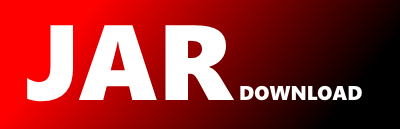
org.refcodes.serial.SerialSugar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-serial Show documentation
Show all versions of refcodes-serial Show documentation
Artifact providing generic (byte) serialization functionality including
a TTY-/COM-Port implementation of the serial framework as well as a (local)
loopback port.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.serial;
import java.io.File;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.charset.Charset;
import java.util.Collection;
import java.util.function.Supplier;
import javax.crypto.Cipher;
import org.refcodes.factory.TypeFactory;
import org.refcodes.mixin.ConcatenateMode;
import org.refcodes.numerical.BijectiveFunction;
import org.refcodes.numerical.ChecksumValidationMode;
import org.refcodes.numerical.CrcAlgorithm;
import org.refcodes.numerical.Endianess;
import org.refcodes.numerical.InverseFunction;
import org.refcodes.numerical.Invertible;
import org.refcodes.properties.Properties;
import org.refcodes.serial.SegmentPackager.DummySegmentPackager;
/**
* Declarative syntactic sugar which may be statically imported in order to
* allow declarative definitions for the construction of various serial types
* such as {@link Segment} or {@link Section} type instances (and the like).
*/
public class SerialSugar {
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new serial sugar.
*/
protected SerialSugar() {}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* The {@link NullSegment} represents a no-operation {@link Segment}, useful
* as placeholder in a {@link SegmentComposite}.
*
* @return The accordingly created {@link NullSegment}.
*/
public static NullSegment nullSegment() {
return new NullSegment();
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param The type of the enumeration.
* @param aType the enumeratrion's type
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( Class aType, TransmissionMetrics aTransmissionMetrics ) {
return new EnumSegment<>( aType, aTransmissionMetrics );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param The type of the enumeration.
* @param aValue The payload to be contained by the
* {@link DoubleArraySection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( Enum aValue, TransmissionMetrics aTransmissionMetrics ) {
return new EnumSegment<>( aValue, aTransmissionMetrics );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param The type of the enumeration.
* @param aAlias The alias which identifies the content of this instance.
* @param aType the enumeratrion's type
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( String aAlias, Class aType, TransmissionMetrics aTransmissionMetrics ) {
return new EnumSegment<>( aAlias, aType, aTransmissionMetrics );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param The type of the enumeration.
* @param aAlias The alias which identifies the content of this instance.
* @param aValue The payload to be contained by the
* {@link DoubleArraySection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( String aAlias, Enum aValue, TransmissionMetrics aTransmissionMetrics ) {
return new EnumSegment<>( aAlias, aValue, aTransmissionMetrics );
}
/**
* Constructs an empty {@link EnumSegment} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link EnumSegment}'s value.
*
* @param The type of the enumeration.
* @param aType the enumeratrion's type
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( Class aType ) {
return new EnumSegment<>( aType );
}
/**
* Constructs an empty {@link EnumSegment} with the given {@link Endianess}.
*
* @param The type of the enumeration.
* @param aType the enumeratrion's type
* @param aEndianess The {@link Endianess} to be used for payload values.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( Class aType, Endianess aEndianess ) {
return new EnumSegment<>( aType, aEndianess );
}
/**
* Constructs a {@link EnumSegment} with the given enumeration value
* (payload) and the given {@link Endianess} for the representation of the
* {@link EnumSegment}'s value.
*
* @param The type of the enumeration.
* @param aValue The value (payload) to be contained by the
* {@link EnumSegment}.
* @param aEndianess The {@link Endianess} to be used for payload values.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( Enum aValue, Endianess aEndianess ) {
return new EnumSegment<>( aValue, aEndianess );
}
/**
* Constructs an empty {@link EnumSegment} with a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the {@link EnumSegment}'s value.
*
* @param The type of the enumeration.
* @param aAlias The alias which identifies the content of this segment.
* @param aType the enumeratrion's type
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( String aAlias, Class aType ) {
return new EnumSegment<>( aAlias, aType );
}
/**
* Constructs an empty {@link EnumSegment} with the given {@link Endianess}.
*
* @param The type of the enumeration.
* @param aAlias The alias which identifies the content of this segment.
* @param aType the enumeratrion's type
* @param aEndianess The {@link Endianess} to be used for payload values.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( String aAlias, Class aType, Endianess aEndianess ) {
return new EnumSegment<>( aAlias, aType, aEndianess );
}
/**
* Constructs a {@link EnumSegment} with the given enumeration value
* (payload) and the given {@link Endianess} for the representation of the
* {@link EnumSegment}'s value.
*
* @param The type of the enumeration.
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The value (payload) to be contained by the
* {@link EnumSegment}.
* @param aEndianess The {@link Endianess} to be used for payload values.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( String aAlias, Enum aValue, Endianess aEndianess ) {
return new EnumSegment<>( aAlias, aValue, aEndianess );
}
/**
* Constructs a {@link EnumSegment} with the given enumeration value
* (payload) and the given {@link Endianess} for the representation of the
* {@link EnumSegment}'s value.
*
* @param The type of the enumeration.
* @param aAlias The alias which identifies the content of this segment.
* @param aType the enumeration's type
* @param aValue The value (payload) to be contained by the
* {@link EnumSegment}.
* @param aEndianess The {@link Endianess} to be used for payload values.
*
* @return The accordingly created {@link EnumSegment}.
*/
public static > EnumSegment enumSegment( String aAlias, Class aType, Enum aValue, Endianess aEndianess ) {
return new EnumSegment<>( aAlias, aType, aValue, aEndianess );
}
/**
* Constructs a {@link AllocSectionDecoratorSegment} with the given
* decoratee and a width of {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH}
* bytes used to specify the decoratee's length a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the decoratee's length.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The decoratee used for this decorator.
*
* @return The accordingly created {@link AllocSectionDecoratorSegment}.
*/
public static AllocSectionDecoratorSegment allocSegment( DECORATEE aDecoratee ) {
return new AllocSectionDecoratorSegment<>( aDecoratee );
}
/**
* Constructs a {@link AllocSectionDecoratorSegment} with the given
* decoratee and a width of {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH}
* bytes used to specify the decoratee's length and the provided
* {@link Endianess} representation of the decoratee's length.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The decoratee used for this decorator.
* @param aEndianess The {@link Endianess} to be used for length values.
*
* @return The accordingly created {@link AllocSectionDecoratorSegment}.
*/
public static AllocSectionDecoratorSegment allocSegment( DECORATEE aDecoratee, Endianess aEndianess ) {
return new AllocSectionDecoratorSegment<>( aDecoratee, aEndianess );
}
/**
* Constructs a {@link AllocSectionDecoratorSegment} with the given
* decoratee and with the given number of bytes used to specify the
* decoratee's length and a {@link TransmissionMetrics#DEFAULT_ENDIANESS}
* endian representation of the decoratee's length.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The decoratee used for this decorator.
* @param aLengthWidth The width (in bytes) to be used for length values.
*
* @return The accordingly created {@link AllocSectionDecoratorSegment}.
*/
public static AllocSectionDecoratorSegment allocSegment( DECORATEE aDecoratee, int aLengthWidth ) {
return new AllocSectionDecoratorSegment<>( aDecoratee, aLengthWidth );
}
/**
* Constructs a {@link AllocSectionDecoratorSegment} with the given
* decoratee and with the given number of bytes used to specify the
* decoratee's length and the provided {@link Endianess} representation of
* the decoratee's length.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The decoratee used for this decorator.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for length values.
*
* @return The accordingly created {@link AllocSectionDecoratorSegment}.
*/
public static AllocSectionDecoratorSegment allocSegment( DECORATEE aDecoratee, int aLengthWidth, Endianess aEndianess ) {
return new AllocSectionDecoratorSegment<>( aDecoratee, aLengthWidth, aEndianess );
}
/**
* Constructs an according {@link AllocSectionDecoratorSegment} instance.
* The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The decoratee used for this decorator.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AllocSectionDecoratorSegment}.
*/
public static AllocSectionDecoratorSegment allocSegment( DECORATEE aDecoratee, TransmissionMetrics aTransmissionMetrics ) {
return new AllocSectionDecoratorSegment<>( aDecoratee, aTransmissionMetrics );
}
/**
* Constructs the decorator with the given decoratee and a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the decoratee's length a {@link TransmissionMetrics#DEFAULT_ENDIANESS}
* endian representation of the decoratee's length.
*
* @param The type of the {@link Section} decoratee.
* @param aDecoratee The decoratee used for this decorator.
*
* @return The accordingly created {@link AllocSegmentBody}.
*/
public static AllocSegmentBody allocSegmentBody( DECORATEE aDecoratee ) {
return new AllocSegmentBody<>( aDecoratee );
}
/**
* Constructs an empty head with a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the body's length and a {@link TransmissionMetrics#DEFAULT_ENDIANESS}
* endian representation of the body's length.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead() {
return new AllocSegmentHead();
}
/**
* Constructs the head with the given body and a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the body's length a {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian
* representation of the body's length.
*
* @param aBody The body referenced by this head.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( AllocSegmentBody> aBody ) {
return new AllocSegmentHead( aBody );
}
/**
* Constructs the head with the given body and a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the body's length and the provided {@link Endianess} representation of
* the body's length.
*
* @param aBody The body referenced by this head.
* @param aEndianess The {@link Endianess} to be used for length values.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( AllocSegmentBody> aBody, Endianess aEndianess ) {
return new AllocSegmentHead( aBody, aEndianess );
}
/**
* Constructs the head with the given body and with the given number of
* bytes used to specify the body's length and a
* {@link TransmissionMetrics#DEFAULT_ENDIANESS} endian representation of
* the body's length.
*
* @param aBody The body referenced by this head.
* @param aLengthWidth The width (in bytes) to be used for length values.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( AllocSegmentBody> aBody, int aLengthWidth ) {
return new AllocSegmentHead( aBody, aLengthWidth );
}
/**
* Constructs the head with the given body and with the given number of
* bytes used to specify the body's length and the provided
* {@link Endianess} representation of the body's length.
*
* @param aBody The body referenced by this head.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for length values.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( AllocSegmentBody> aBody, int aLengthWidth, Endianess aEndianess ) {
return new AllocSegmentHead( aBody, aLengthWidth, aEndianess );
}
/**
* Constructs the head with the given body. The configuration attributes are
* taken from the {@link TransmissionMetrics} configuration object, though
* only those attributes are supported which are also supported by the other
* constructors!
*
* @param aBody The body referenced by this head.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( AllocSegmentBody> aBody, TransmissionMetrics aTransmissionMetrics ) {
return new AllocSegmentHead( aBody, aTransmissionMetrics );
}
/**
* Constructs an empty head with a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the body's length and the provided {@link Endianess} representation of
* the body's length.
*
* @param aEndianess The {@link Endianess} to be used for length values.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( Endianess aEndianess ) {
return new AllocSegmentHead( aEndianess );
}
/**
* Constructs an empty head with the given number of bytes used to specify
* the body's length and a {@link TransmissionMetrics#DEFAULT_ENDIANESS}
* endian representation of the body's length.
*
* @param aLengthWidth The width (in bytes) to be used for length values.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( int aLengthWidth ) {
return new AllocSegmentHead( aLengthWidth );
}
/**
* Constructs an empty head with a width of
* {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} bytes used to specify
* the body's length and the provided {@link Endianess} representation of
* the body's length.
*
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for length values.
*
* @return The accordingly created {@link AllocSegmentHead}.
*/
public static AllocSegmentHead allocSegmentHead( int aLengthWidth, Endianess aEndianess ) {
return new AllocSegmentHead( aLengthWidth, aEndianess );
}
/**
* Constructs a {@link SegmentArraySection} with the given {@link Segment}
* elements. {@link Segment} instances for the array are created using the
* provided array's component type.
*
* @param The type of the {@link Segment} elements to be contained
* in this {@link SegmentArraySection}.
* @param aSegmentArray The array containing the according {@link Segment}
* elements.
*
* @return The accordingly created {@link Segment}.
*/
@SuppressWarnings("unchecked")
public static SegmentArraySection arraySection( ARRAY... aSegmentArray ) {
return new SegmentArraySection<>( aSegmentArray );
}
/**
* Constructs a {@link SegmentArraySection} with instances of the array
* being created using the provided {@link Class} instance.
*
* @param The type of the {@link Segment} elements to be contained
* in this {@link SegmentArraySection}.
* @param aSegmentClass The class from which to produce the the fixed length
* {@link Segment} elements.
*
* @return The accordingly created {@link Segment}.
*/
public static SegmentArraySection arraySection( Class aSegmentClass ) {
return new SegmentArraySection<>( aSegmentClass );
}
/**
* Constructs a {@link SegmentArraySection} with instances of the array
* being created using the provided {@link TypeFactory} instance.
*
* @param The type of the {@link Segment} elements to be contained
* in this {@link SegmentArraySection}.
* @param aSegmentFactory The factory producing the the fixed length
* {@link Segment} elements.
*
* @return The accordingly created {@link Segment}.
*/
public static SegmentArraySection arraySection( TypeFactory aSegmentFactory ) {
return new SegmentArraySection<>( aSegmentFactory );
}
/**
* Constructs a {@link SegmentArraySection} with the given elements.
* {@link Segment} instances for the array are created using the provided
* {@link TypeFactory} instance.
*
* @param The type of the {@link Segment} elements to be contained
* in this {@link SegmentArraySection}.
* @param aSegmentFactory The factory producing the the fixed length
* {@link Segment} elements.
* @param aSegmentArray The array containing the according {@link Segment}
* elements.
*
* @return The accordingly created {@link Segment}.
*/
@SuppressWarnings("unchecked")
public static SegmentArraySection arraySection( TypeFactory aSegmentFactory, ARRAY... aSegmentArray ) {
return new SegmentArraySection<>( aSegmentFactory, aSegmentArray );
}
/**
* Constructs an {@link AsciizArraySegment} using the provided
* {@link String} elements and using the provided {@link Segment} class for
* creating {@link Segment} instances.
*
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
* @param aLengthWidth The width (in bytes) to be used for size values
* (number of elements in the payload array). * @param aEndianess The
* {@link Endianess} to be used for size values.
* @param aEndianess the endianess
* @param aPayload The {@link String} elements being contained in this
* instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( byte aEndOfStringByte, int aLengthWidth, Endianess aEndianess, String... aPayload ) {
return new AsciizArraySegment( aEndOfStringByte, aLengthWidth, aEndianess, aPayload );
}
/**
* Constructs an {@link AsciizArraySegment} containing the provided payload
* and using the {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} as well as
* the {@link TransmissionMetrics#DEFAULT_ENDIANESS}.
*
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
* @param aPayload The {@link String} elements being contained in this
* instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( byte aEndOfStringByte, String... aPayload ) {
return new AsciizArraySegment( aEndOfStringByte, aPayload );
}
/**
* Constructs an {@link AsciizArraySegment} using the provided
* {@link String} elements and using the provided {@link Segment} class for
* creating {@link Segment} instances.
*
* @param aLengthWidth The width (in bytes) to be used for size values
* (number of elements in the payload array). * @param aEndianess The
* {@link Endianess} to be used for size values.
* @param aEndianess the endianess
* @param aPayload The {@link String} elements being contained in this
* instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( int aLengthWidth, Endianess aEndianess, String... aPayload ) {
return new AsciizArraySegment( aLengthWidth, aEndianess, aPayload );
}
/**
* Constructs an {@link AsciizArraySegment} containing the provided payload
* and using the {@link TransmissionMetrics#DEFAULT_LENGTH_WIDTH} as well as
* the {@link TransmissionMetrics#DEFAULT_ENDIANESS}.
*
* @param aPayload The {@link String} elements being contained in this
* instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( String... aPayload ) {
return new AsciizArraySegment( aPayload );
}
// ------------------------------------------------------------------------
/**
* Constructs an {@link AsciizArraySegment} using the provided arguments.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
* @param aLengthWidth The width (in bytes) to be used for size values
* (number of elements in the payload array).
* @param aEndianess The {@link Endianess} to be used for size values.
* @param aPayload The {@link String} elements being contained in this
* instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( String aAlias, byte aEndOfStringByte, int aLengthWidth, Endianess aEndianess, String... aPayload ) {
return new AsciizArraySegment( aAlias, aEndOfStringByte, aLengthWidth, aEndianess, aPayload );
}
/**
* Constructs an {@link AsciizArraySegment} using the provided arguments.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aLengthWidth The width (in bytes) to be used for size values
* (number of elements in the payload array).
* @param aEndianess The {@link Endianess} to be used for size values.
* @param aPayload The {@link String} elements being contained in this
* instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( String aAlias, int aLengthWidth, Endianess aEndianess, String... aPayload ) {
return new AsciizArraySegment( aAlias, aLengthWidth, aEndianess, aPayload );
}
/**
* Constructs an {@link AsciizArraySegment} from the given configuration.
* The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( String aAlias, TransmissionMetrics aTransmissionMetrics ) {
return new AsciizArraySegment( aAlias, aTransmissionMetrics );
}
/**
* Constructs an {@link AsciizArraySegment} from the given configuration.
* The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( String aAlias, TransmissionMetrics aTransmissionMetrics, byte aEndOfStringByte ) {
return new AsciizArraySegment( aAlias, aTransmissionMetrics.getEndOfStringByte(), aTransmissionMetrics.getLengthWidth(), aTransmissionMetrics.getEndianess() );
}
/**
* Constructs an {@link AsciizArraySegment} from the given configuration.
* The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link AsciizArraySegment}.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( String aAlias, TransmissionMetrics aTransmissionMetrics, String... aValue ) {
return new AsciizArraySegment( aAlias, aTransmissionMetrics, aValue );
}
/**
* Constructs an {@link AsciizArraySegment} from the given configuration.
* The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( TransmissionMetrics aTransmissionMetrics ) {
return new AsciizArraySegment( aTransmissionMetrics );
}
/**
* Constructs an {@link AsciizArraySegment} from the given configuration.
* The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link AsciizArraySegment}.
*
* @return The accordingly created {@link AsciizArraySegment}.
*/
public static AsciizArraySegment asciizArraySegment( TransmissionMetrics aTransmissionMetrics, String... aValue ) {
return new AsciizArraySegment( aTransmissionMetrics, aValue );
}
/**
* Constructs an empty {@link AsciizSegment}. The serialized content (as of
* {@link AsciizSegment#toSequence()} or
* {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString).
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment() {
return new AsciizSegment();
}
/**
* Constructs an empty {@link AsciizSegment}. The serialized content (as of
* {@link AsciizSegment#toSequence()} or
* {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString).
*
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( byte aEndOfStringByte ) {
return new AsciizSegment( aEndOfStringByte );
}
/**
* Constructs an {@link AsciizSegment} with the given (ASCII encoded) bytes
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the bytes do not contain any 0 ("zero") values as a value of 0
* unintendedly terminates the resulting {@link String}.
*
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( byte[] aValue ) {
return new AsciizSegment( aValue );
}
/**
* Constructs an {@link AsciizSegment} with the given (ASCII encoded) bytes
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the bytes do not contain any 0 ("zero") values as a value of 0
* unintendedly terminates the resulting {@link String}.
*
* @param aValue The payload to be contained by this {@link AsciizSegment}.
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( byte[] aValue, byte aEndOfStringByte ) {
return new AsciizSegment( aValue, aEndOfStringByte );
}
/**
* Constructs an {@link AsciizSegment} with the given {@link String}
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the {@link String} does not contain any 0 ("zero") values as a value
* of 0 unintendedly terminates the resulting {@link String}.
*
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aValue ) {
return new AsciizSegment( aValue );
}
/**
* Constructs an {@link AsciizSegment} with the given {@link String}
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the {@link String} does not contain any 0 ("zero") values as a value
* of 0 unintendedly terminates the resulting {@link String}.
*
* @param aValue The payload to be contained by this {@link AsciizSegment}.
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aValue, byte aEndOfStringByte ) {
return new AsciizSegment( aValue, aEndOfStringByte );
}
/**
* Constructs an {@link AsciizSegment} with the given (ASCII encoded) bytes
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the bytes do not contain any 0 ("zero") values as a value of 0
* unintendedly terminates the resulting {@link String}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aAlias, byte[] aValue ) {
return new AsciizSegment( aAlias, aValue );
}
/**
* Constructs an {@link AsciizSegment} with the given (ASCII encoded) bytes
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the bytes do not contain any 0 ("zero") values as a value of 0
* unintendedly terminates the resulting {@link String}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aAlias, byte[] aValue, byte aEndOfStringByte ) {
return new AsciizSegment( aAlias, aValue, aEndOfStringByte );
}
/**
* Constructs an {@link AsciizSegment} with the given {@link String}
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the {@link String} does not contain any 0 ("zero") values as a value
* of 0 unintendedly terminates the resulting {@link String}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aAlias, String aValue ) {
return new AsciizSegment( aAlias, aValue );
}
/**
* Constructs an {@link AsciizSegment} with the given {@link String}
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the {@link String} does not contain any 0 ("zero") values as a value
* of 0 unintendedly terminates the resulting {@link String}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
* @param aEndOfStringByte The alternate value instead of 0 "zero"
* identifying the end of the string.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aAlias, String aValue, byte aEndOfStringByte ) {
return new AsciizSegment( aAlias, aValue, aEndOfStringByte );
}
/**
* Constructs an {@link AsciizSegment} with the given (ASCII encoded) bytes
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the bytes do not contain any 0 ("zero") values as a value of 0
* unintendedly terminates the resulting {@link String}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aAlias, TransmissionMetrics aTransmissionMetrics, byte[] aValue ) {
return new AsciizSegment( aAlias, aValue, aTransmissionMetrics.getEndOfStringByte() );
}
/**
* Constructs an {@link AsciizSegment} with the given {@link String}
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the {@link String} does not contain any 0 ("zero") values as a value
* of 0 unintendedly terminates the resulting {@link String}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( String aAlias, TransmissionMetrics aTransmissionMetrics, String aValue ) {
return new AsciizSegment( aAlias, aValue, aTransmissionMetrics.getEndOfStringByte() );
}
/**
* Constructs an empty {@link AsciizSegment}. The serialized content (as of
* {@link AsciizSegment#toSequence()} or
* {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString).
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( TransmissionMetrics aTransmissionMetrics ) {
return new AsciizSegment( aTransmissionMetrics.getEndOfStringByte() );
}
/**
* Constructs an {@link AsciizSegment} with the given (ASCII encoded) bytes
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the bytes do not contain any 0 ("zero") values as a value of 0
* unintendedly terminates the resulting {@link String}.
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( TransmissionMetrics aTransmissionMetrics, byte[] aValue ) {
return new AsciizSegment( aValue, aTransmissionMetrics.getEndOfStringByte() );
}
/**
* Constructs an {@link AsciizSegment} with the given {@link String}
* payload. The serialized content (as of {@link AsciizSegment#toSequence()}
* or {@link AsciizSegment#transmitTo(java.io.OutputStream)}) of an
* {@link AsciizSegment} represents an an ASCIIZ {@link String} (also known
* as CString). It is in the responsibility of the programmer to make sure
* that the {@link String} does not contain any 0 ("zero") values as a value
* of 0 unintendedly terminates the resulting {@link String}.
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by this {@link AsciizSegment}.
*
* @return The accordingly created {@link AsciizSegment}.
*/
public static AsciizSegment asciizSegment( TransmissionMetrics aTransmissionMetrics, String aValue ) {
return new AsciizSegment( aValue, aTransmissionMetrics.getEndOfStringByte() );
}
/**
* Enriches the provided {@link Section} with the given magic bytes being
* prefixed. Enforces the configured magic bytes to match the received magic
* bytes (as of
* {@link AssertMagicBytesSectionDecorator#fromTransmission(Sequence, int, int)}
* and
* {@link AssertMagicBytesSectionDecorator#receiveFrom(java.io.InputStream, int, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Section} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Section} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The magic bytes to be prefixed.
*
* @return The accordingly created {@link AssertMagicBytesSectionDecorator}.
*/
public static AssertMagicBytesSectionDecorator assertMagicBytesSection( DECORATEE aDecoratee, byte... aMagicBytes ) {
return new AssertMagicBytesSectionDecorator<>( aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Section} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSectionDecorator#fromTransmission(Sequence, int, int)}
* and
* {@link AssertMagicBytesSectionDecorator#receiveFrom(java.io.InputStream, int, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Section} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Section} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes (uses the
* {@link TransmissionMetrics#DEFAULT_ENCODING}) for byte
* conversion).
*
* @return The accordingly created {@link AssertMagicBytesSectionDecorator}.
*/
public static AssertMagicBytesSectionDecorator assertMagicBytesSection( DECORATEE aDecoratee, String aMagicBytes ) {
return new AssertMagicBytesSectionDecorator<>( aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Section} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSectionDecorator#fromTransmission(Sequence, int, int)}
* and
* {@link AssertMagicBytesSectionDecorator#receiveFrom(java.io.InputStream, int, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Section} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Section} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aCharset The {@link Charset} to use when converting the
* {@link String} to a byte array.
*
* @return The accordingly created {@link AssertMagicBytesSectionDecorator}.
*/
public static AssertMagicBytesSectionDecorator assertMagicBytesSection( DECORATEE aDecoratee, String aMagicBytes, Charset aCharset ) {
return new AssertMagicBytesSectionDecorator<>( aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Section} with the given magic bytes being
* prefixed. Enforces the configured magic bytes to match the received magic
* bytes (as of
* {@link AssertMagicBytesSectionDecorator#fromTransmission(Sequence, int, int)}
* and
* {@link AssertMagicBytesSectionDecorator#receiveFrom(java.io.InputStream, int, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Section} type describing the
* {@link Segment} subclass decoratee.
* @param aAlias The alias which identifies the content of this segment.
* @param aDecoratee The {@link Section} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The magic bytes to be prefixed.
*
* @return The accordingly created {@link AssertMagicBytesSectionDecorator}.
*/
public static AssertMagicBytesSectionDecorator assertMagicBytesSection( String aAlias, DECORATEE aDecoratee, byte... aMagicBytes ) {
return new AssertMagicBytesSectionDecorator<>( aAlias, aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Section} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSectionDecorator#fromTransmission(Sequence, int, int)}
* and
* {@link AssertMagicBytesSectionDecorator#receiveFrom(java.io.InputStream, int, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Section} type describing the
* {@link Segment} subclass decoratee.
* @param aAlias The alias which identifies the content of this segment.
* @param aDecoratee The {@link Section} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes (uses the
* {@link TransmissionMetrics#DEFAULT_ENCODING}) for byte
* conversion).
*
* @return The accordingly created {@link AssertMagicBytesSectionDecorator}.
*/
public static AssertMagicBytesSectionDecorator assertMagicBytesSection( String aAlias, DECORATEE aDecoratee, String aMagicBytes ) {
return new AssertMagicBytesSectionDecorator<>( aAlias, aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Section} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSectionDecorator#fromTransmission(Sequence, int, int)}
* and
* {@link AssertMagicBytesSectionDecorator#receiveFrom(java.io.InputStream, int, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Section} type describing the
* {@link Segment} subclass decoratee.
* @param aAlias The alias which identifies the content of this segment.
* @param aDecoratee The {@link Section} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aCharset The {@link Charset} to use when converting the
* {@link String} to a byte array.
*
* @return The accordingly created {@link AssertMagicBytesSectionDecorator}.
*/
public static AssertMagicBytesSectionDecorator assertMagicBytesSection( String aAlias, DECORATEE aDecoratee, String aMagicBytes, Charset aCharset ) {
return new AssertMagicBytesSectionDecorator<>( aAlias, aDecoratee, aMagicBytes );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes. Enforces the configured magic bytes to match the received
* magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aMagicBytes The magic bytes to be stored by this instance.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( byte... aMagicBytes ) {
return new AssertMagicBytesSegment( aMagicBytes );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes. Enforces the configured magic bytes to match the received
* magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aMagicBytes The bytes to be stored by this instance as magic
* bytes.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( byte[] aMagicBytes, TransmissionMetrics aTransmissionMetrics ) {
return new AssertMagicBytesSegment( aMagicBytes, aTransmissionMetrics );
}
/**
* Enriches the provided {@link Segment} with the given magic bytes being
* prefixed. Enforces the configured magic bytes to match the received magic
* bytes (as of {@link AssertMagicBytesSegment#fromTransmission(Sequence)}
* and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The magic bytes to be prefixed.
*
* @return The accordingly created {@link AssertMagicBytesSegmentDecorator}.
*/
public static AssertMagicBytesSegmentDecorator assertMagicBytesSegment( DECORATEE aDecoratee, byte... aMagicBytes ) {
return new AssertMagicBytesSegmentDecorator<>( aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Segment} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegmentDecorator#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegmentDecorator#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes (uses the
* {@link TransmissionMetrics#DEFAULT_ENCODING}) for byte
* conversion).
*
* @return The accordingly created {@link AssertMagicBytesSegmentDecorator}.
*/
public static AssertMagicBytesSegmentDecorator assertMagicBytesSegment( DECORATEE aDecoratee, String aMagicBytes ) {
return new AssertMagicBytesSegmentDecorator<>( aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Segment} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegmentDecorator#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegmentDecorator#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aCharset The {@link Charset} to use when converting the
* {@link String} to a byte array.
*
* @return The accordingly created {@link AssertMagicBytesSegmentDecorator}.
*/
public static AssertMagicBytesSegmentDecorator assertMagicBytesSegment( DECORATEE aDecoratee, String aMagicBytes, Charset aCharset ) {
return new AssertMagicBytesSegmentDecorator<>( aDecoratee, aMagicBytes );
}
/**
* Constructs an {@link AssertMagicBytesSegment} with the according magic
* bytes (retrieved from the given {@link String}). Enforces the configured
* magic bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes (uses the
* {@link TransmissionMetrics#DEFAULT_ENCODING}) for byte
* conversion).
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aMagicBytes ) {
return new AssertMagicBytesSegment( aMagicBytes );
}
/**
* Constructs an {@link AssertMagicBytesSegment} with the according magic
* bytes. Enforces the configured magic bytes to match the received magic
* bytes (as of {@link AssertMagicBytesSegment#fromTransmission(Sequence)}
* and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aMagicBytes The magic bytes to be stored by this instance.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aAlias, byte... aMagicBytes ) {
return new AssertMagicBytesSegment( aAlias, aMagicBytes );
}
/**
* Constructs an {@link AssertMagicBytesSegment} with the according magic
* bytes. Enforces the configured magic bytes to match the received magic
* bytes (as of {@link AssertMagicBytesSegment#fromTransmission(Sequence)}
* and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aMagicBytes The bytes to be stored by this instance as magic
* bytes.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aAlias, byte[] aMagicBytes, TransmissionMetrics aTransmissionMetrics ) {
return new AssertMagicBytesSegment( aAlias, aMagicBytes, aTransmissionMetrics );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes (retrieved from the given {@link String}). Enforces the
* configured magic bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aCharset The {@link Charset} to use when converting the
* {@link String} to a byte array.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aMagicBytes, Charset aCharset ) {
return new AssertMagicBytesSegment( aMagicBytes, aCharset );
}
/**
* Enriches the provided {@link Segment} with the given magic bytes being
* prefixed.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee. Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegmentDecorator#fromTransmission(Sequence)}
* and
* {@link AssertMagicBytesSegmentDecorator#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes
* fails during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
* @param aAlias The alias which identifies the content of this segment.
* @param aDecoratee The {@link Segment} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The magic bytes to be prefixed.
*
* @return The accordingly created {@link AssertMagicBytesSegmentDecorator}.
*/
public static AssertMagicBytesSegmentDecorator assertMagicBytesSegment( String aAlias, DECORATEE aDecoratee, byte... aMagicBytes ) {
return new AssertMagicBytesSegmentDecorator<>( aAlias, aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Segment} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegmentDecorator#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegmentDecorator#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aAlias The alias which identifies the content of this segment.
* @param aDecoratee The {@link Segment} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes (uses the
* {@link TransmissionMetrics#DEFAULT_ENCODING}) for byte
* conversion).
*
* @return The accordingly created {@link AssertMagicBytesSegmentDecorator}.
*/
public static AssertMagicBytesSegmentDecorator assertMagicBytesSegment( String aAlias, DECORATEE aDecoratee, String aMagicBytes ) {
return new AssertMagicBytesSegmentDecorator<>( aAlias, aDecoratee, aMagicBytes );
}
/**
* Enriches the provided {@link Segment} with magic bytes being prefixed
* (retrieved from the given {@link String}). Enforces the configured magic
* bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegmentDecorator#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegmentDecorator#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aAlias The alias which identifies the content of this segment.
* @param aDecoratee The {@link Segment} which is to be prefixed with magic
* bytes.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aCharset The {@link Charset} to use when converting the
* {@link String} to a byte array.
*
* @return The accordingly created {@link AssertMagicBytesSegmentDecorator}.
*/
public static AssertMagicBytesSegmentDecorator assertMagicBytesSegment( String aAlias, DECORATEE aDecoratee, String aMagicBytes, Charset aCharset ) {
return new AssertMagicBytesSegmentDecorator<>( aAlias, aDecoratee, aMagicBytes );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes (retrieved from the given {@link String}). Enforces the
* configured magic bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes (uses the
* {@link TransmissionMetrics#DEFAULT_ENCODING}) for byte
* conversion).
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aAlias, String aMagicBytes ) {
return new AssertMagicBytesSegment( aAlias, aMagicBytes );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes (retrieved from the given {@link String}). Enforces the
* configured magic bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aCharset The {@link Charset} to use when converting the
* {@link String} to a byte array.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aAlias, String aMagicBytes, Charset aCharset ) {
return new AssertMagicBytesSegment( aAlias, aMagicBytes, aCharset );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes (retrieved from the given {@link String}). Enforces the
* configured magic bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aAlias, String aMagicBytes, TransmissionMetrics aTransmissionMetrics ) {
return new AssertMagicBytesSegment( aAlias, aMagicBytes, aTransmissionMetrics );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes (retrieved from the given {@link String}). Enforces the
* configured magic bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( TransmissionMetrics aTransmissionMetrics ) {
return new AssertMagicBytesSegment( aTransmissionMetrics );
}
/**
* Constructs an {@link AbstractMagicBytesTransmission} with the according
* magic bytes (retrieved from the given {@link String}). Enforces the
* configured magic bytes to match the received magic bytes (as of
* {@link AssertMagicBytesSegment#fromTransmission(Sequence)} and
* {@link AssertMagicBytesSegment#receiveFrom(java.io.InputStream, java.io.OutputStream)}
* or the like). In case the assertion of the configured magic bytes fails
* during receiving, then a {@link BadMagicBytesException} or
* {@link BadMagicBytesSequenceException} is thrown.
*
* @param aMagicBytes The {@link String} to be stored by this instance as
* magic bytes.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link AssertMagicBytesSegment}.
*/
public static AssertMagicBytesSegment assertMagicBytesSegment( String aMagicBytes, TransmissionMetrics aTransmissionMetrics ) {
return new AssertMagicBytesSegment( aMagicBytes, aTransmissionMetrics );
}
/**
* Constructs an empty {@link BooleanArraySection}.
*
* @return The empty {@link BooleanArraySection}.
*/
public static BooleanArraySection booleanArraySection() {
return new BooleanArraySection();
}
/**
* Constructs a {@link BooleanArraySection} with the given boolean array
* payload.
*
* @param aValue The array (payload) to be contained by the
* {@link BooleanArraySection}.
*
* @return The accordingly created {@link BooleanArraySection}.
*/
public static BooleanArraySection booleanArraySection( boolean... aValue ) {
return new BooleanArraySection( aValue );
}
/**
* Constructs a {@link BooleanArraySection} with the given boolean array
* payload.
*
* @param aValue The array (payload) to be contained by the
* {@link BooleanArraySection}.
*
* @return The accordingly created {@link BooleanArraySection}.
*/
public static BooleanArraySection booleanArraySection( Boolean... aValue ) {
return new BooleanArraySection( aValue );
}
/**
* Constructs an empty {@link BooleanArraySection}.
*
* @param aAlias The alias which identifies the content of this segment.
*
* @return The empty {@link BooleanArraySection}.
*/
public static BooleanArraySection booleanArraySection( String aAlias ) {
return new BooleanArraySection( aAlias );
}
/**
* Constructs a {@link BooleanArraySection} with the given boolean array
* payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The array (payload) to be contained by the
* {@link BooleanArraySection}.
*
* @return The accordingly created {@link BooleanArraySection}.
*/
public static BooleanArraySection booleanArraySection( String aAlias, boolean... aValue ) {
return new BooleanArraySection( aAlias, aValue );
}
/**
* Constructs a {@link BooleanArraySection} with the given boolean array
* payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The array (payload) to be contained by the
* {@link BooleanArraySection}.
*
* @return The accordingly created {@link BooleanArraySection}.
*/
public static BooleanArraySection booleanArraySection( String aAlias, Boolean... aValue ) {
return new BooleanArraySection( aAlias, aValue );
}
/**
* Constructs an empty {@link BooleanSegment}.
*
* @return The accordingly created {@link BooleanSegment}.
*/
public static BooleanSegment booleanSegment() {
return new BooleanSegment();
}
/**
* Constructs a {@link BooleanSegment} with the given boolean payload.
*
* @param aValue The value (payload) to be contained by the
* {@link BooleanSegment}.
*
* @return The accordingly created {@link BooleanSegment}.
*/
public static BooleanSegment booleanSegment( boolean aValue ) {
return new BooleanSegment( aValue );
}
/**
* Constructs an empty {@link BooleanSegment}.
*
* @param aAlias The alias which identifies the content of this segment.
*
* @return The accordingly created {@link BooleanSegment}.
*/
public static BooleanSegment booleanSegment( String aAlias ) {
return new BooleanSegment( aAlias );
}
/**
* Constructs a {@link BooleanSegment} with the given boolean payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The value (payload) to be contained by the
* {@link BooleanSegment}.
*
* @return The accordingly created {@link BooleanSegment}.
*/
public static BooleanSegment booleanSegment( String aAlias, boolean aValue ) {
return new BooleanSegment( aAlias, aValue );
}
/**
* Constructs a {@link BreakerSectionDecorator} instance with the given
* decoratee breaking deserialization of the decorated segments by the given
* number of times. After the total number of breaking the decoratee has
* been reached, the decorator behaves transparent (it just delegates
* without breaking the decoratee any more). This is good to see if a retry
* mechanism works when using some kind of error correction segment.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The decoratee to be contained by this facade.
* @param aBreakNumber The number of times to break deserialization.
*
* @return The accordingly created {@link BreakerSectionDecorator}.
*/
public static BreakerSectionDecorator breakerSection( DECORATEE aDecoratee, int aBreakNumber ) {
return new BreakerSectionDecorator<>( aDecoratee, aBreakNumber );
}
/**
* Constructs a {@link BreakerSegmentDecorator} instance with the given
* decoratee breaking deserialization of the decorated segments by the given
* number of times. After the total number of breaking the decoratee has
* been reached, the decorator behaves transparent (it just delegates
* without breaking the decoratee any more). This is good to see if a retry
* mechanism works when using some kind of error correction segment.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The decoratee to be contained by this facade.
* @param aBreakNumber The number of times to break deserialization.
*
* @return The accordingly created {@link BreakerSegmentDecorator}.
*/
public static BreakerSegmentDecorator breakerSegment( DECORATEE aDecoratee, int aBreakNumber ) {
return new BreakerSegmentDecorator<>( aDecoratee, aBreakNumber );
}
/**
* Constructs an empty {@link ByteArraySection}.
*
* @return The empty {@link ByteArraySection}.
*/
public static ByteArraySection byteArraySection() {
return new ByteArraySection();
}
/**
* Constructs a {@link ByteArraySection} with the given byte array payload.
*
* @param aArray The array (payload) to be contained by the
* {@link ByteArraySection}.
*
* @return The accordingly created {@link ByteArraySection}.
*/
public static ByteArraySection byteArraySection( byte... aArray ) {
return new ByteArraySection( aArray );
}
/**
* Constructs a {@link ByteArraySection} with the given byte array payload.
*
* @param aArray The array (payload) to be contained by the
* {@link ByteArraySection}.
*
* @return The accordingly created {@link ByteArraySection}.
*/
public static ByteArraySection byteArraySection( Byte... aArray ) {
return new ByteArraySection( aArray );
}
/**
* Constructs an empty {@link ByteArraySection}.
*
* @param aAlias The alias which identifies the content of this segment.
*
* @return The empty {@link ByteArraySection}.
*/
public static ByteArraySection byteArraySection( String aAlias ) {
return new ByteArraySection( aAlias );
}
/**
* Constructs a {@link ByteArraySection} with the given byte array payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aArray The array (payload) to be contained by the
* {@link ByteArraySection}.
*
* @return The accordingly created {@link ByteArraySection}.
*/
public static ByteArraySection byteArraySection( String aAlias, byte... aArray ) {
return new ByteArraySection( aAlias, aArray );
}
/**
* Constructs a {@link ByteArraySection} with the given byte array payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aArray The array (payload) to be contained by the
* {@link ByteArraySection}.
*
* @return The accordingly created {@link ByteArraySection}.
*/
public static ByteArraySection byteArraySection( String aAlias, Byte... aArray ) {
return new ByteArraySection( aAlias, aArray );
}
/**
* Constructs an empty {@link ByteSegment}.
*
* @return The accordingly created {@link ByteSegment}.
*/
public static ByteSegment byteSegment() {
return new ByteSegment();
}
/**
* Constructs a {@link ByteSegment} with the given byte payload.
*
* @param aValue The value (payload) to be contained by the
* {@link ByteSegment}.
*
* @return The accordingly created {@link ByteSegment}.
*/
public static ByteSegment byteSegment( Byte aValue ) {
return new ByteSegment( aValue );
}
/**
* Constructs an empty {@link ByteSegment}.
*
* @param aAlias The alias which identifies the content of this segment.
*
* @return The accordingly created {@link ByteSegment}.
*/
public static ByteSegment byteSegment( String aAlias ) {
return new ByteSegment( aAlias );
}
/**
* Constructs a {@link ByteSegment} with the given byte payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The value (payload) to be contained by the
* {@link ByteSegment}.
*
* @return The accordingly created {@link ByteSegment}.
*/
public static ByteSegment byteSegment( String aAlias, Byte aValue ) {
return new ByteSegment( aAlias, aValue );
}
/**
* Constructs an empty {@link CharArraySection}. Uses UTF-16 by default for
* encoding.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection() {
return new CharArraySection();
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( char[] aPayload ) {
return new CharArraySection( aPayload );
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( Character[] aPayload ) {
return new CharArraySection( aPayload );
}
/**
* Constructs an empty {@link CharArraySection}.
*
* @param aCharset The charset to be used for encoding.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( Charset aCharset ) {
return new CharArraySection( aCharset );
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aCharset The charset to be used for encoding.
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( Charset aCharset, char... aPayload ) {
return new CharArraySection( aCharset, aPayload );
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aCharset The charset to be used for encoding.
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( Charset aCharset, Character... aPayload ) {
return new CharArraySection( aCharset, aPayload );
}
/**
* Constructs an empty {@link CharArraySection}. Uses UTF-16 by default for
* encoding.
*
* @param aAlias The alias which identifies the content of this segment.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias ) {
return new CharArraySection( aAlias );
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, char[] aPayload ) {
return new CharArraySection( aAlias, aPayload );
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, Character[] aPayload ) {
return new CharArraySection( aAlias, aPayload );
}
/**
* Constructs an empty {@link CharArraySection}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aCharset The charset to be used for encoding.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, Charset aCharset ) {
return new CharArraySection( aAlias, aCharset );
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aCharset The charset to be used for encoding.
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, Charset aCharset, char... aPayload ) {
return new CharArraySection( aAlias, aCharset, aPayload );
}
/**
* Constructs a {@link CharArraySection} with the given char array payload.
* Uses {@link TransmissionMetrics#DEFAULT_ENCODING} by default for
* encoding.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aCharset The charset to be used for encoding.
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, Charset aCharset, Character... aPayload ) {
return new CharArraySection( aAlias, aCharset, aPayload );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics ) {
return new CharArraySection( aAlias, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link CharArraySection}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics, char... aValue ) {
return new CharArraySection( aAlias, aTransmissionMetrics.getEncoding(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link CharArraySection}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( String aAlias, TransmissionMetrics aTransmissionMetrics, Character... aValue ) {
return new CharArraySection( aAlias, aTransmissionMetrics.getEncoding(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( TransmissionMetrics aTransmissionMetrics ) {
return new CharArraySection( aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link CharArraySection}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( TransmissionMetrics aTransmissionMetrics, char... aValue ) {
return new CharArraySection( aTransmissionMetrics.getEncoding(), aValue );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aValue The payload to be contained by the
* {@link CharArraySection}.
*
* @return The accordingly created {@link CharArraySection}.
*/
public static CharArraySection charArraySection( TransmissionMetrics aTransmissionMetrics, Character... aValue ) {
return new CharArraySection( aTransmissionMetrics.getEncoding(), aValue );
}
/**
* Constructs an empty {@link CharSection}.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection() {
return new CharSection( (char) 0 );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( char aValue, TransmissionMetrics aTransmissionMetrics ) {
return new CharSection( aValue, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs a {@link CharSection} with the given char payload.
*
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( Character aPayload ) {
return new CharSection( aPayload );
}
/**
* Constructs a {@link CharSection} with the given char payload and the
* given {@link Charset}.
*
* @param aPayload The payload to be contained by the {@link Section}.
* @param aCharset The charset to be used for encoding.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( Character aPayload, Charset aCharset ) {
return new CharSection( aPayload, aCharset );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( Character aValue, TransmissionMetrics aTransmissionMetrics ) {
return new CharSection( aValue, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an empty {@link CharSection} with the given {@link Charset}.
*
* @param aCharset The charset to be used for encoding.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( Charset aCharset ) {
return new CharSection( (char) 0, aCharset );
}
/**
* Constructs an empty {@link CharSection}.
*
* @param aAlias The alias which identifies the content of this segment.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( String aAlias ) {
return new CharSection( aAlias, (char) 0 );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( String aAlias, char aValue, TransmissionMetrics aTransmissionMetrics ) {
return new CharSection( aAlias, aValue, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs a {@link CharSection} with the given char payload.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( String aAlias, Character aPayload ) {
return new CharSection( aAlias, aPayload );
}
/**
* Constructs a {@link CharSection} with the given char payload and the
* given {@link Charset}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aPayload The payload to be contained by the {@link Section}.
* @param aCharset The charset to be used for encoding.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( String aAlias, Character aPayload, Charset aCharset ) {
return new CharSection( aAlias, aPayload, aCharset );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aValue The payload to be contained by the {@link CharSection}.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( String aAlias, Character aValue, TransmissionMetrics aTransmissionMetrics ) {
return new CharSection( aAlias, aValue, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an empty {@link CharSection} with the given {@link Charset}.
*
* @param aAlias The alias which identifies the content of this segment.
* @param aCharset The charset to be used for encoding.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( String aAlias, Charset aCharset ) {
return new CharSection( aAlias, (char) 0, aCharset );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aAlias The alias which identifies the content of this instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( String aAlias, TransmissionMetrics aTransmissionMetrics ) {
return new CharSection( aAlias, aTransmissionMetrics.getEncoding() );
}
/**
* Constructs an according instance from the given configuration. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CharSection}.
*/
public static CharSection charSection( TransmissionMetrics aTransmissionMetrics ) {
return new CharSection( aTransmissionMetrics.getEncoding() );
}
/**
* Constructs a {@link Cipher} transmission decorator applying bijective and
* inverse functions upon the delegated methods.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The decorator having applied the {@link Cipher}'s
* {@link BijectiveFunction} to encode and the {@link Cipher}'s
* {@link InverseFunction} to decode any data being delegated.
* @param aCipher The {@link Cipher} providing the {@link BijectiveFunction}
* to encode and the {@link Cipher}'s {@link InverseFunction} to
* decode any data being delegated.
*
* @return The accordingly created {@link CipherSectionDecorator}.
*/
public static CipherSectionDecorator cipherSection( DECORATEE aDecoratee, Cipher aCipher ) {
return new CipherSectionDecorator<>( aDecoratee, aCipher );
}
/**
* Constructs a {@link Cipher} transmission decorator applying bijective and
* inverse functions upon the delegated methods.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The decorator having applied the {@link Cipher}'s
* {@link BijectiveFunction} to encode and the {@link Cipher}'s
* {@link InverseFunction} to decode any data being delegated.
* @param aCipher The {@link Cipher} providing the {@link BijectiveFunction}
* to encode and the {@link Cipher}'s {@link InverseFunction} to
* decode any data being delegated.
*
* @return The accordingly created {@link CipherSegmentDecorator}.
*/
public static CipherSegmentDecorator cipherSegment( DECORATEE aDecoratee, Cipher aCipher ) {
return new CipherSegmentDecorator<>( aDecoratee, aCipher );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed all and in alphabetical order. For specifying a predefined set
* of attributes and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aType The data structure's type.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( Class aType ) {
return new ComplexTypeSegment<>( aType );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed all and in alphabetical order. For specifying a predefined set
* of attributes and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aType The data structure's type.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( Class aType, int aLengthWidth, Endianess aEndianess, Charset aCharset ) {
return new ComplexTypeSegment<>( aType, aLengthWidth, aEndianess, aCharset );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed using the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aType The data structure's type.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( Class aType, int aLengthWidth, Endianess aEndianess, Charset aCharset, String... aAttributes ) {
return new ComplexTypeSegment<>( aType, aLengthWidth, aEndianess, aCharset, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed using the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aType The data structure's type.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( Class aType, String... aAttributes ) {
return new ComplexTypeSegment<>( aType, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed all and in alphabetical order. For specifying a predefined set
* of attributes and their order, please invoke
* {@link #complexTypeSegment(Class, TransmissionMetrics, String[])}
* instead! The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param The type of the data structure representing the body.
* @param aType The data structure's type.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( Class aType, TransmissionMetrics aTransmissionMetrics ) {
return new ComplexTypeSegment<>( aType, aTransmissionMetrics );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed using the predefined set of attributes in their according order
* The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param The type of the data structure representing the body.
* @param aType The data structure's type.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( Class aType, TransmissionMetrics aTransmissionMetrics, String... aAttributes ) {
return new ComplexTypeSegment<>( aType, aTransmissionMetrics, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed all and in alphabetical order. For specifying a predefined set
* of attributes and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aType The data structure's type.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, Class aType ) {
return new ComplexTypeSegment<>( aAlias, aType );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed all and in alphabetical order. For specifying a predefined set
* of attributes and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aType The data structure's type.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, Class aType, int aLengthWidth, Endianess aEndianess, Charset aCharset ) {
return new ComplexTypeSegment<>( aAlias, aType, aLengthWidth, aEndianess, aCharset );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed using the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aType The data structure's type.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, Class aType, int aLengthWidth, Endianess aEndianess, Charset aCharset, String... aAttributes ) {
return new ComplexTypeSegment<>( aAlias, aType, aLengthWidth, aEndianess, aCharset, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given {@link Class} for
* the according type T. The attributes of the given data structure are
* processed using the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aType The data structure's type.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, Class aType, String... aAttributes ) {
return new ComplexTypeSegment<>( aAlias, aType, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance. The attributes of the given data structure are processed all
* and in alphabetical order. For specifying a predefined set of attributes
* and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The data structure instance.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, T aValue ) {
return new ComplexTypeSegment<>( aAlias, aValue );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance. The attributes of the given data structure are processed all
* and in alphabetical order. For specifying a predefined set of attributes
* and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The data structure instance.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, T aValue, int aLengthWidth, Endianess aEndianess, Charset aCharset ) {
return new ComplexTypeSegment<>( aAlias, aValue, aLengthWidth, aEndianess, aCharset );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance.. The attributes of the given data structure are processed using
* the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The value from which to construct the complex type..
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, T aValue, int aLengthWidth, Endianess aEndianess, Charset aCharset, String... aAttributes ) {
return new ComplexTypeSegment<>( aAlias, aValue, aLengthWidth, aEndianess, aCharset, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance.. The attributes of the given data structure are processed using
* the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aAlias The alias which identifies the content of this segment.
* @param aValue The value from which to construct the complex type..
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( String aAlias, T aValue, String... aAttributes ) {
return new ComplexTypeSegment<>( aAlias, aValue, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance. The attributes of the given data structure are processed all
* and in alphabetical order. For specifying a predefined set of attributes
* and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aValue The data structure instance.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( T aValue ) {
return new ComplexTypeSegment<>( aValue );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance. The attributes of the given data structure are processed all
* and in alphabetical order. For specifying a predefined set of attributes
* and their order, please invoke
* {@link #complexTypeSegment(Class, String[])} instead!
*
* @param The type of the data structure representing the body.
* @param aValue The data structure instance.
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( T aValue, int aLengthWidth, Endianess aEndianess, Charset aCharset ) {
return new ComplexTypeSegment<>( aValue, aLengthWidth, aEndianess, aCharset );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance.. The attributes of the given data structure are processed using
* the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aValue The value from which to construct the complex type..
* @param aLengthWidth The width (in bytes) to be used for length values.
* @param aEndianess The {@link Endianess} to be used for (length) values.
* @param aCharset The {@link Charset} to be used for encoding and decoding
* {@link String} instances.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( T aValue, int aLengthWidth, Endianess aEndianess, Charset aCharset, String... aAttributes ) {
return new ComplexTypeSegment<>( aValue, aLengthWidth, aEndianess, aCharset, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance.. The attributes of the given data structure are processed using
* the predefined set of attributes in their according order
*
* @param The type of the data structure representing the body.
* @param aValue The value from which to construct the complex type..
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( T aValue, String... aAttributes ) {
return new ComplexTypeSegment<>( aValue, aAttributes );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance. The attributes of the given data structure are processed all
* and in alphabetical order. For specifying a predefined set of attributes
* and their order, please invoke
* {@link #complexTypeSegment(Class, TransmissionMetrics, String[])}
* instead! The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param The type of the data structure representing the body.
* @param aValue The data structure instance.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( T aValue, TransmissionMetrics aTransmissionMetrics ) {
return new ComplexTypeSegment<>( aValue, aTransmissionMetrics );
}
/**
* Constructs a {@link ComplexTypeSegment} from the given data structure
* instance.. The attributes of the given data structure are processed using
* the predefined set of attributes in their according order. The
* configuration attributes are taken from the {@link TransmissionMetrics}
* configuration object, though only those attributes are supported which
* are also supported by the other constructors!
*
* @param The type of the data structure representing the body.
* @param aValue The value from which to construct the complex type..
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
* @param aAttributes The attributes or null if all attributes are to be
* processed in alphabetical order.
*
* @return The accordingly created {@link ComplexTypeSegment}.
*/
public static ComplexTypeSegment complexTypeSegment( T aValue, TransmissionMetrics aTransmissionMetrics, String... aAttributes ) {
return new ComplexTypeSegment<>( aValue, aTransmissionMetrics, aAttributes );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcPrefixSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, ConcatenateMode.PREPEND );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcPrefixSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, aChecksumValidationMode );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section}.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcPrefixSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode, Endianess aEndianess ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, ConcatenateMode.PREPEND, aChecksumValidationMode, aEndianess );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section}.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcPrefixSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, Endianess aEndianess ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, ConcatenateMode.PREPEND, aEndianess );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcPrefixSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, ConcatenateMode.PREPEND );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcPrefixSegment( DECORATEE aDecoratee, TransmissionMetrics aTransmissionMetrics ) {
return new CrcSegmentDecorator<>( aDecoratee, aTransmissionMetrics );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcPrefixSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, ConcatenateMode.PREPEND, aChecksumValidationMode );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment}.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcPrefixSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ChecksumValidationMode aChecksumValidationMode, Endianess aEndianess ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, ConcatenateMode.PREPEND, aChecksumValidationMode, aEndianess );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment}.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcPrefixSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, Endianess aEndianess ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, ConcatenateMode.PREPEND, aEndianess );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode, ChecksumValidationMode aChecksumValidationMode ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode, aChecksumValidationMode );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section}.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode, ChecksumValidationMode aChecksumValidationMode, Endianess aEndianess ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode, aChecksumValidationMode, aEndianess );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section}.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcSection( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode, Endianess aEndianess ) {
return new CrcSectionDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode, aEndianess );
}
/**
* Constructs a {@link CrcSectionDecorator} wrapping the given
* {@link Section}.
*
* @param The {@link Section} type describing the
* {@link Section} subclass decoratee.
* @param aDecoratee The {@link Section} to be wrapped.
* @param aTransmissionMetrics The {@link TransmissionMetrics} to be used
* for configuring this instance.
*
* @return The accordingly created {@link CrcSectionDecorator}.
*/
public static CrcSectionDecorator crcSection( DECORATEE aDecoratee, TransmissionMetrics aTransmissionMetrics ) {
return new CrcSectionDecorator<>( aDecoratee, aTransmissionMetrics );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment} (using {@link TransmissionMetrics#DEFAULT_ENDIANESS} by
* default).
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode, ChecksumValidationMode aChecksumValidationMode ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode, aChecksumValidationMode );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment}.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
* @param aChecksumValidationMode The mode of operation when validating
* provided CRC checksums against calculated ones.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode, ChecksumValidationMode aChecksumValidationMode, Endianess aEndianess ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode, aChecksumValidationMode, aEndianess );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment}.
*
* @param The {@link Segment} type describing the
* {@link Segment} subclass decoratee.
* @param aDecoratee The {@link Segment} to be wrapped.
* @param aCrcAlgorithm The {@link CrcAlgorithm} to be used for CRC checksum
* calculation.
* @param aCrcChecksumConcatenateMode The mode of concatenation to use when
* concatenating the CRC checksum with the transmission's
* {@link Sequence}.
* @param aEndianess The {@link Endianess} to use when calculating the CRC
* checksum.
*
* @return The accordingly created {@link CrcSegmentDecorator}.
*/
public static CrcSegmentDecorator crcSegment( DECORATEE aDecoratee, CrcAlgorithm aCrcAlgorithm, ConcatenateMode aCrcChecksumConcatenateMode, Endianess aEndianess ) {
return new CrcSegmentDecorator<>( aDecoratee, aCrcAlgorithm, aCrcChecksumConcatenateMode, aEndianess );
}
/**
* Constructs a {@link CrcSegmentDecorator} wrapping the given
* {@link Segment}. The configuration attributes are taken from the
* {@link TransmissionMetrics} configuration object, though only those
* attributes are supported which are also supported by the other
* constructors!
*
* @param