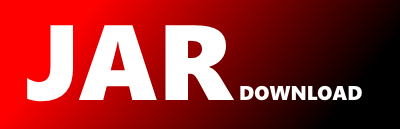
org.refcodes.web.BearerAuthCredentials Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-web Show documentation
Show all versions of refcodes-web Show documentation
This artifact provides web (HTTP) related definitions and types being used
by REFCODES.ORG web related functionality and artifacts.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.web;
/**
* The {@link BearerAuthCredentials} defines a type for representing a
* Bearer-Authentication credentials Header-Field. Parse the Header-Field via
* {@link #fromHttpAuthorization(String)} and feed it with the header's
* {@link HeaderField#AUTHORIZATION} field's value. To create the according
* Header-Field's value from the {@link BearerAuthCredentials} type, call
* {@link #toHttpAuthorization()}.
*/
public class BearerAuthCredentials implements TokenCredentials, AuthTypeCredentials {
// /////////////////////////////////////////////////////////////////////////
// STATICS:
// /////////////////////////////////////////////////////////////////////////
public static final char DELIMITER_AUTH_TYPE = ' ';
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _token;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new bearer auth credentials impl.
*/
public BearerAuthCredentials() {}
/**
* Instantiates a new bearer auth credentials impl.
*
* @param aToken the secret
*/
public BearerAuthCredentials( String aToken ) {
_token = aToken;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Validates the provided {@link TokenCredentials} with this
* {@link BearerAuthCredentials} via {@link #isValid(TokenCredentials)}. In
* case the provided {@link TokenCredentials} are not valid, then a
* {@link ForbiddenException} is thrown.
*
* @param aCredentials The {@link TokenCredentials} to be verified.
*
* @throws ForbiddenException thrown in case the provided
* {@link TokenCredentials} do not match.
*/
@Override
public void validate( TokenCredentials aCredentials ) throws ForbiddenException {
if ( !isValid( aCredentials ) ) {
throw new ForbiddenException( "Access denied as of unsuccessfull authentification!" );
}
}
/**
* Validates the provided user-name and secret with this
* {@link BearerAuthCredentials} via {@link #isValid(String)}. In case the
* provided credentials are not valid, then a {@link ForbiddenException} is
* thrown.
*
* @param aToken The secret part to be tested if it fits with the this
* {@link TokenCredentials} instance.
*
* @throws ForbiddenException thrown in case the provided
* {@link TokenCredentials} do not match.
*/
public void validate( String aToken ) throws ForbiddenException {
if ( !isValid( aToken ) ) {
throw new ForbiddenException( "Access denied as of unsuccessfull authentification!" );
}
}
/**
* {@inheritDoc}
*/
@Override
public BearerAuthCredentials withHttpAuthorization( String aHttpAuthorization ) {
fromHttpAuthorization( aHttpAuthorization );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public AuthType getAuthType() {
return AuthType.BEARER;
}
/**
* {@inheritDoc}
*/
@Override
public String getToken() {
return _token;
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ( ( _token == null ) ? 0 : _token.hashCode() );
return result;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals( Object obj ) {
if ( this == obj ) {
return true;
}
if ( obj == null ) {
return false;
}
if ( getClass() != obj.getClass() ) {
return false;
}
final BearerAuthCredentials other = (BearerAuthCredentials) obj;
if ( _token == null ) {
if ( other._token != null ) {
return false;
}
}
else if ( !_token.equals( other._token ) ) {
return false;
}
return true;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return "<...>";
}
/**
* {@inheritDoc}
*/
@Override
public void fromHttpAuthorization( String aHttpAuthorization ) {
if ( aHttpAuthorization == null ) {
throw new IllegalArgumentException( "The provided HTTP authorization value does not conform to the format expected for bearer authentication." );
}
final int theMarker = aHttpAuthorization.indexOf( DELIMITER_AUTH_TYPE );
if ( theMarker == -1 || !aHttpAuthorization.substring( 0, theMarker ).equalsIgnoreCase( AuthType.BEARER.getName() ) ) {
throw new IllegalArgumentException( "The provided HTTP authorization value does not conform to the format expected for <" + AuthType.BEARER.getName() + "> authentication." );
}
_token = aHttpAuthorization.substring( theMarker + 1 );
}
/**
* {@inheritDoc}
*/
@Override
public String toHttpAuthorization() {
return getAuthType().getName() + DELIMITER_AUTH_TYPE + getToken();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy