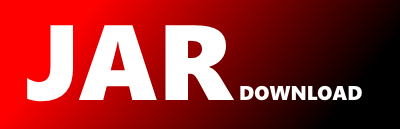
org.refcodes.web.HeaderField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-web Show documentation
Show all versions of refcodes-web Show documentation
This artifact provides web (HTTP) related definitions and types being used
by REFCODES.ORG web related functionality and artifacts.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.web;
import org.refcodes.mixin.NameAccessor;
/**
* The Enum HeaderField.
*/
public enum HeaderField implements NameAccessor {
AUTHORIZATION("Authorization"),
ALLOW("Allow"),
CONTENT_ENCODING("Content-Encoding"),
CONTENT_LANGUAGE("Content-Language"),
CONTENT_LENGTH("Content-Length"),
CONTENT_LOCATION("Content-Location"),
CONTENT_MD5("Content-MD5"),
CONTENT_RANGE("Content-Range"),
CONTENT_TYPE("Content-Type"),
EXPIRES("Expires"),
LAST_MODIFIED("Last-Modified"),
EXTENSION_HEADER("Extension-Header"),
/**
* The Cookie HTTP Request-Header contains stored HTTP cookies previously
* sent by the server with the {@link #SET_COOKIE} header.
*/
COOKIE("Cookie"),
/**
* The Set-Cookie HTTP Response-Header is used to send cookies from the
* server to the user agent.
*/
SET_COOKIE("Set-Cookie"),
ACCEPT("Accept"),
ACCEPT_ENCODING("Accept-Encoding"),
/**
* "... The Accept-Language request HTTP header advertises which languages
* the client is able to understand, and which locale variant is preferred
* ..."
*
* @see "https://developer.mozilla.org/en-US/docs/Web/HTTP/Headers/Accept-Language"
*/
ACCEPT_LANGUAGE("Accept-Language"),
ACCEPT_CHARSET("Accept-Charset"),
CONNECTION("Connection"),
HOST("Host"),
/**
* "... The Location response-header field is used to redirect the recipient
* to a location other than the Request-URI for completion of the request or
* identification of a new resource. For 201 (Created) responses, the
* Location is that of the new resource which was created by the request.
* For 3xx responses, the location SHOULD indicate the server's preferred
* URI for automatic redirection to the resource. The field value consists
* of a single absolute URI ..."
*
* @see "https://tools.ietf.org/html/rfc2616#page-135"
*/
LOCATION("Location"),
USER_AGENT("User-Agent"),
REQUEST_ID("Request-TID"),
SESSION_ID("Session-TID"),
WWW_AUTHENTICATE("WWW-Authenticate"),
/**
* B3 ids are fixed-length lowerhex encoded values.
*/
X_B3_TRACE_ID("X-B3-TraceId"),
/**
* B3 ids are fixed-length lowerhex encoded values.
*/
X_B3_TRACE_SPAN_ID("X-B3-SpanId"),
/**
* B3 ids are fixed-length lowerhex encoded values.
*/
X_B3_PARENT_SPAN_ID("X-B3-ParentSpanId"),
/**
* "... The X-RequestId Header-Field MUST be a combination of a globally
* unique value in the format of a GUID followed by an increasing decimal
* counter which MUST increase with every new HTTP-Request (for example,
* "{E2EA6C1C-E61B-49E9-9CFB-38184F907552}:123456"). The GUID portion of the
* X-RequestId header MUST be unique across all Session Contexts and MUST
* NOT change for the life of the Session Context. The client MUST send this
* header on every request and the server MUST return this header with the
* same information in the response back to the client ..."
*
* @see "https://msdn.microsoft.com/en-us/library/dn530858(v=exchg.80).aspx"
*/
X_REQUEST_ID("X-RequestId"),
/**
* For OAuth: OAuth scopes let you specify exactly how your application
* needs to access a user's resource. Scope is specified on either the
* authorization or token endpoints using the parameter scope. Scope is
* expressed as a set of case-sensitive and space-delimited strings. The
* authorization server may override the scope request, in this case it must
* include scope in its response to inform a client of their actual scope.
* When a scope is not specified, the server may either fallback to a
* well-documented default, or fail the request. "... OPTIONAL, if identical
* to the scope requested by the client; otherwise, REQUIRED. The scope of
* the access token as described by Section 3.3. ...", "... OPTIONAL. The
* scope of the access request as described by Section 3.3 ..."
* (https://tools.ietf.org/html/rfc6749)
*/
SCOPE("Scope"),
/**
* For OAuth: "... REQUIRED. The access token issued by the authorization
* server ..." (https://tools.ietf.org/html/rfc6749)
*/
ACCESS_TOKEN("access_token"),
/**
* For OAuth: "... OPTIONAL. The refresh token, which can be used to obtain
* new access tokens using the same authorization grant as described in
* Section 6. ..." (https://tools.ietf.org/html/rfc6749)
*/
REFRESH_TOKEN("refresh_token"),
/**
* Token type, see also {@link TokenType}. For OAuth: "... REQUIRED. The
* type of the token issued as described in Section 7.1. Value is case
* insensitive ..." (https://tools.ietf.org/html/rfc6749)
*/
TOKEN_TYPE("token_type"),
/**
* For OAuth: "... RECOMMENDED. The lifetime in seconds of the access token.
* For example, the value "3600" denotes that the access token will expire
* in one hour from the time the response was generated. If omitted, the
* authorization server SHOULD provide the expiration time via other means
* or document the default value ..." (https://tools.ietf.org/html/rfc6749)
*/
EXPIRES_IN("expires_in"),
/**
* For OAuth: "... REQUIRED. The client identifier issued to the client
* during the registration process described by Section 2.2. ..."
* (https://tools.ietf.org/html/rfc6749)
*/
CLIENT_ID("client_id"),
/**
* For OAuth: "... REQUIRED. The client secret. The client MAY omit the
* parameter if the client secret is an empty string ..."
* (https://tools.ietf.org/html/rfc6749)
*/
CLIENT_SECRET("client_secret"),
/**
* For OAuth, it must be set to {@link GrantType#AUTHORIZATION_CODE}: "...
* grant_type REQUIRED. Value MUST be set to "authorization_code" ..."
* (https://tools.ietf.org/html/rfc6749)
*/
GRANT_TYPE("grant_type"),
/**
* For OAuth: "... REQUIRED, if the "redirect_uri" parameter was included in
* the authorization request as described in Section 4.1.1, and their values
* MUST be identical ..." (https://tools.ietf.org/html/rfc6749)
*/
REDIRECT_URI("redirect_uri"),
/**
* For OAuth: "... REQUIRED. The resource owner username ..."
* (https://tools.ietf.org/html/rfc6749)
*/
USERNAME("username"),
/**
* For OAuth: "... REQUIRED. The resource owner password ..."
* (https://tools.ietf.org/html/rfc6749)
*/
PASSWORD("password"),
/**
* Probably legacy, encountered upon using KeyCloak with OAuth.
*/
SESSION_STATE("session_state"),
/**
* Probably legacy, encountered upon using KeyCloak with OAuth.
*/
REFRESH_EXPIRES_IN("refresh_expires_in"),
/**
* Probably legacy, encountered upon using KeyCloak with OAuth.
*/
NOT_BEFORE_POLICY("not-before-policy");
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private String _name;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new Header-Field.
*
* @param aName the name
*/
private HeaderField( String aName ) {
_name = aName;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public String getName() {
return _name;
}
// /////////////////////////////////////////////////////////////////////////
// HELPER:
// /////////////////////////////////////////////////////////////////////////
/**
* Retrieves the {@link HeaderField} which corresponds to the given HTTP
* Header-Field name. The provided HTTP Header-Field name is tested case
* insensitive.
*
* @param aHttpHeaderField The HTTP Header-Field name for which to get a
* unified {@link HeaderField}.
*
* @return Returns the according {@link HeaderField} or null if none such
* {@link HeaderField} has yet been defined.
*/
public static HeaderField toHeaderField( String aHttpHeaderField ) {
for ( HeaderField eField : values() ) {
if ( eField.getName().equalsIgnoreCase( aHttpHeaderField ) ) {
return eField;
}
}
return null;
}
/**
* Normalizes the input HTTP Header-Field to match the case of the
* Header-Fields as defined in this {@link HeaderField} enumeration. If
* there is none such corresponding Header-Field, then the provided
* Header-Field is returned as is.
*
* @param aHttpHeaderField The incoming Header-Field to be normalized.
*
* @return The normalized HeaderF-Field or, if not possible, the unmodified
* Header-Field-
*/
public static String toHttpHeaderField( String aHttpHeaderField ) {
for ( HeaderField eField : values() ) {
if ( eField.getName().equalsIgnoreCase( aHttpHeaderField ) ) {
return eField.getName();
}
}
return aHttpHeaderField;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy