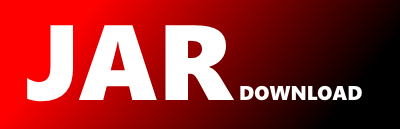
org.refcodes.web.FormFields Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-web Show documentation
Show all versions of refcodes-web Show documentation
This artifact provides web (HTTP) related definitions and types being used
by REFCODES.ORG web related functionality and artifacts.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.web;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.List;
import java.util.Map;
import org.refcodes.data.Delimiter;
import org.refcodes.data.Encoding;
/**
* The {@link FormFields} represent the URL's Query-String by the notation
* originally used by the GET request of the ancient web forms (see
* "https://en.wikipedia.org/wiki/Query_string#Web_forms"). Therefore the name
* {@link FormFields}, as them web forms provided fields with values (see
* "https://en.wikipedia.org/wiki/Form_(HTML)")
*
* @see "https://en.wikipedia.org/wiki/Query_string"
*/
public class FormFields extends AbstractHttpFields {
private static final long serialVersionUID = 1L;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new form fields impl.
*/
public FormFields() {}
/**
* Initializes the instance with the fields provided by the given
* {@link Map}.
*
* @param aHttpFields The {@link Map} from which to get the keys and values
* for initializing this instance.
*/
public FormFields( Map> aHttpFields ) {
super( aHttpFields );
}
/**
* Initializes the instance with the fields provided by the given HTTP
* Form-Fields (HTTP Query-String).
*
* @param aHttpFormFields The {@link String} from which to parse the keys
* and values for initializing this instance.
*/
public FormFields( String aHttpFormFields ) {
fromUrlQueryString( aHttpFormFields );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* Processes the HTTP Query-String and retrieves the therein defined
* Form-Fields and adds them to the {@link FormFields} instance.
*
* @param aQueryString The HTTP Query-String from which to extract the form
* being added to the {@link FormFields} instance.
*/
public void fromUrlQueryString( String aQueryString ) {
if ( aQueryString != null && aQueryString.length() != 0 ) {
if ( aQueryString.startsWith( "" + Delimiter.URL_QUERY.getChar() ) ) {
aQueryString = aQueryString.substring( 1 );
}
fromBodyFormFields( aQueryString );
}
}
/**
* Processes the HTTP Form-Fields body {@link String} and retrieves the
* therein defined Form-Fields and adds them to the {@link FormFields}
* instance.
*
* @param aHttpFormFields The HTTP Form-Fields {@link String} from which to
* extract the form being added to the {@link FormFields} instance.
*/
public void fromBodyFormFields( String aHttpFormFields ) {
if ( aHttpFormFields != null ) {
final String[] theFields = aHttpFormFields.split( "\\" + Delimiter.WEB_FIELD.getChar() );
String[] eFieldPair;
for ( String eField : theFields ) {
eFieldPair = eField.split( "" + Delimiter.PROPERTY.getChar() );
if ( eFieldPair.length == 1 ) {
addTo( eFieldPair[0], "" );
}
else {
try {
addTo( eFieldPair[0], URLDecoder.decode( eFieldPair[1], Encoding.UTF_8.getCode() ) );
}
catch ( UnsupportedEncodingException e ) {
addTo( eFieldPair[0], eFieldPair[1] );
}
}
}
}
}
/**
* Produces an HTTP Query-String form the herein contain HTTP query fields.
*
* @return The HTTP Query-String representation of this {@link FormFields}
* instance or null if this instance is empty.
*/
public String toUrlQueryString() {
if ( isEmpty() ) {
return null;
}
return Delimiter.URL_QUERY.getChar() + toBodyFormFields();
}
/**
* Produces an HTTP Form-Fields body {@link String} form the herein contain
* HTTP query fields.
*
* @return The HTTP Form-Fields {@link String} representation of this
* {@link FormFields} instance or null if this instance is empty.
*/
public String toBodyFormFields() {
if ( isEmpty() ) {
return null;
}
String theQueryString = "";
List eValues;
for ( String eKey : keySet() ) {
eValues = get( eKey );
for ( String eValue : eValues ) {
try {
eValue = URLEncoder.encode( eValue, Encoding.UTF_8.getCode() );
}
catch ( UnsupportedEncodingException ignore ) { /* unencoded */ }
if ( theQueryString.length() != 0 && theQueryString.charAt( theQueryString.length() - 1 ) != Delimiter.URL_QUERY.getChar() && theQueryString.charAt( theQueryString.length() - 1 ) != Delimiter.WEB_FIELD.getChar() ) {
theQueryString += "" + Delimiter.WEB_FIELD.getChar();
}
theQueryString += eKey + Delimiter.PROPERTY.getChar() + eValue;
}
}
return theQueryString;
}
/**
* Calculates the length (number of characters) of the {@link FormFields}
* when used for a HTTP-Body as of {@link #toBodyFormFields()}.
*
* @return The length of the HTTP-Body representation of the content of
* these {@link FormFields}.
*/
public int toContentLength() {
return toBodyFormFields().length();
}
/**
* Extracts the HTTP Query-String from the provided URL by identifying the
* first question mark ("?") and retrieves the therein defined Form-Fields
* and adds them to the {@link FormFields} instance.
*
* @param aUrl The URL from which to extract the HTTP Query-String which's
* Form-Fields are to be added to the {@link FormFields} instance.
*/
public void fromUrl( String aUrl ) {
if ( aUrl != null ) {
final int index = aUrl.indexOf( Delimiter.URL_QUERY.getChar() );
if ( index != -1 ) {
fromUrlQueryString( aUrl.substring( index + 1 ) );
}
}
}
/**
* Creates a new {@link FormFields} instance.
*
* @return The newly created {@link FormFields} instance.
*/
// public static FormFields toFormFields() {
// return new FormFieldsImpl();
// }
/**
* Creates a new {@link FormFields} instance.
*
* @param aFormFields The Form-Fields' {@link String} representation from
* which to construct the {@link FormFields} instance.
*
* @return The newly created {@link FormFields} instance.
*/
// public static FormFields toFormFields( String aFormFields ) {
// return aFormFields != null ? new FormFieldsImpl( aFormFields ) : null;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy