onReceiveSegment(Segment)} or
* {@link #onReceiveSegment(Segment, SegmentConsumer)}.
*/
@Override
void open() throws IOException;
/**
* {@inheritDoc} Attention: Implementations of this method should do a
* {@link Object#notifyAll()} in order to terminate any pending asynchronous
* operations such as {@link #onReceiveSegment(Segment)} or
* {@link #onReceiveSegment(Segment, SegmentConsumer)}.
*/
@Override
void close() throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy