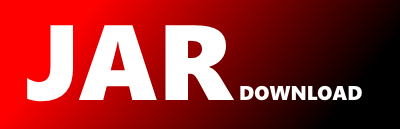
org.refcodes.servicebus.alt.spring.SpringServiceLookupImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-servicebus-alt-spring Show documentation
Show all versions of refcodes-servicebus-alt-spring Show documentation
Artifact for Spring dependency injection implementations of (parts of)
the refcodes-servicebus artifact.
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.servicebus.alt.spring;
import java.net.MalformedURLException;
import java.net.URI;
import java.util.Set;
import org.refcodes.configuration.Properties;
import org.refcodes.factory.CollectionFactory;
import org.refcodes.factory.TypeLookupFactory;
import org.refcodes.factory.alt.spring.SpringBeanFactoryImpl;
import org.refcodes.servicebus.Service;
import org.refcodes.servicebus.ServiceDescriptor;
import org.refcodes.servicebus.ServiceLookupImpl;
/**
* The Class SpringServiceLookupImpl.
*
* @param the generic type
* @param the generic type
*/
public abstract class SpringServiceLookupImpl, SCTX> extends ServiceLookupImpl {
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a factory with the given Spring configuration files.
*
* @param aType The actual type (class) of the {@link ServiceDescriptor}
* instances to be looked up.
*
* @param aConfigurationFiles The URIs of the configuration files describing
* the handled Spring application context.
*
* @throws MalformedURLException If a given URI is malformed.
*/
public SpringServiceLookupImpl( Class> aType, URI[] aConfigurationFiles ) throws MalformedURLException {
super( new SpringFactoryWrapper>( aType, new SpringBeanFactoryImpl( aConfigurationFiles ) ) );
}
/**
* Creates a factory with the given Spring configuration files. If the
* configuration files contains placeholder (e.g. ${jdbc.url}), the given
* property files are used to replace them. The provided property files have
* to follow the JAVA properties scheme (key=value).
*
* @param aType The actual type (class) of the {@link ServiceDescriptor}
* instances to be looked up.
*
* @param aConfigurationFiles The URIs of the configuration files describing
* the handled Spring application context.
*
* @param aPropertyFiles The URIs of the property files containing the
* values for the used placeholder.
*
* @throws MalformedURLException If a given URI is malformed
*/
public SpringServiceLookupImpl( Class> aType, URI[] aConfigurationFiles, URI[] aPropertyFiles ) throws MalformedURLException {
super( new SpringFactoryWrapper>( aType, new SpringBeanFactoryImpl( aConfigurationFiles, aPropertyFiles ) ) );
}
/**
* Creates a factory with the given Spring configuration files and the given
* properties. If the configuration files contains placeholder (e.g.
* ${jdbc.url}), the given properties are used to replace them.
*
* @param aType The actual type (class) of the {@link ServiceDescriptor}
* instances to be looked up.
*
* @param aConfigurationFiles The URIs of the configuration files describing
* the handled Spring application context.
*
* @param aProperties The dynamic properties which are not defined by an
* configuration file.
*
* @throws MalformedURLException If a given URI is malformed
*/
public SpringServiceLookupImpl( Class> aType, URI[] aConfigurationFiles, Properties aProperties ) throws MalformedURLException {
super( new SpringFactoryWrapper>( aType, new SpringBeanFactoryImpl( aConfigurationFiles, aProperties ) ) );
}
/**
* Creates a factory with the given Spring configuration files and the given
* properties. If the configuration files contains placeholder (e.g.
* ${jdbc.url}), the given property files and properties are used to replace
* them. The provided property files have to follow the JAVA properties
* scheme (key=value).
*
* @param aType The actual type (class) of the {@link ServiceDescriptor}
* instances to be looked up.
*
* @param aConfigurationFiles The URIs of the configuration files describing
* the handled Spring application context.
*
* @param aPropertyFiles The URIs of the property files containing the
* values for the used placeholder.
*
* @param aProperties The dynamic properties which are not defined by an
* configuration file.
*
* @throws MalformedURLException If a given URI is malformed
*/
public SpringServiceLookupImpl( Class> aType, URI[] aConfigurationFiles, URI[] aPropertyFiles, Properties aProperties ) throws MalformedURLException {
super( new SpringFactoryWrapper>( aType, new SpringBeanFactoryImpl( aConfigurationFiles, aPropertyFiles, aProperties ) ) );
}
// /////////////////////////////////////////////////////////////////////////
// INNER CLASSES:
// /////////////////////////////////////////////////////////////////////////
/**
* The {@link CollectionFactory} is a wrapper for the
* {@link TypeLookupFactory} using the {@link TypeLookupFactory} for
* creating the dedicated type.
*
* @param The type of the instances to be created by this factory.
*/
private static class SpringFactoryWrapper implements CollectionFactory> {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private TypeLookupFactory _lookupTypeFactory;
private Class _type;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs a {@link SpringFactoryWrapper} wrapping
* {@link TypeLookupFactory} for creating instances of the required
* type.
*
* @param aType The type of the instances to be created.
*
* @param aLookupTypeFactory The {@link TypeLookupFactory} to be used
* for creating the instances of the required type.
*/
public SpringFactoryWrapper( Class aType, TypeLookupFactory aLookupTypeFactory ) {
_lookupTypeFactory = aLookupTypeFactory;
_type = aType;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* To instances.
*
* @return the sets the
*/
@Override
public Set createInstances() {
return _lookupTypeFactory.createInstances( _type );
}
/**
* To instances.
*
* @param aProperties the a properties
* @return the sets the
*/
@Override
public Set createInstances( Properties aProperties ) {
return _lookupTypeFactory.toInstances( _type, aProperties );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy